How to Validate Input in Java
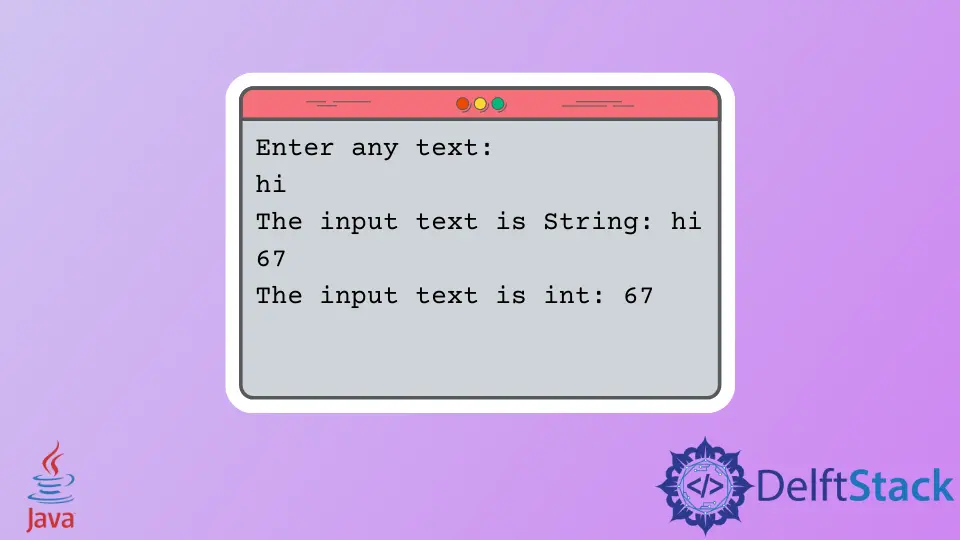
Validation is the process of checking user input or the values from the database against specific constraints. Validation is applied to reduce the time taken by the program for running actual business logic and then finding issues in input from the user. The process increases the performance and hence result in any further debugging sessions in case of failure.
In Java code, we take the user input using the Scanner
class. There are various methods of the Scanner class that helps in input content validation.
Below is the code block that explains the methods.
import java.util.Scanner;
public class InputValidation {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter any text: ");
while (!scanner.hasNext("quit")) {
System.out.println(scanner.hasNextInt() ? "The Input text is int: " + scanner.nextInt()
: scanner.hasNextLong() ? "The Input text is long: " + scanner.nextLong()
: scanner.hasNextDouble() ? "The Input text is double: " + scanner.nextDouble()
: scanner.hasNextBoolean() ? "The Input text is boolean: " + scanner.nextBoolean()
: "The Input text is String: " + scanner.next());
}
}
}
In the above code block, an instance of the Scanner
class gets created. The constructor calls using a new keyword takes an Input Stream parameter, say System.in
.
Now the actual work on the scanner instance gets started. First, in the while condition, a check is applied to give a termination condition. The expression uses the Scanner class hasNext
method. The method takes a pattern to match with the input token. And checks if the input is anything other than the quit
text. The hasNext
method throws IllegalStateException
when the scanner object is closed.
Now when the block gets initiated, the input is validated using the hasNextInt
method. It returns true only if the entered text is int
value. The input text is scanned from the console as an int
using the nextInt
method and printed over the console. The nextInt()
method throws InputMismatchException
when the text does not match the Integer Regex, IllegalStateException
when the scanner instance is closed.
Now the whole of this check block is written in nested ternary statements. If the first condition is evaluated to be false, then another check is applied. The hasNextDouble
method interprets the text as double and returns true if matched. The nextDouble()
method returns a double value from the standard output and prints the same in console otherwise moves for the next check. The nextDouble()
method throws InputMismatchException
when the next token from the console does not match the Float Regex, IllegalStateException
when the scanner instance is closed.
Another check is using the hasNextBoolean
method. This method checks for the boolean
value, true or false. If the returned value is boolean true, the use of the nextBoolean()
is made to take console input and print the same to the output screen. Otherwise, it proceeds with ternary operators else section. The nextBoolean
method throws InputMismatchException
when the next token does not found a Boolean value, IllegalStateException
when the scanner instance is closed.
Following the same pattern, the input gets validated using the next
method. The method becomes the last condition of the while input check section. The method searches and returns the entire ahead text from the console. The function may block the console output while waiting for input to scan. The method always returns a String value. The evaluation treats special characters also as strings and prints in the output. The same exception gets thrown as other methods. Say IllegalStateException
if the scanner is closed or NoSuchElementException
when no more tokens are available.
Below is the output block for the above validation code.
Enter any text:
--
The input text is String: --
67
The input text is int: 67
1234567890000000
The input text is long: 1234567890000000
true
The input text is boolean: true
FALSE
The input text is boolean: false
90.08939782639
The input text is double: 90.08939782639
hi
The input text is String: hi
quit
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn