Question Mark Operator in TypeScript
-
Use the Question Mark (
?
) Operator in TypeScript -
Use the Question Mark
(?)
to Check forundefined
in TypeScript
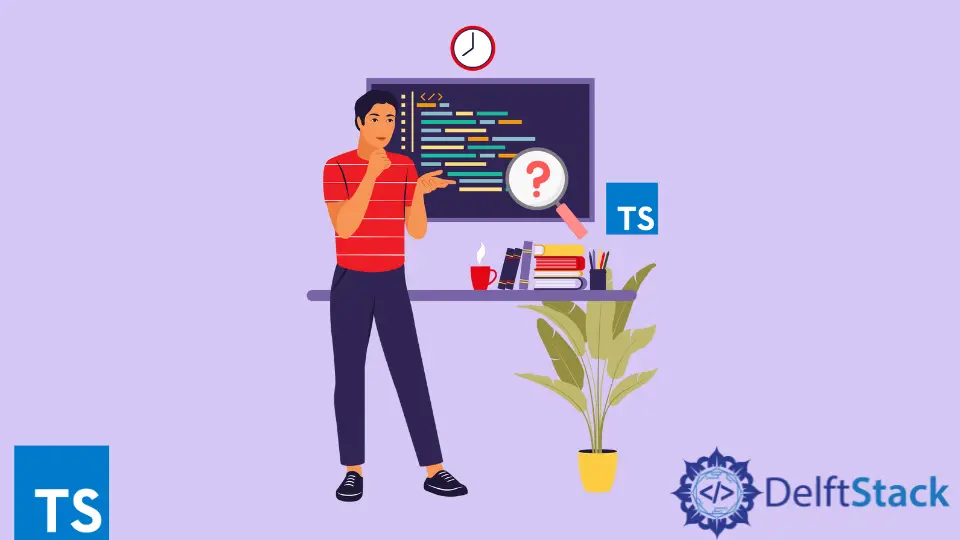
TypeScript has strict matching policies for type checking. The question mark or ?
has relieved the users by defining optional parameters.
Moreover, the ?
operator can also act as a shorthand for checking if a certain attribute of an object is null
or undefined
. We will discuss the use of the question mark operator in TypeScript in this article.
Use the Question Mark (?
) Operator in TypeScript
The parameter? : type
is a shorthand for parameter: type | undefined = undefined
. Thus it gives a convenient shortcut for assigning undefined parameters.
Code Snippet:
interface Props{
name : string ;
age? : number ;
}
function getDetails(props : Props){
if ( props.age !== undefined){
console.log("Hi my name is " + props.name + " , and my age is " + props.age.toString() );
} else {
console.log("Hi my name is " + props.name );
}
}
getDetails({ name : "Geralt"});
getDetails( { name : "Geralt", age : 95});
Output:
"Hi my name is Geralt"
"Hi my name is Geralt , and my age is 95"
The optional field in the Props
interface allowed the age attribute to be empty while passing it to the getDetails
function. It is also applicable for functions with arguments.
Let’s have another example without the Props
interface.
Code Snippet:
function getDetails(name :string, age? : number){
if ( age === undefined){
console.log("Name : " + name);
} else {
console.log("Name : " + name + ", Age : " + age.toString());
}
}
getDetails("Geralt");
getDetails("Geralt", 95);
Output:
"Name : Geralt"
"Name : Geralt, Age : 95"
The above function definition can also be written in the long-form as:
function getDetails(name :string, age : number | undefined = undefined){
...
}
Use the Question Mark (?)
to Check for undefined
in TypeScript
The question mark or ?
operator can also be used to check if a certain attribute of an object is null
or undefined
.
Syntax:
user?.name
The above TypeScript code trans-compiles to JavaScript as:
user === null || user === undefined ? undefined : user.name;
The ?
operator can also be chained to check for repeated null
or undefined
checks.
Code Snippet:
interface User {
user : {
name : string;
}
}
interface Data {
data : User
}
// faulty data from an API call
let d : Data = {
data : {
user : undefined
}
}
let userName = d?.data?.user?.name ?? 'default name';
console.log(userName);
Output:
"default name"
Thus some faulty data from an API call may have some undefined
fields. The above code segment shows how to chain the ?
operator to have null
and finally use the ??
operator if an undefined
was returned.