How to Get Height of the Div Element in JavaScript
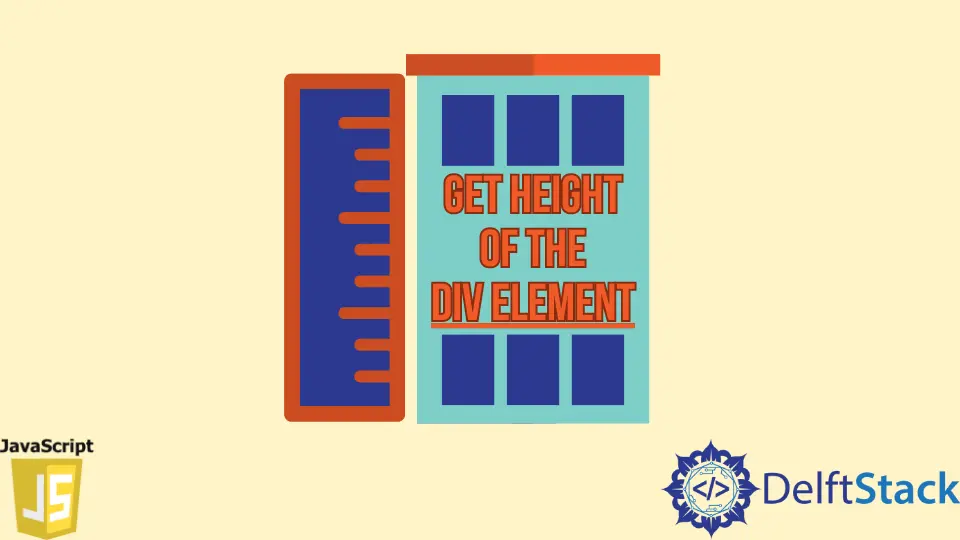
In HTML, whatever CSS properties which you provide to an element either by using CSS stylesheets or by using JavaScript are set inside the Document Object Model (DOM). Through this DOM, you can easily access these values later inside the JavaScript code. There are various ways to get the values of the CSS properties inside JavaScript.
This article will introduce how to get the CSS property height
from our JavaScript code.
Below is the HTML document which we have created. Inside, we have a body
tag containing only a single div
element having an id
of container
. In the head
tag, we have provided some basic styles to our div element like width, height, border, padding, etc.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#container{
width: 100px;
height: 50px;
border: 1px solid red;
padding: 4px;
background-color: cadetblue;
}
</style>
</head>
<body>
<div id="container"></div>
<script></script>
</body>
</html>
Output:
If you run the above HTML document, you will see a rectangle like this on your browser window.
Now that we have everything set up, let’s apply various properties on this container
div element to get its height. The code we will be writing will go inside the <script></script>
tags present inside the body
tag.
Various Ways to Get the div
Elements Height in JavaScript
First, we will get the reference of the container
element from the HTML DOM using the getElementById()
method. Then we will store that element inside the element
variable in JavaScript.
let element = document.getElementById('container');
Now that we have the div element, let’s get the height of the div
using various properties using JavaScript.
1. clientHeight
The clientHeight
returns the height of an element including the padding as an integer value.
console.log(element.clientHeight);
Output:
58
Since we have added padding of 4px
to all four sides of the container while calculating the height, which is currently 50px
, the padding of 4px
top and 4px
bottom, i.e., total padding of 8px
will be added to the height. This will result in a total height of 58px
.
2. offsetHeight
The offsetHeight
consists of the element’s height which also includes any padding, borders, and horizontal scrollbar (if present).
console.log(element.offsetHeight);
Output:
60
Similar to the clientHeight
property, the offsetHeight
property will also take 8px
padding, content’s height which is 50px
and also the border-width which is 1px
top and 1px
bottom that is total of 2px. Therefore the overall height returned by this property will be 8 + 50 + 2
i.e 60px
.
3. scrollHeight
The scrollHeight
returns the content’s height, including the content that is not visible on the screen due to overflow. It only includes padding of the element but not the border, margin, or horizontal scrollbar.
The scrollHeight
is similar to the clientHeight
. The only difference between clientHeight
and scrollHeight
is that the scrollheight
can also include the content’s height, which is currently not visible on the screen due to overflow.
console.log(element.scrollHeight);
Output:
58
The content’s height is 50px
and total padding is 8px
. Therefore, the total height returned by the scrollHeight
property is 58px
.
4. getBoundingClientRect()
The getBoundingClientRect()
method returns an object which contains information related to the element’s width and height as well as its position (x, y, top, left, etc) relative to the viewport. The height calculated by this method also contains padding and borders.
console.log(element.getBoundingClientRect().height);
Output:
60
So, to get the height of the element from the object returned by the getBoundingClientRect()
method, we have to use the height
key to get the height. Here, element height is 50px
, padding is 8px
and border-width is 2px
. So, a total 60px
height will be returned by this function.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn