How to Read Input from System.in in Java
-
Read Input by Using
System.in
in Java -
Read Input Using the
System.in
andBufferedReader
Class in Java -
Read Input Using the
System.console()
Method in Java
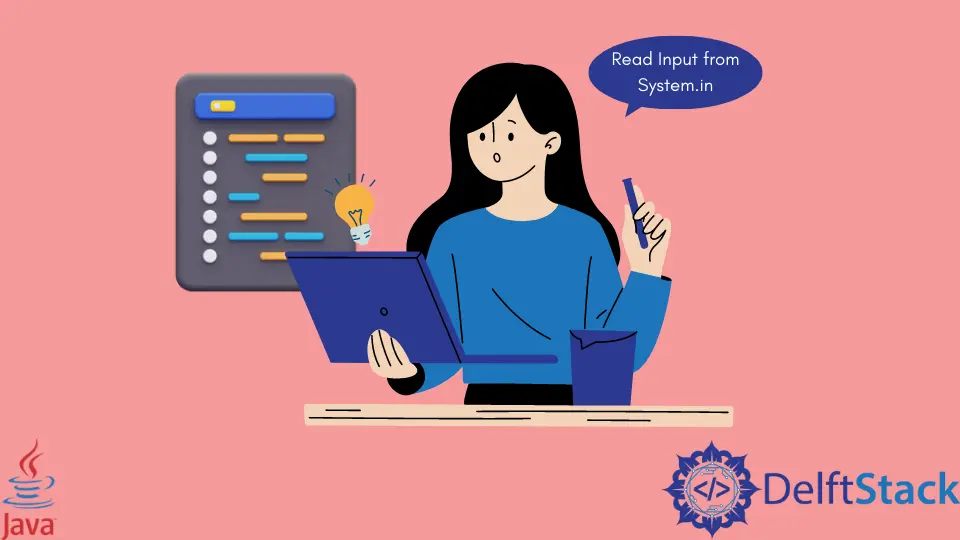
This tutorial introduces how to read user input from the console using the System.in
in Java.
Java provides a low-level stream class System
to read user input, which uses an input stream to read input. The System
is a class in Java that helps to perform system-related tasks.
We can pass this to the Scanner
class, and then by using its methods; we can get user input of several types such as String
, int
, float
, etc. Let’s understand by some examples.
Read Input by Using System.in
in Java
Using the System.in
in a Java code is easy; pass the class in the Scanner
constructor and use the nextLine()
method. This method reads and returns a string.
See the example below.
import java.util.Scanner;
public class SimpleTesting {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter a value :");
String str = sc.nextLine();
System.out.println("User input: " + str);
}
}
Output:
Enter a value :
2
User input: 2
Read Input Using the System.in
and BufferedReader
Class in Java
This is another solution to read user input where we used the BufferedReader
class rather than the Scanner
class. This code does the same task, and we used the readLine()
method here to read the data.
This method belongs to the BufferedReader
class and returns a string. See the example below.
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
System.out.println("Enter a value :");
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String str = br.readLine();
System.out.println(str);
}
}
Output:
Enter a value :
sam
sam
Read Input Using the System.console()
Method in Java
Java System
class provides a console()
method to deal with console-related tasks. So, to read data, we can use this method also.
This method returns a console object by which we can call the readLine()
method to read data. See the example below.
import java.io.Console;
import java.io.IOException;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
Console c = System.console();
System.out.println("Enter a value : ");
String str = c.readLine();
System.out.println(str);
}
}
Output:
Enter a value :
sam
sam
The Java Scanner
class is commonly used to read user data and provides methods for each data type.
We can use these methods to read specific data. Some of them are below.
public int nextInt(); // reads integer input
public float nextFloat(); // reads decimal input
public String nextLine(); // reads string input
In the example below, we used these methods to read a different type of user input in Java. It will help you to understand the Java console.
See the example below.
import java.io.IOException;
import java.util.Scanner;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
Scanner sc = new Scanner(System.in);
System.out.println("Enter a string value : ");
String str = sc.nextLine();
System.out.println(str);
System.out.println("Enter an int value : ");
int a = sc.nextInt();
System.out.println(a);
System.out.println("Enter a float value : ");
float f = sc.nextFloat();
System.out.println(f);
}
}
Output:
Enter a string value :
string
string
Enter an int value :
23
23
Enter a float value :
34
34.0