Tkinter Tutorial - Button
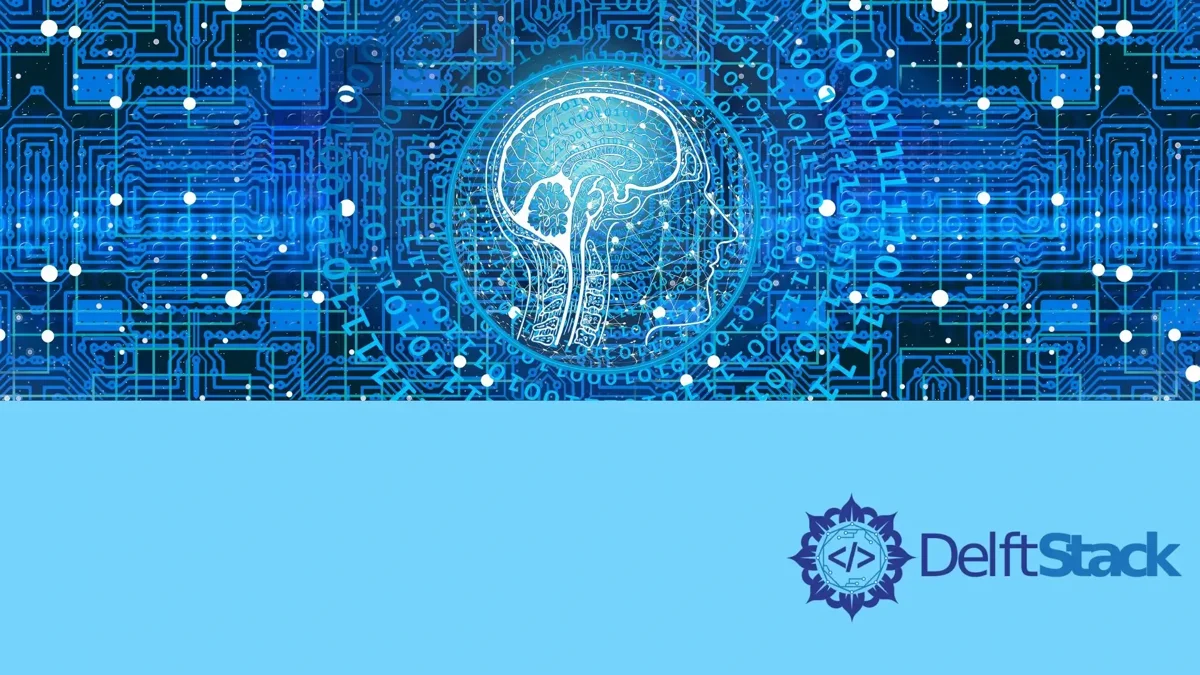
Tkinter button widget is quite similar to the Tkinter Label widget. It has almost the same options as those in the label, except it has one extra default
option. We will give details of this default
after one basic button example.
You could generate two basic buttons using the demo codes below.
from sys import version_info
if version_info.major == 2:
import Tkinter as tk
elif version_info.major == 3:
import tkinter as tk
app = tk.Tk()
button1 = tk.Button(app, text="Python Label 1")
button2 = tk.Button(app, text="Python Label 2")
button1.pack(side=tk.LEFT)
button2.pack(side=tk.LEFT)
app.mainloop()
You could see the window with two buttons adjacent to each other.
Tkinter Button default
Option
The default
option in the button tells the button is the default button in the GUI, for example, the one invoked automatically when the user presses the Enter
or Return
key.
Tkinter Button Command Callback
The command
option invokes the callback function when the button is pressed.
from sys import version_info
if version_info.major == 2:
import Tkinter as tk
elif version_info.major == 3:
import tkinter as tk
app = tk.Tk()
labelExample = tk.Button(app, text="0")
def change_label_number():
counter = int(str(labelExample["text"]))
counter += 1
labelExample.config(text=str(counter))
buttonExample = tk.Button(app, text="Increase", width=30, command=change_label_number)
buttonExample.pack()
labelExample.pack()
app.mainloop()
It creates a button and a label widget in the main window. The number in the label text is increased by one every time the button is clicked.
def change_label_number():
counter = int(str(labelExample["text"]))
counter += 1
labelExample.config(text=str(counter))
The change_label_number
is the function invoked when the button is clicked. It gets the number of the label and then sets the label increased by one.
buttonExample = tk.Button(app, text="Increase", width=30, command=change_label_number)
It builds the binding between the button and its callback function.
Tkinter Button Command Callback Function With Arguments
You need a partial
object from functools
if you need to pass arguments to the callback functions. partial
objects are callable objects with the positional arguments args
and keyword arguments keywords
.
The below code snippet demonstrates how to use this partial
function in the button command.
def change_label_number(num):
counter = int(str(labelExample["text"]))
counter += num
labelExample.config(text=str(counter))
buttonExample = tk.Button(
app, text="Increase", width=30, command=partial(change_label_number, 2)
)
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook