How to Add and Customized Legend in Seaborn Plot
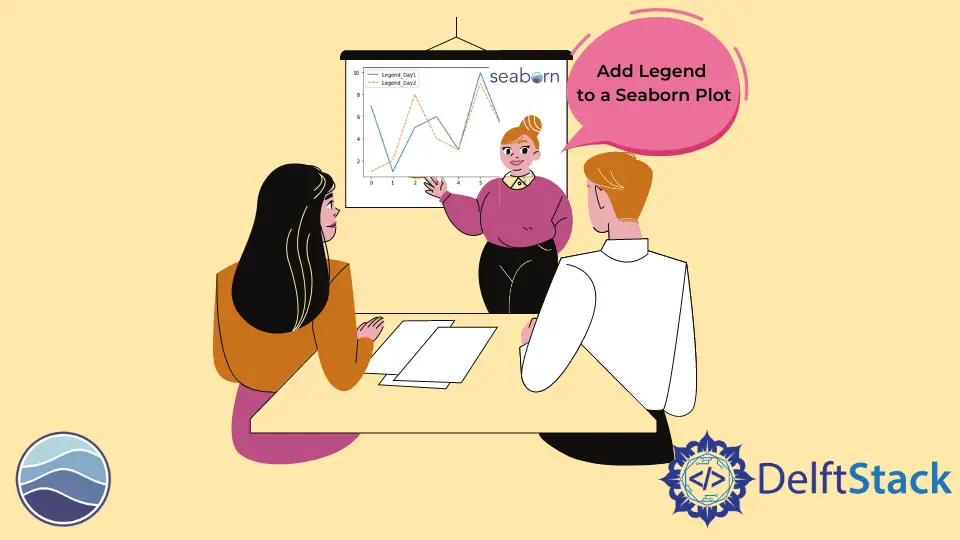
A legend is usually a small box, which appears at some corner of your graph and is used to tell about the different elements of the plot. And if there are multiple data in the graph, then it tells which component represents which data.
In this tutorial, we will learn how to add or customize a legend to a simple seaborn plot.
By default, seaborn automatically adds a legend to the graph.
For example,
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
sns.lineplot(data=df)
Notice the legend is at the top right corner.
If we want to explicitly add a legend, we can use the legend()
function from the matplotlib library. In this way, we can add our own labels explicitly.
For example,
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
sns.lineplot(data=df)
plt.legend(labels=["Legend_Day1", "Legend_Day2"])
Note that the seaborn library is based on and uses the matplotlib module to create its graphs. So we can use the legend()
function for seaborn plots as well.
We can also perform small customizations on the legend. For example, we can add a title to the legend using the title
parameter in the legend()
function, as shown below.
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
sns.lineplot(data=df)
plt.legend(labels=["Legend_Day1", "Legend_Day2"], title="Title_Legend")
The fontsize
and title_fontsize
are the two parameters that are used to alter the font of the content in the legend and its title, respectively.
See the code below.
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
sns.lineplot(data=df)
plt.legend(
labels=["Legend_Day1", "Legend_Day2"],
title="Title_Legend",
fontsize="large",
title_fontsize="10",
)
Note that the title_fontsize
is not present in every version of matplotlib so kindly check your version before using it.
We can specify the size and position of the legend box using the loc
and bbox_to_anchor
parameters.
loc
is used to specify the location of the legend. Different numbers specify different locations. Its value is 0 by default, which means that it searches for the best position for placing legend where minimum overlapping happens.
The bbox_to_anchor
specifies the position of the legend with respect to the location specified in the loc
parameter. If we set the bbox_to_anchor
parameter with a 2 elements tuple, then it considers their values as the x and y values for positioning along the specified loc
.
For example,
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
sns.lineplot(data=df)
plt.legend(labels=["Legend_Day1", "Legend_Day2"], loc=2, bbox_to_anchor=(1, 1))
Note that the value of the loc
argument is 2, which indicates the upper left location.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn