How to Change the Tkinter Label Font Size
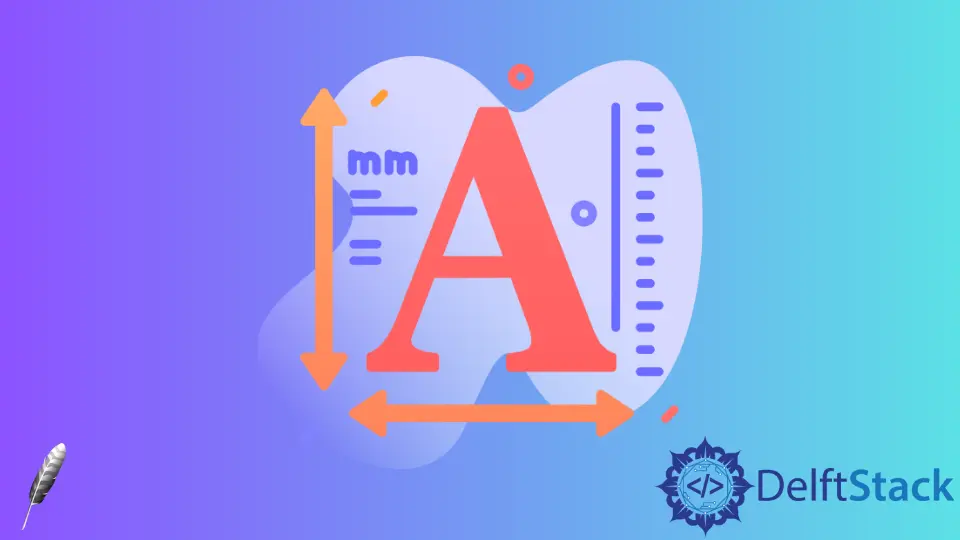
This tutorial guide demonstrates how to change the Tkinter label font size. We create two buttons Increase
and Decrease
to increase/decrease the Tkinter label font size.
Change the Tkinter Label Font Size
import tkinter as tk
import tkinter.font as tkFont
app = tk.Tk()
fontStyle = tkFont.Font(family="Lucida Grande", size=20)
labelExample = tk.Label(app, text="20", font=fontStyle)
def increase_label_font():
fontsize = fontStyle["size"]
labelExample["text"] = fontsize + 2
fontStyle.configure(size=fontsize + 2)
def decrease_label_font():
fontsize = fontStyle["size"]
labelExample["text"] = fontsize - 2
fontStyle.configure(size=fontsize - 2)
buttonExample1 = tk.Button(app, text="Increase", width=30, command=increase_label_font)
buttonExample2 = tk.Button(app, text="Decrease", width=30, command=decrease_label_font)
buttonExample1.pack(side=tk.LEFT)
buttonExample2.pack(side=tk.LEFT)
labelExample.pack(side=tk.RIGHT)
app.mainloop()
fontStyle = tkFont.Font(family="Lucida Grande", size=20)
We specify the font to be font family Lucida Grande
with size of 20
, and assign it to be the font of label labelExample
.
def increase_label_font():
fontsize = fontStyle["size"]
labelExample["text"] = fontsize + 2
fontStyle.configure(size=fontsize + 2)
The font size is updated with tkinter.font.configure()
method. The widget that uses this specific font will be updated automatically as you could see from the gif animation.
labelExample["text"] = fontsize + 2
We also update the label text to be same with font size to make the animation more intuitive.
Change the Tkinter Label Font Family
We will also introduce how to change the Tkinter label font family by clicking the button.
import tkinter as tk
import tkinter.font as tkFont
app = tk.Tk()
fontfamilylist = list(tkFont.families())
fontindex = 0
fontStyle = tkFont.Font(family=fontfamilylist[fontindex])
labelExample = tk.Label(app, text=fontfamilylist[fontindex], font=fontStyle)
def increase_label_font():
global fontindex
fontindex = fontindex + 1
labelExample.configure(
font=fontfamilylist[fontindex], text=fontfamilylist[fontindex]
)
buttonExample1 = tk.Button(
app, text="Change Font", width=30, command=increase_label_font
)
buttonExample1.pack(side=tk.LEFT)
labelExample.pack(side=tk.RIGHT)
app.mainloop()
fontfamilylist = list(tkFont.families())
It gets the available Tkinter font families list.
labelExample.configure(font=fontfamilylist[fontindex], text=fontfamilylist[fontindex])
The font property of labelExample
will change to the next font in the font.families
list, and the label text is also updated to the font name.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - Tkinter Label
- How to Hide, Recover and Delete Tkinter Widgets
- How to Change the Tkinter Label Text
- How to Get the Tkinter Label Text
- How to Set Border of Tkinter Label Widget