How to Wait for Input in Java
-
Java Wait for User Input Using the
nextLine()
Method of theScanner
Class -
Java Wait for User Input Using
BufferedReader
-
Java Wait for User Input Using
JOptionPane
for GUI Applications - Conclusion
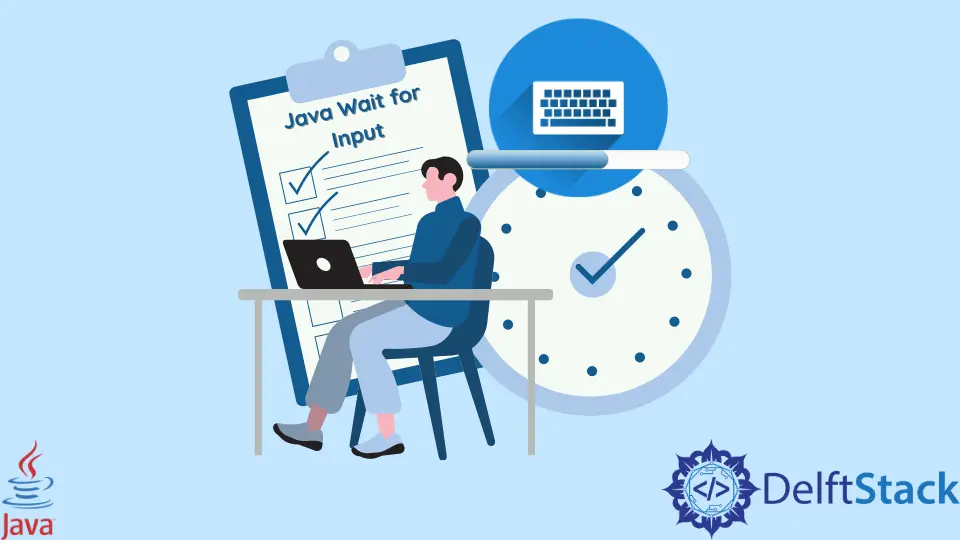
User input plays a crucial role in programming, allowing users to provide data or information to the program. There are scenarios in Java programming where we need the program to pause its execution and wait for the user to input a value.
To achieve this, we can utilize three common approaches to waiting for user input in Java: using the nextLine()
method of the Scanner
class for console input, the BufferedReader
class for console input, and JOptionPane
for GUI input dialogs.
Java Wait for User Input Using the nextLine()
Method of the Scanner
Class
The nextLine()
method is a function available in the java.util.Scanner
class in Java. It is used to advance past the current line in the input and return the input provided by the user as a string.
This method waits for the user to input a valid string and then continues with the program compilation. It’s important to note that this method is specifically applicable to string data types.
Let’s demonstrate how to use the nextLine()
method through an example:
import java.util.Scanner;
public class ScannerExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String userInput = scanner.nextLine();
System.out.println("The line entered by the user: " + userInput);
scanner.close();
}
}
Input:
Hello World.
Output:
The line entered by the user: Hello World.
In the example above, the program will wait for the user to input a line and then display the input provided by the user.
Automatic Blocking of nextLine()
One of the advantages of using the nextLine()
method is that it automatically blocks the program execution until a line of input is available. There’s no need to explicitly wait or check for the availability of input; Scanner.nextLine()
handles this internally.
Let’s explore this through the following code:
import java.util.Scanner;
public class ScannerExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
try {
while (true) {
System.out.println("Please input a line");
long startTime = System.currentTimeMillis();
String line = scanner.nextLine();
long endTime = System.currentTimeMillis();
System.out.printf("Waited %.3fs for user input%n", (endTime - startTime) / 1000d);
System.out.printf("User input was: %s%n", line);
}
} catch (IllegalStateException | NoSuchElementException e) {
System.out.println("System.in was closed; exiting");
}
}
}
Output:
Please input a line
Hello World.
Waited 1.892s for user input
User input was: Hello World.
Please input a line
^D
System.in was closed; exiting
In this enhanced example, we calculated and displayed the time the program waited for user input using the currentTimeMillis()
function.
The IllegalStateException
is raised if the Scanner
is closed, and the NoSuchElementException
is raised if no line is found.
Java Wait for User Input Using BufferedReader
Another effective way to make a Java program pause and wait for user input is by using the BufferedReader
class.
The BufferedReader
class, part of the java.io
package, provides efficient reading of text from a character input stream. It reads characters and stores them in an internal buffer, allowing for more efficient reading by reducing the number of I/O operations.
This buffering improves performance, especially when dealing with input sources that might have slow I/O operations, such as reading from a disk or network.
To use BufferedReader
, you first need to create an instance by wrapping it around an InputStreamReader
that reads from a particular input stream. The most common use case is to read from the standard input stream (System.in
), which is the keyboard for console-based applications.
Here’s how you create a BufferedReader
instance to read from the standard input stream:
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
The readLine()
method of BufferedReader
is fundamental for obtaining user input. This method reads a line of text from the input stream and returns it as a String
.
It waits until the user provides input (presses Enter) and hits return, indicating the completion of their input.
Here’s how you use the readLine()
method to get user input:
try {
System.out.print("Enter your name: ");
String name = reader.readLine();
System.out.println("Hello, " + name + "!");
} catch (IOException e) {
System.err.println("Error reading input: " + e.getMessage());
}
In this example, the program prompts the user to enter their name. The readLine()
method pauses the program’s execution, waiting for the user to input their name and press Enter.
Once the user provides input, it is returned as a String
, and the program continues.
Here’s a complete working example demonstrating the usage of BufferedReader
to obtain user input:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class UserInputExample {
public static void main(String[] args) {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
try {
System.out.print("Enter your name: ");
String name = reader.readLine();
System.out.println("Hello, " + name + "!");
} catch (IOException e) {
System.err.println("Error reading input: " + e.getMessage());
}
}
}
Input:
Enter your name: Jane Doe
Output:
Hello, Jane Doe!
In this example, we create a BufferedReader
instance by wrapping it around the System.in
input stream using InputStreamReader
. We then use the readLine()
method to wait for the user to input a line of text.
When using BufferedReader
, it’s important to handle potential exceptions that may occur during input reading. The readLine()
method may throw an IOException
if an I/O error occurs, so it’s essential to handle this exception appropriately.
Java Wait for User Input Using JOptionPane
for GUI Applications
For graphical user interface (GUI) applications, a commonly used method is the JOptionPane
, which is a part of the Swing framework, which allows developers to display dialog boxes and obtain user input in a user-friendly manner.
These dialog boxes are commonly used to prompt the user for input, display messages, ask for confirmation, or present various options.
To obtain user input using JOptionPane
, you can use the showInputDialog
method. This method displays an input dialog, prompting the user to enter some text or data.
Here’s a basic example demonstrating the usage of JOptionPane
to obtain user input:
import javax.swing.JOptionPane;
public class UserInputExample {
public static void main(String[] args) {
String userInput = JOptionPane.showInputDialog("Enter your name:");
JOptionPane.showMessageDialog(null, "Hello, " + userInput + "!");
}
}
In this example, JOptionPane.showInputDialog
prompts the user to enter their name. The entered name is then displayed in a message dialog using JOptionPane.showMessageDialog
.
The showInputDialog
method returns the input provided by the user as a String
. You can then use this input for further processing or display it in the application.
String userInput = JOptionPane.showInputDialog("Enter your name:");
System.out.println("User input: " + userInput);
If the user cancels the input dialog, showInputDialog
returns null
. You should handle this case accordingly.
String userInput = JOptionPane.showInputDialog("Enter your name:");
if (userInput != null) {
System.out.println("User input: " + userInput);
} else {
System.out.println("User canceled the input.");
}
Below is the complete working code example demonstrating how to use JOptionPane
to obtain user input and display it in a GUI application:
import javax.swing.JOptionPane;
public class UserInputExample {
public static void main(String[] args) {
String userInput = JOptionPane.showInputDialog("Enter your name:");
if (userInput != null) {
JOptionPane.showMessageDialog(null, "Hello, " + userInput + "!");
} else {
JOptionPane.showMessageDialog(null, "You canceled the input.");
}
}
}
When you run this code, a dialog box will appear, prompting the user to enter their name. If the user enters a name and clicks OK
, a greeting message will be displayed.
If the user clicks Cancel
or closes the dialog, a message indicating the cancellation will be shown. Compile and run this Java program to see how JOptionPane
can be used to interact with users in a GUI application.
Conclusion
Obtaining user input is an essential aspect of programming, and Java offers multiple methods to achieve this based on the requirements of your application.
Whether you are building a console-based application or a graphical user interface, using the appropriate approach—Scanner
for console input, BufferedReader
for enhanced console input, or JOptionPane
for GUI input dialogs—allows you to interact with users effectively and provide a seamless user experience in your Java applications.
Choose the method that best fits the context of your application and the type of user interaction you wish to achieve.