How to Filter an Array in TypeScript
- Using the filter Method
- Filtering Objects in an Array
- Chaining filter with Other Array Methods
- Conclusion
- FAQ
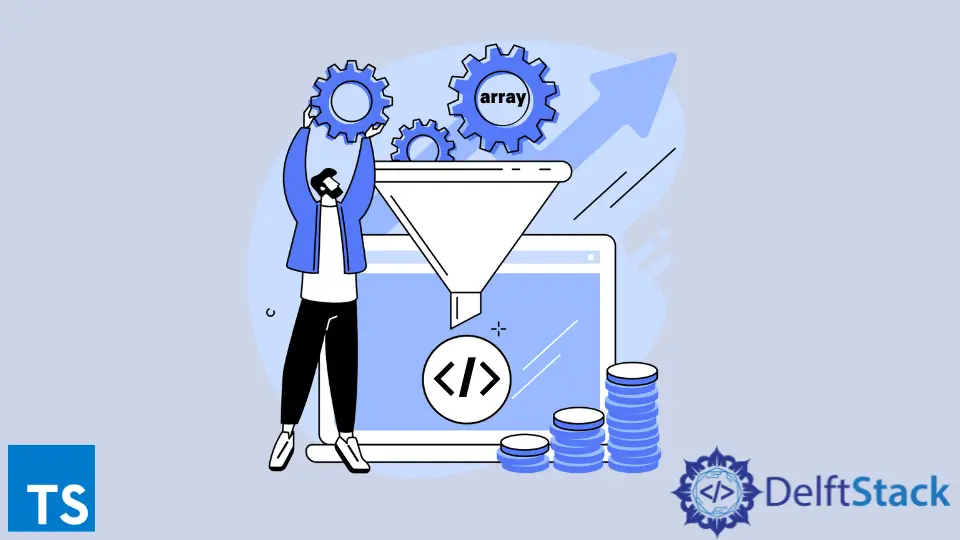
Filtering an array is a fundamental operation in programming that allows developers to extract specific elements based on defined criteria. In TypeScript, which builds upon JavaScript, filtering an array is both straightforward and powerful.
This tutorial will guide you through the various methods of filtering arrays in TypeScript, providing clear examples to help you understand each approach. Whether you’re dealing with numbers, strings, or objects, you’ll find the techniques covered here invaluable for enhancing your TypeScript skills. By the end of this article, you’ll be equipped to efficiently filter arrays in your TypeScript projects, improving code readability and functionality.
Using the filter Method
One of the most common ways to filter an array in TypeScript is by using the built-in filter
method. This method creates a new array with all elements that pass the test implemented by the provided function. It’s particularly useful when you want to retain elements that meet specific conditions.
Here’s how you can use the filter
method:
const numbers: number[] = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evenNumbers: number[] = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers);
Output:
[2, 4, 6, 8, 10]
In this example, we have an array of numbers from 1 to 10. The filter
method is called on the numbers
array, with a callback function that checks if each number is even (i.e., divisible by 2). The result is a new array, evenNumbers
, which contains only the even values from the original array. This method is concise and leverages the functional programming paradigm, making your code cleaner and easier to understand.
Filtering Objects in an Array
When working with arrays of objects, you might want to filter based on specific object properties. The filter
method can still be used effectively in this scenario. Let’s say you have an array of user objects and you want to filter out users based on their age.
Here’s an example:
interface User {
name: string;
age: number;
}
const users: User[] = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 35 },
{ name: 'David', age: 20 }
];
const filteredUsers: User[] = users.filter(user => user.age >= 30);
console.log(filteredUsers);
Output:
[
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 35 }
]
In this code snippet, we define a User
interface to represent user objects. We then create an array of users with different ages. By using the filter
method, we can easily extract users who are 30 years old or older. The resulting array, filteredUsers
, contains only the users that meet this age criterion. This demonstrates how TypeScript’s type system can enhance the clarity and safety of your code while filtering complex data structures.
Chaining filter with Other Array Methods
One of the powerful features of the filter
method is that it can be chained with other array methods like map
and reduce
. This allows for more complex data manipulations in a clean and readable manner. For instance, you might want to filter an array and then transform the filtered results.
Here’s an example that combines both filter
and map
:
const products = [
{ name: 'Laptop', price: 1000 },
{ name: 'Phone', price: 500 },
{ name: 'Tablet', price: 750 },
{ name: 'Monitor', price: 300 }
];
const expensiveProducts = products
.filter(product => product.price > 600)
.map(product => product.name);
console.log(expensiveProducts);
Output:
['Laptop', 'Tablet']
In this scenario, we have an array of product objects, each with a name and a price. First, we filter the products to include only those that cost more than 600. Then, we use map
to create a new array containing just the names of those expensive products. The final result is an array of product names that meet the filtering criteria. This method showcases the flexibility and power of functional programming in TypeScript, enabling developers to write clean and efficient code.
Conclusion
Filtering arrays in TypeScript is a vital skill that can significantly enhance your programming capabilities. By utilizing the filter
method, you can easily extract elements from arrays based on specific conditions, whether dealing with primitive data types or complex objects. Combining filter
with other array methods like map
allows for even more powerful data manipulation. As you continue to explore TypeScript, mastering these techniques will undoubtedly improve your code quality and efficiency. So go ahead, apply these methods in your projects, and watch your TypeScript skills soar!
FAQ
-
What is the filter method in TypeScript?
The filter method creates a new array with all elements that pass the test implemented by the provided function. -
Can I filter arrays of objects in TypeScript?
Yes, you can filter arrays of objects based on specific properties using the filter method. -
How do I combine filter with other array methods in TypeScript?
You can chain the filter method with other array methods like map and reduce to perform complex data manipulations. -
Is TypeScript’s filter method similar to JavaScript’s filter?
Yes, TypeScript’s filter method operates the same way as JavaScript’s filter, as TypeScript is a superset of JavaScript. -
Can I use filter with asynchronous operations in TypeScript?
The filter method itself is synchronous, but you can use it with asynchronous operations by combining it with async/await or Promises in a different approach.