How to Change Location of Legend in Seaborn Plot
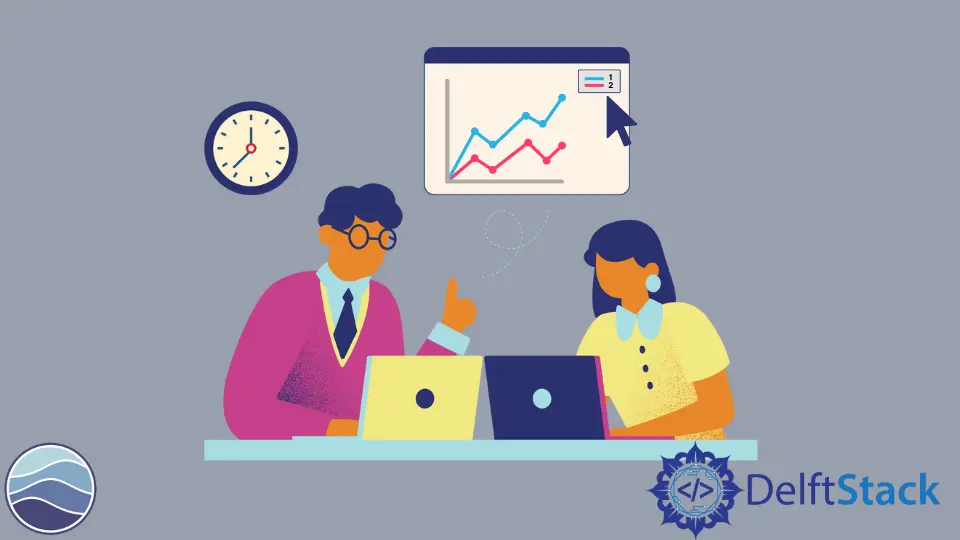
In this tutorial, we will learn how to change the location of the legend in a seaborn plot in Python.
For this, we will need to disable the default legend. This can be done by setting the legend
parameter as False in the plot function. We will explicitly add the legend using the matplotlib.pyplot.legend()
function from the matplotlib library.
To alter the position of the legend, we will use the loc
parameter from this function. We can specify the required location of the legend as the value of this parameter.
For example,
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
sns.lineplot(data=df, legend=False)
plt.legend(labels=["Day1", "Day2"], loc="upper right")
We can also specify different numbers as the value for this parameter. Different numbers correspond to different locations. For example, 1 for the upper right position, 2 specifies the upper left position, etc. Refer to this Matplotlib article to get the table of the relationship between numbers and positions.
By default, the value of the loc
parameter is 0, which means it searches for the position within the plot so that minimum overlapping takes place.
We can also place the legend box outside the plot by using the bbox_to_anchor
parameter. The bbox_to_anchor
specifies the position of the legend with respect to the location specified in the loc
parameter. If we set the bbox_to_anchor
parameter with a two-elements tuple, then it considers their values as the x and y values for positioning along the specified loc
.
For example,
import seaborn as sns
import pandas as pd
import matplotlib.pyplot as plt
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
sns.lineplot(data=df, legend=False)
plt.legend(labels=["Day1", "Day2"], loc=2, bbox_to_anchor=(1, 1))
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn