How to Hide, Recover and Delete Tkinter Widgets
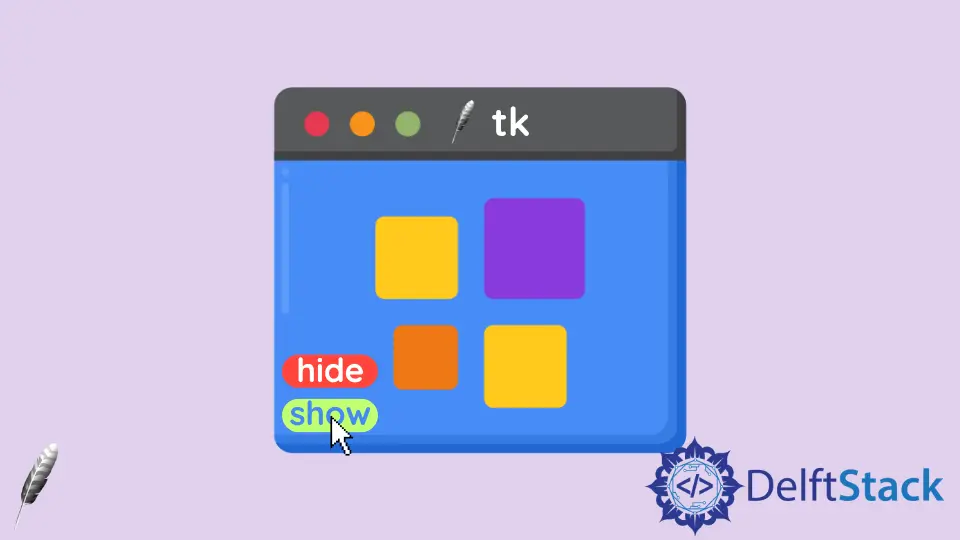
In this tutorial, we will demonstrate how to hide, recover the Tkinter widgets, by clicking a Tkinter button. Eventually, we will also show you how to delete or kill existing Tkinter widgets.
Hide and Recover Tkinter Widgets
pack_forget()
Method to Hide Tkinter Widgets
In contrast to pack
method in Tkinter widgets, we could hide the Tkinter widgets by calling pack_forget()
method to make the widgets invisible.
try:
import Tkinter as tk
except:
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.label = tk.Label(self.root, text="Label")
self.buttonForget = tk.Button(
self.root,
text="Click to hide Label",
command=lambda: self.label.pack_forget(),
)
self.buttonRecover = tk.Button(
self.root, text="Click to show Label", command=lambda: self.label.pack()
)
self.buttonForget.pack()
self.buttonRecover.pack()
self.label.pack(side="bottom")
self.root.mainloop()
def quit(self):
self.root.destroy()
app = Test()
self.buttonForget = tk.Button(
self.root, text="Click to hide Label", command=lambda: self.label.pack_forget()
)
pack_forget()
is bind to the command of the button buttonForget
.
It will hide the label widget after clicking the button, but the label itself still exists but becomes invisible.
We need to call the pack()
method again to pack the widget to make it visible, or in other words, to recover it.
grid_forget()
Method to Hide Tkinter Widgets if grid
Layout Is Used
If the widgets are placed with the grid
layout manager, we should use grid_forget()
method to make the Tkinter widgets invisible.
try:
import Tkinter as tk
except:
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.label = tk.Label(self.root, text="Label")
self.buttonForget = tk.Button(
self.root,
text="Click to hide Label",
command=lambda: self.label.grid_forget(),
)
self.buttonRecover = tk.Button(
self.root, text="Click to show Label", command=lambda: self.label.grid()
)
self.buttonForget.grid(column=0, row=0, padx=10, pady=10)
self.buttonRecover.grid(column=0, row=1, padx=10, pady=10)
self.label.grid(column=0, row=2, padx=10, pady=10)
self.root.mainloop()
def quit(self):
self.root.destroy()
app = Test()
self.buttonForget = tk.Button(
self.root, text="Click to hide Label", command=lambda: self.label.grid_forget()
)
Here, we bind the grid_forget
method to the command of the button.
You could notice that the position of the label is not the same before it is hidden after you call grid
method again.
If we intend to restore the label to its original position, grid_remove
method shall be the right option.
grid_remove()
Method to Hide Tkinter Widgets if grid
Layout Is Used
grid_remove()
hides the widget but remembers the grid options like column
and row
.
try:
import Tkinter as tk
except:
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.label = tk.Label(self.root, text="Label")
self.buttonForget = tk.Button(
self.root,
text="Click to hide Label",
command=lambda: self.label.grid_remove(),
)
self.buttonRecover = tk.Button(
self.root, text="Click to show Label", command=lambda: self.label.grid()
)
self.buttonForget.grid(column=0, row=0, padx=10, pady=10)
self.buttonRecover.grid(column=0, row=1, padx=10, pady=10)
self.label.grid(column=0, row=2, padx=10, pady=20)
self.root.mainloop()
def quit(self):
self.root.destroy()
app = Test()
Delete Tkinter Widgets Permanently by Clicking a Button
We will show how to delete Tkinter widgets permanently by calling the destroy
method in this section.
try:
import Tkinter as tk
except:
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.label = tk.Label(self.root, text="Label")
self.buttonForget = tk.Button(
self.root, text="Click to hide Label", command=lambda: self.label.destroy()
)
self.buttonRecover = tk.Button(
self.root, text="Click to show Label", command=lambda: self.label.pack()
)
self.buttonForget.pack()
self.buttonRecover.pack()
self.label.pack(side="bottom")
self.root.mainloop()
def quit(self):
self.root.destroy()
app = Test()
The button is deleted after clicking the button.
You could try to click the button buttonRecover
but will get the _tkinter.TclError
error notification as below,
Exception in Tkinter callback
Traceback (most recent call last):
File "D:\WinPython\WPy-3661\python-3.6.6.amd64\lib\tkinter\__init__.py", line 1702, in __call__
return self.func(*args)
File "C:\Users\jinku\OneDrive\Bureaublad\test.py", line 17, in <lambda>
command=lambda: self.label.pack())
File "D:\WinPython\WPy-3661\python-3.6.6.amd64\lib\tkinter\__init__.py", line 2140, in pack_configure
+ self._options(cnf, kw))
_tkinter.TclError: bad window path name ".!label"
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook