Java 等待輸入
Siddharth Swami
2023年10月12日
Java
Java Input
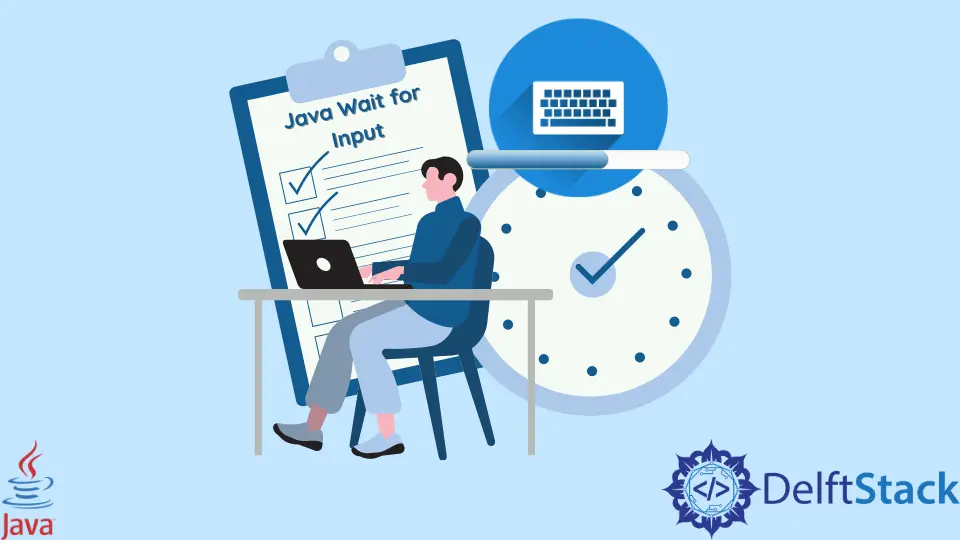
使用者輸入可以指使用者希望編譯器處理的任何資訊或資料。我們可能會遇到希望程式暫停編譯並等待使用者輸入某個值的情況。
對於這種情況,我們可以使用 nextLine()
函式。
在本教程中,我們將學習如何使用 nextLine()
方法讓 Java 等待使用者輸入。
nextLine()
函式位於 Java 的 java.util.Scanner
類中。此函式用於移過當前行並返回一些輸入。
因此,通過使用此方法,編譯器等待使用者輸入有效字串並繼續編譯程式。此方法僅適用於字串資料型別。
例如,
// Java program to illustrate the
// nextLine() method of Scanner class in Java
import java.util.Scanner;
public class Scanner_Class {
public static void main(String[] args) {
// create a new scanner
// with the specified String Object
Scanner scanner = new Scanner(System.in);
String s = scanner.nextLine();
// print the next line
System.out.println("The line entered by the user: " + s);
scanner.close();
}
}
輸入:
Hello World.
輸出:
The line entered by the user: Hello World.
無需等待檢查輸入的可用性,因為 Scanner.nextLine()
將自動阻塞,直到有一行可用。
下面的程式碼解釋了這一點。
import java.util.Scanner;
public class Scanner_Test {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
try {
while (true) {
System.out.println("Please input a line");
long then = System.currentTimeMillis();
String line = scanner.nextLine();
long now = System.currentTimeMillis();
System.out.printf("Waited %.3fs for user input%n", (now - then) / 1000d);
System.out.printf("User input was: %s%n", line);
}
} catch (IllegalStateException | NoSuchElementException e) {
// System.in has been closed
System.out.println("System.in was closed; exiting");
}
}
}
輸出:
Please input a line
Hello World.
Waited 1.892s for user input
User input was: Hello World.
Please input a line
^D
System.in was closed; exiting
在上面的例子中,我們使用 currentTimeMillis()
函式計算並顯示了編譯器等待輸入的時間。
這個函式可能會返回兩個異常。關閉 Scanner
時會引發 IllegalStateException
,當找不到行時會引發 NoSuchElementException
。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe