How to Convert a Number to Binary Format in JavaScript
- Create a Function to Convert the Number to Binary in JavaScript
-
Convert a Number to Binary With
toString(2)
Function
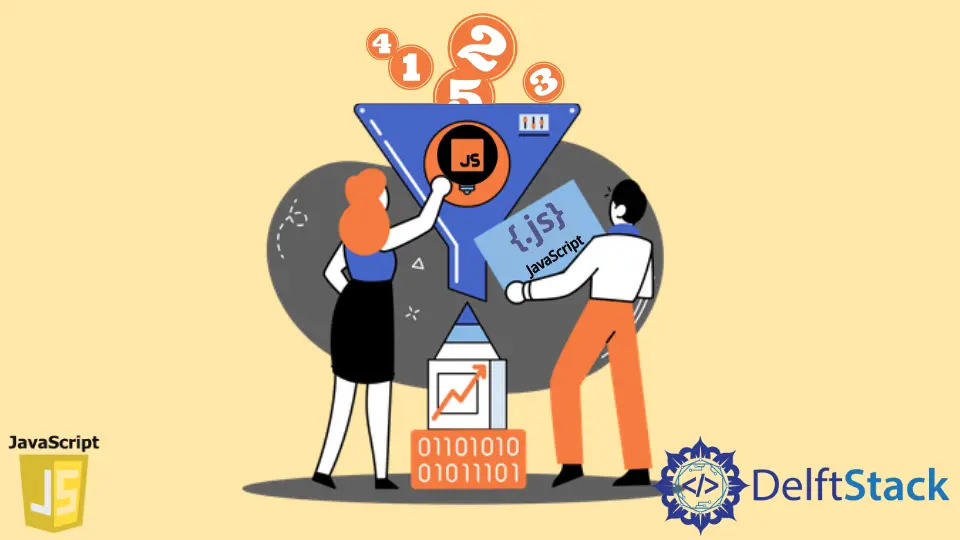
Converting a number to binary is quite a complex process. If we are to sit and convert the numbers manually, the result will be error-prone. How do we change a number to a binary format? JavaScript does not have many built-in functions to do so. We will introduce how to convert a number to binary in JavaScript.
Create a Function to Convert the Number to Binary in JavaScript
Before we go to the code, we need to know the conversion process from a decimal number (base 10) to a binary number (base 2). For simplicity, we will cover the conversion of positive whole numbers in this article. Hence, the change of negative integers and floating-point numbers is out of the scope of this article.
Understand the Conversion Process
Given an integer (or JavaScript number), we keep dividing the number by two and capturing its remainder till the number turns less than 2. For example, if we have a number 25, keep dividing 25
by 2
until we get the quotient less than 2.
Divisor | Quotient | Remainder | Bits |
---|---|---|---|
25 / 2 | 12 | 1 | 0 |
12 / 2 | 6 | 0 | 1 |
6 / 2 | 3 | 0 | 2 |
3 / 2 | 1 | 1 | 3 |
1 / 2 | 0 | 1 | 4 |
We read the digits from highest to lowest. Hence the binary value for number 25 is 1101.
We use the following set of calculations to confirm whether the binary value represents the correct decimal number. Each bit represented in the binary number is multiplied by 2
to power the bit position value (starting from 0).
= 2^4*(1) + 2^3*(1) + 2^2*(0) + 2^1*(0) + 2^0*(1)
= 16 + 8 + 0 + 0 + 1
= 25
JavaScript Code to Convert Number Into Its Binary Format
We build the following code on the method discussed above. The function convertToBinary1
consoles the binary equivalent of a decimal number passed as a parameter. Note that we read the result backward. Hence the code has been created considering all the afore-said factors in mind.
Using Iteration
function convertToBinary1(number) {
let num = number;
let binary = (num % 2).toString();
for (; num > 1;) {
num = parseInt(num / 2);
binary = (num % 2) + (binary);
}
console.log(binary);
}
window.onload = function() {
console.log(convertToBinary1(25));
console.log(convertToBinary1(8));
}
Output:
11001
1000
Steps
-
Make a copy of the parameter passed to the function and store it in a temporary variable
num
. -
Create a variable to store the binary bits. It essentially is of type string to make handling easier.
-
Start the iteration for generating the binary bits and let it continue till the number is no more divisible by 2.
In each iteration, we divide the number by
2
to get the quotient. We calculate the modulous of the quotient. This step generates the binary bits as modulous of a number with 2 generates the binary bits0
or1
. -
Append the binary bit generated by the modulous value to the binary variable that holds the binary bits generated in each iteration.
-
Once the number is no more divisible by
2
(checked with condition (num / 2) > 1), the iteration stops. -
In the final step, we log the result to the console. And hence we get the binary equivalent for the decimal number passed as a parameter to this function.
Using Recursion
We can use the recursion method to convert the decimal bit into binary. This approach requires fewer lines of code but more thinking. The recursion stops when the number is no more divisible by 2, and it keeps calling itself until it reaches the breakout condition. Recursions are elegant but consume more memory for the function call stack than for the simple iteration approach.
function convertToBinary(number, bin) {
if (number > 0) {
return convertToBinary(parseInt(number / 2)) + (number % 2)
};
return '';
}
window.onload = function() {
console.log(convertToBinary(25));
console.log(convertToBinary(8));
}
Output:
11001
1000
Convert a Number to Binary With toString(2)
Function
The toString()
function is quite familiar for converting a number to a string. But, we can use it to convert a number to its binary format too. Usually, it is used with the Number
object to convert the number to binary format. The toString(2)
function of javascript, when used on a number object, returns the binary equivalent of the numeric value, as depicted in the examples below. The method takes the radix value as input. Hence, we can use it to convert a given number to other base systems (like the base of 16 (hexadecimal) and that of eight (Octal)).
(8).toString(2)(25).toString(2)(235).toString(2)
Output:
"1000"
"11001"
"11101011"
Note that the return type of the toString(2)
method remains a string. Hence, it converts the number to a binary bit in a string format.