How to Change the Tkinter Label Text
-
Use
StringVar
to Change/Update the Tkinter Label Text -
Label
text
Property to Change/Update the Python Tkinter Label Text
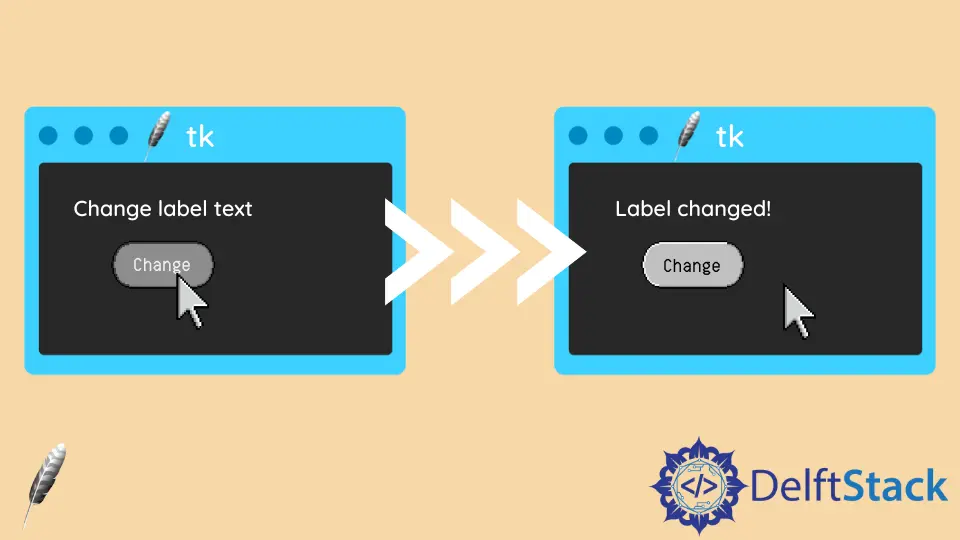
In this tutorial, we will introduce how to change the Tkinter label text when clicking a button.
Use StringVar
to Change/Update the Tkinter Label Text
StringVar
is one type of Tkinter constructor to create the Tkinter string variable.
After we associate the StringVar
variable to the Tkinter widgets, Tkinter will update this particular widget when the variable is modified.
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.text = tk.StringVar()
self.text.set("Test")
self.label = tk.Label(self.root, textvariable=self.text)
self.button = tk.Button(
self.root, text="Click to change text below", command=self.changeText
)
self.button.pack()
self.label.pack()
self.root.mainloop()
def changeText(self):
self.text.set("Text updated")
app = Test()
self.text = tk.StringVar()
self.text.set("Test")
The Tkinter constructor couldn’t initiate the string variable with the string like self.text = tk.StringVar()
.
We should call set
method to set the StringVar
value, like self.text.set("Test")
.
self.label = tk.Label(self.root, textvariable=self.text)
It associates the StringVar
variable self.text
to the label widget self.label
by setting textvariable
to be self.text
. The Tk toolkit begins to track the changes of self.text
and will update the text self.label
if self.text
is modified.
The above code creates a Tkinter dynamic label. It automatically displays the Tkinter label text upon modification of self.text
.
Label text
Property to Change/Update the Python Tkinter Label Text
Another solution to change the Tkinter label text is to change the text
property of the label.
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.label = tk.Label(self.root, text="Text")
self.button = tk.Button(
self.root, text="Click to change text below", command=self.changeText
)
self.button.pack()
self.label.pack()
self.root.mainloop()
def changeText(self):
self.label["text"] = "Text updated"
app = Test()
The text of the label could be initiated with text="Text"
and could also be updated by assigning the new value to the text
key of the label object.
We could also change the text
property with the tk.Label.configure()
method as shown below. It works the same with the above codes.
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.label = tk.Label(self.root, text="Text")
self.button = tk.Button(
self.root, text="Click to change text below", command=self.changeText
)
self.button.pack()
self.label.pack()
self.root.mainloop()
def changeText(self):
self.label.configure(text="Text Updated")
app = Test()
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook