The Ternary Operator in TypeScript
- TypeScript Operators
- Use the Ternary Operator in TypeScript
- Implement Nested Conditions With the Ternary Operator in TypeScript
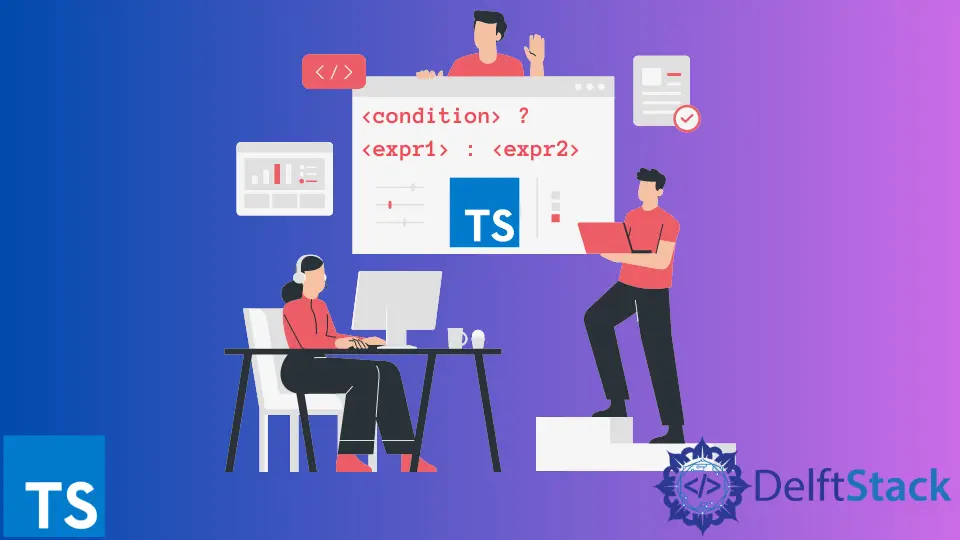
In this article, we will briefly introduce the different operators in TypeScript and discuss the ternary operators and how to use them.
TypeScript Operators
Software applications are intended to work with data. Hence, they have designed a way to perform different operations on this data.
Each operation works with one or more data values and generates a final result. These operations can be divided into various groups.
Operands and Operators in TypeScript
Usually, an operator operates on at least one data value called an operand. For example, in the expression 500 + 200
, the values 500
and 200
are two operands, and the +
is the operator.
Several operators can be seen in TypeScript. These can be divided into groups based on the nature of each operator’s operation, such as arithmetic, logical, bitwise, relational, etc.
Also, these operators can be grouped based on the number of operands each operator expects. The binary operator has two operands, as shown in the following.
Example:
x * y
20 / 2
The unary operator takes only one operand.
Example:
x++
y--
TypeScript language supports a ternary operator that operates on three operands; it is the shortened format of the if...else
syntax. We call it the TypeScript conditional operator.
Use the Ternary Operator in TypeScript
The TypeScript conditional operator takes three operands. The first is the condition to be evaluated; it can be identified as the if()
part in the usual if...else
syntax.
The next two operands are the two expressions to be executed based on the evaluated condition results. Hence, the second operand is the expression to be executed when the condition is evaluated to true
.
Otherwise, the third operand expression is returned.
Syntax:
<your_condition> ? <expression_A> : <expression_B>
Where:
<your_condition>
is the condition to be evaluated. It is a boolean expression that returns eithertrue
orfalse
.<expression_A>
is the expression to be returned when the condition istrue
.<expression_B>
is the expression to be returned when the condition isfalse
.
The conditional operator is the only ternary operator available in the TypeScript language.
Let’s write a TypeScript code to check the user’s age, which will return a message based on that. First, we will write the conditional logic using ordinary if...else
.
const MAX_ALLOWED_AGE = 18;
let userAge = 15;
let finalMessage = '';
if( userAge >= MAX_ALLOWED_AGE ) {
finalMessage = 'You are allowed to this site';
} else {
finalMessage = 'Get Back!!'
}
console.log(finalMessage);
Output:
The same logic can be written more compactly using the ternary operator.
const MAX_ALLOWED_AGE = 18;
let userAge = 15;
let finalMessage = userAge >= MAX_ALLOWED_AGE ? 'You are allowed to this site' : 'Get Back!!';
console.log(finalMessage);
You will be getting the same output as in the above if...else
logic. This is fewer lines than the if...else
syntax and is cleaner.
Implement Nested Conditions With the Ternary Operator in TypeScript
The ternary operator is not limited to a single condition. It also supports multiple conditions.
Let’s look at the nested if...else
conditional logic, as shown in the following.
let studentMark = 68;
const GRADE_A_MARK = 75;
const GRADE_B_MARK = 61;
let finalMessage = '';
if( studentMark >= GRADE_A_MARK ) {
finalMessage = 'Great work!';
} else if(studentMark >= 61 && studentMark < 75) {
finalMessage = 'Good work!';
} else {
finalMessage = 'Study more!!!';
}
console.log(finalMessage);
Output:
Let’s write the nested conditions above using the ternary operator.
let studentMark = 68;
const GRADE_A_MARK = 75;
const GRADE_B_MARK = 61;
let finalMessage = studentMark >= GRADE_A_MARK ? 'Great work!' : studentMark >= 61 && studentMark < 75 ? 'Good work!' : 'Study more!!!';
console.log(finalMessage);
If you transpile the above TypeScript code and run it using node, you will be getting the same output as in the above if...else
scenario.
It is recommended to use a conditional operator in your code. It is a one-line expression that makes your code cleaner.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.