Exclamation Mark in TypeScript
-
Use the
!
Operator to Assert Non-Null in TypeScript -
Example Usage of the
!
Operator in TypeScript -
Harmful Effects of the
!
Operator in TypeScript
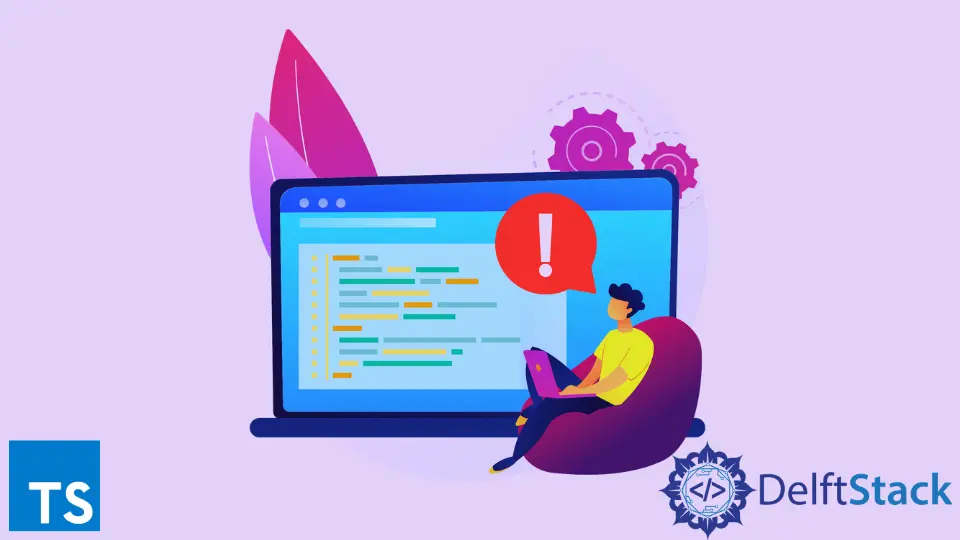
TypeScript is a strongly-typed language that supports creating both user-defined and native types. Apart from types, there are also null
and undefined
, which determine the intentional or unintentional absence of a value in a variable.
TypeScript will complain if a null
value is assigned to a typed variable. This is where the exclamation or bang or !
operator comes into use to force the compiler to interpret the value as not null.
This tutorial will focus on how to use the !
operator.
Use the !
Operator to Assert Non-Null in TypeScript
The !
operator can force the compiler to assert the value as not null
or not undefined
, although the value type may be null or is a union of types with null
being one of the types. This is done on the user’s part when it is known that the value will never be null
.
The !
operator is a TypeScript syntax removed when transcompiling to JavaScript. It can be thought of as similar assertion operators like as
.
The following basic example will show how the !
can be used.
function getValue() : number | undefined {
return 3;
}
var realValue : number | undefined;
realValue = getValue();
var value : number;
value = realValue!;
console.log(value);
Output:
3
The getValue()
function can be a heavy computational call, but always sure to return a number
type. Without the !
operator, TypeScript will show errors like Type 'number | undefined' is not assignable to type 'number'
.
The !
operator forces the compiler to assert the value as not null and not to throw an error henceforth.
Example Usage of the !
Operator in TypeScript
An example where the !
operator can be used is given in the code segment.
interface Fruit {
code : string;
fruit : string;
}
const fruits : Fruit[] = [
{
code : "ORA",
fruit : "Orange"
},
{
code : "APL",
fruit : "Apple"
},
{
code : "GRA",
fruit : "Grapes"
},
{
code : "LITC",
fruit : "Litchi"
}
]
const findFruit = ( fruitToFilter : string ) => {
return fruits.find( fruit => fruit.code === fruitToFilter);
}
const fruit : Fruit = findFruit("APL")!;
console.log(fruit);
Output:
{
"code": "APL",
"fruit": "Apple"
}
The find
operation returns the type string | undefined
. The compiler is forced to treat it as a string and not consider the undefined
as the user is sure that the value returned will not be undefined
.
Harmful Effects of the !
Operator in TypeScript
The user provides the !
operator and forces the compiler to consider the value as not null
. If the value returned was undefined
or null
, the compiler will not catch any errors, and the application may face runtime errors.
So the !
operator should be avoided and only used when it is guaranteed that the value returned will not be null
or undefined
.