Cómo cambiar el texto de la etiqueta Tkinter
-
Utilice
StringVar
para cambiar el texto de la etiqueta de Tkinter -
Propiedad Label
text
para cambiar el texto de la etiqueta
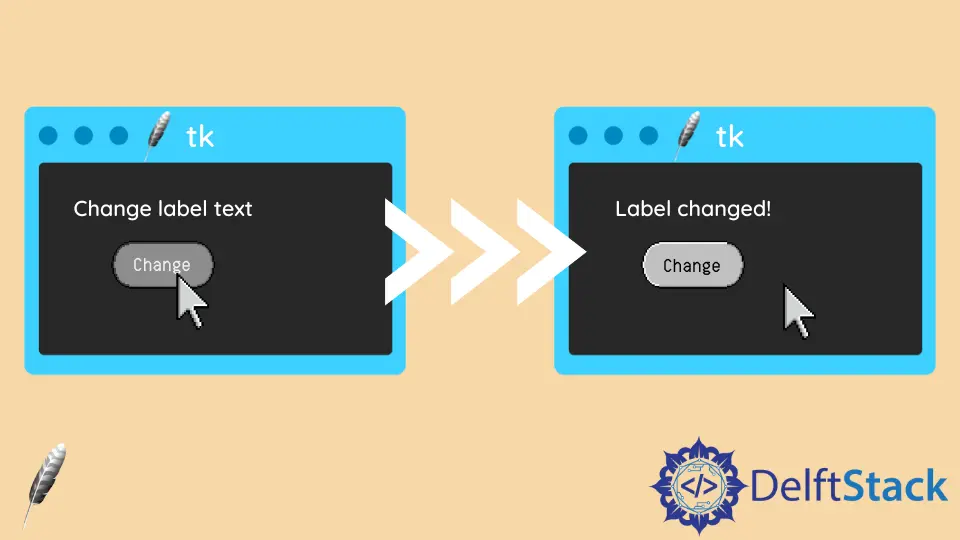
En este tutorial, introduciremos cómo cambiar el texto de la etiqueta de Tkinter haciendo clic en un botón.
Utilice StringVar
para cambiar el texto de la etiqueta de Tkinter
StringVar
es un tipo de constructor de Tkinter para crear la variable de cadena de Tkinter.
Después de asociar la variable StringVar
a los widgets de Tkinter, Tkinter actualizará este widget particular cuando la variable sea modificada.
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.text = tk.StringVar()
self.text.set("Test")
self.label = tk.Label(self.root, textvariable=self.text)
self.button = tk.Button(
self.root, text="Click to change text below", command=self.changeText
)
self.button.pack()
self.label.pack()
self.root.mainloop()
def changeText(self):
self.text.set("Text updated")
app = Test()
self.text = tk.StringVar()
self.text.set("Test")
El constructor de Tkinter no podría iniciar la variable string con la cadena como self.text = tk.StringVar()
.
Deberíamos llamar al método set
para establecer el valor StringVar
, como self.text.set("Test")
.
self.label = tk.Label(self.root, textvariable=self.text)
Asocia la variable StringVar
self.text
al widget de la etiqueta self.label
estableciendo textvariable
como self.text
. El conjunto de herramientas Tk comienza a rastrear los cambios de self.text
y actualizará el texto self.label
si self.text
es modificado.
Propiedad Label text
para cambiar el texto de la etiqueta
Otra solución para cambiar el texto de la etiqueta de Tkinter es cambiar la propiedad text
de la etiqueta.
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.label = tk.Label(self.root, text="Text")
self.button = tk.Button(
self.root, text="Click to change text below", command=self.changeText
)
self.button.pack()
self.label.pack()
self.root.mainloop()
def changeText(self):
self.label["text"] = "Text updated"
app = Test()
El texto de la etiqueta podría iniciarse con text="Text"
y también podría actualizarse asignando el nuevo valor a la clave text
del objeto etiqueta.
También podríamos cambiar la propiedad text
por el método tk.Label.configure()
como se muestra a continuación. Funciona igual con los códigos anteriores.
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.label = tk.Label(self.root, text="Text")
self.button = tk.Button(
self.root, text="Click to change text below", command=self.changeText
)
self.button.pack()
self.label.pack()
self.root.mainloop()
def changeText(self):
self.label.configure(text="Text Updated")
app = Test()
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook