How to Get User Input in Java
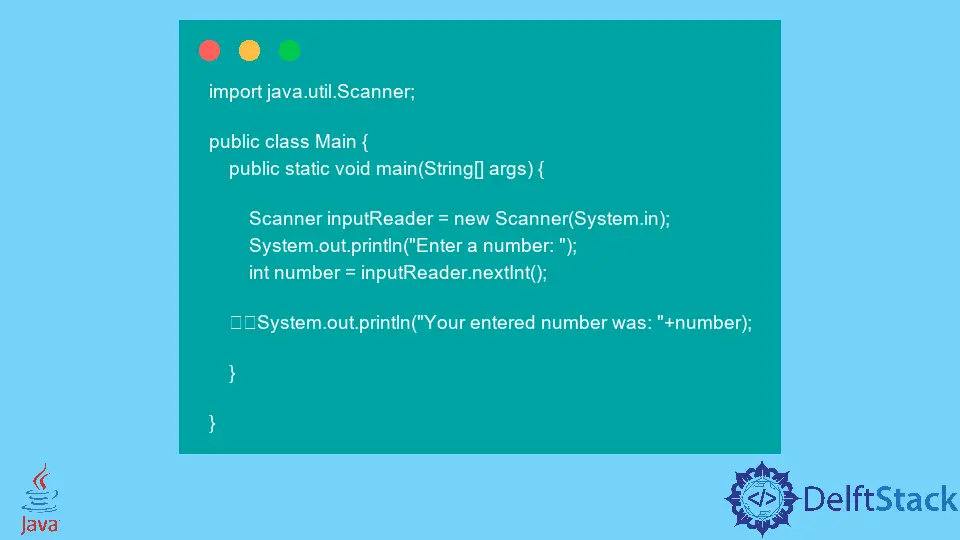
In this article, we will discuss the best approach to get user input in Java. Though there are many ways to do it, some of those methods are already deprecated, and thus we will be avoiding them.
Scanner
to Get User Input in Java
We can use the Scanner
to achieve our goal. We need to create an object of the class and pass System.in
to its constructor because it opens an InputStream
to get input from the user.
The next step is to use the Scanner
object and call one of the following methods. Every method takes the input value of different data types.
Method | Description |
---|---|
next() |
String value |
nextInt() |
Integer value |
nextByte() |
Byte value |
nextLong() |
Long value |
nextFloat() |
Float value |
nextDouble() |
Double value |
In our example below, we will use the nextInt()
method, which takes integer values.
Example:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner inputReader = new Scanner(System.in);
System.out.println("Enter a number: ");
int number = inputReader.nextInt();
System.out.println("Your entered number was: " + number);
}
}
Output:
Enter a number:
454
Your entered number was: 454
BufferedReader
to Get User Input in Java
We have another class that could get the input from the user. BufferedReader
uses character streams to read text from the input, while Scanner
can be used when we the primitive type of input.
It means that it doesn’t have methods like nextInt()
in BufferedReader
class, but has is a readLine()
method that takes input then we can parse it later.
In the below example, we are taking the input as an int
. We have to read the input and then parse it into int
type using Integer.parseInt(String)
. We should surround the statements by a try-catch
block as IOException may appear if there are no external input devices.
Example:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Main {
public static void main(String[] args) throws IOException {
InputStreamReader isr = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(isr);
try {
System.out.println("Please enter a number: ");
String s = br.readLine();
int i = Integer.parseInt(s);
System.out.println("Your entered number was: " + i);
} catch (IOException e) {
e.printStackTrace();
}
br.close();
}
}
Output:
Please enter a number:
454
Your entered number was: 454
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn