How to Sort an Array in Scala
-
Use the
sorted
Method to Sort an Array in Scala -
Use the
sortBy(attribute)
Method to Sort an Array in Scala -
Use the
sortWith
Method to Sort an Array in Scala
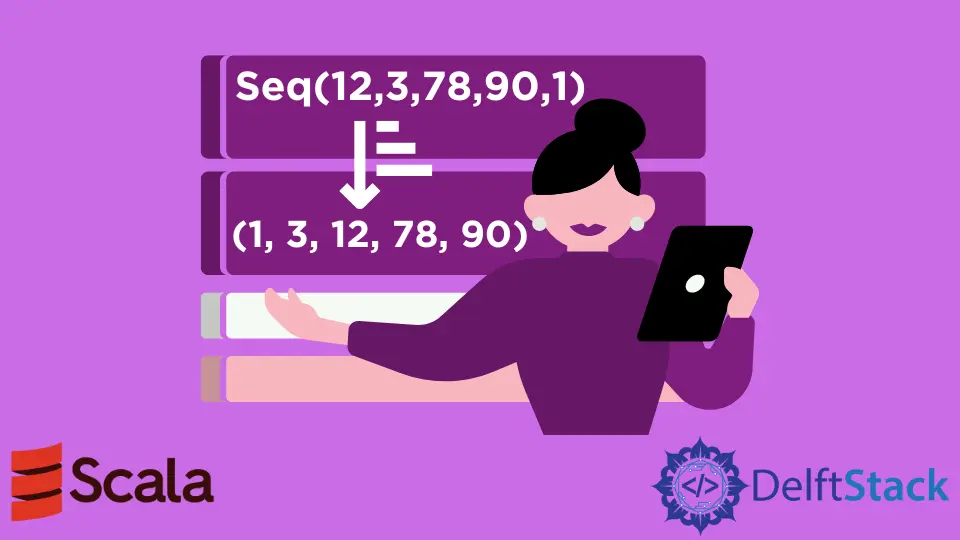
In this article, we will learn how to sort an array of data in the Scala programming language.
Sorting refers to arranging the data in ascending or descending order based on some condition. It’s a very commonly used method when we want to search something in a large dataset, as we can apply the binary search algorithm, which works only on the sorted dataset.
Scala sorting methods internally use TimSort, a hybrid of Merge Sort and Insertion sort algorithm. Now, let’s look at three sorting methods present in Scala.
Use the sorted
Method to Sort an Array in Scala
The sorted
method is used to sort the sequences in Scala like List, Array, Vector, and Seq. This method returns a new collection sorted by its natural order.
Method definition:
def sorted[Y >:X] (implicit ord:Ordering[Y]): Repr
Here, X
refers to the type of element we use in the collection. Repr
is the type of actual collection having the elements.
Let’s see an example:
object MyClass {
def main(args: Array[String]) {
val seq = Seq(12,3,78,90,1)
println(seq.sorted)
}
}
Output:
List(1, 3, 12, 78, 90)
By default, sorting is done in ascending order. If we want to sort the data in descending order, we can use the syntax:
sorted(Ordering.DataType.reverse)
Example code:
object MyClass {
def main(args: Array[String]) {
val seq = Seq(12,3,78,90,1)
println(seq.sorted(Ordering.Int.reverse))
}
}
Output:
List(90, 78, 12, 3, 1)
If we want to use the sorted
method to sort the data based on some attribute of a case class, then we have to extend the Ordered
trait and then override the abstract method compare
. Inside the compare
method, we have to define which attribute we want to sort the objects of the case class.
This is called custom data sorting, i.e., specifying our conditions to sort the data.
Example code:
case class student(id: Int,name: String) extends Ordered[student]
{
def compare(that: student) = this.name compare that.name
}
val obj1 = student(1, "tony")
val obj2 = student(2, "bruce")
val obj3 = student(3, "stark")
val studentList = List(obj1,obj2,obj3)
println(studentList.sorted)
Output:
List(student(2,bruce), student(3,stark), student(1,tony))
In the above code, we have sorted the objects based on the name
attribute of the case class student
.
Use the sortBy(attribute)
Method to Sort an Array in Scala
The sortBy
method in Scala can sort based on one or more class attributes. This method can be used if we have an Ordering
field type in the scope.
Here, the default sorting is ascending as well.
Method definition:
def sortBy[B](f: A => B)(implicit ord: Ordering[B]): Repr
Example code:
case class employee(id: Int, name: String, salary: Double)
val obj1 = employee(1,"tony",12000.00)
val obj2 = employee(2,"bruce",11000.00)
val obj3 = employee(3,"stark",15000.00)
val empList = List(obj1,obj2,obj3)
println(empList.sortBy(_.name))
println(empList.sortBy(_.salary))
Output:
List(employee(2,bruce,11000.0), employee(3,stark,15000.0), employee(1,tony,12000.0))
List(employee(2,bruce,11000.0), employee(1,tony,12000.0), employee(3,stark,15000.0))
In the above code, first, we printed the sorted data based on the attribute name
, and in the second print statement, we sorted the data based on the attribute salary
.
We can sort the data in descending order by tweaking the method a bit into:
sortBy(_.attribute)(Ordering[data_type_of_attribute].reverse)
Example code:
case class employee(id: Int, name: String, salary: Double)
val obj1 = employee(1,"tony",12000.00)
val obj2 = employee(2,"bruce",11000.00)
val obj3 = employee(3,"stark",15000.00)
val empList = List(obj1,obj2,obj3)
println(empList.sortBy(_.name)(Ordering[String].reverse))
Output:
List(employee(1,tony,12000.0), employee(3,stark,15000.0), employee(2,bruce,11000.0))
We sorted the data based on the name
attribute in the above code.
This method is also useful for sorting data based on multiple attributes; it works by sorting data based on the first attribute. If the first attribute has the same values, it is sorted based on the second attribute.
Example code:
case class employee(id: Int, name: String, salary: Double)
val obj1 = employee(1,"tony",12000.00)
val obj2 = employee(2,"bruce",11000.00)
val obj3 = employee(3,"stark",15000.00)
val obj4 = employee(4,"tony",9000.0)
val obj5 = employee(5,"stark",19000.0)
val obj6 = employee(6,"tony",10000.0)
val empList = List(obj1,obj2,obj3,obj4,obj5,obj6)
println(empList.sortBy((empList =>(empList.name,empList.salary))))
In the output, we can see that if the name
is the same, then the data is sorted based on the salary
attribute.
Output:
List(employee(2,bruce,11000.0), employee(3,stark,15000.0), employee(5,stark,19000.0), employee(4,tony,9000.0), employee(6,tony,10000.0), employee(1,tony,12000.0))
Using this method, we can also sort a list of tuples
based on the first or the second element. Below is an example of sorting the tuples based on the second element.
Example code:
val list = List(('b',60),('c',10),('a',40))
println(list.sortBy(_._2))
Output:
List((c,10), (a,40), (b,60))
In the same way, we can sort based on their first element as well.
Example code:
val list = List(('b',60),('c',10),('a',40))
println(list.sortBy(_._1))
Output:
List((a,40), (b,60), (c,10))
Use the sortWith
Method to Sort an Array in Scala
The sortWith(function)
is very useful when we want to forget about the ordering and sort elements based on the given comparison function. So, we can pass any custom comparison function.
Method definition:
def sortWith(lt: (X, X) => Boolean): Repr
Example code 1:
Here, we have sorted the employees from highest to lowest salary.
case class employee(id: Int, name: String, salary: Double)
val obj1 = employee(1,"tony",12000.00)
val obj2 = employee(2,"bruce",11000.00)
val obj3 = employee(3,"stark",15000.00)
val obj4 = employee(4,"naruto",200)
val empList = List(obj1,obj2,obj3,obj4)
println(empList.sortWith(_.salary > _.salary))
Output:
List(employee(3,stark,15000.0), employee(1,tony,12000.0), employee(2,bruce,11000.0), employee(4,naruto,200.0))
Example code 2:
Here, we pass our custom function in the sortWith
method.
case class employee(id: Int, name: String, salary: Double)
def sortBySalary(emp1 :employee,emp2:employee): Boolean =
{
emp1.salary < emp2.salary
}
val obj1 = employee(1,"tony",12000.00)
val obj2 = employee(2,"bruce",11000.00)
val obj3 = employee(3,"stark",15000.00)
val obj4 = employee(4,"naruto",200)
val empList = List(obj1,obj2,obj3,obj4)
println(empList.sortWith((emp1,emp2) => sortBySalary(emp1,emp2)))
Output:
List(employee(4,naruto,200.0), employee(2,bruce,11000.0), employee(1,tony,12000.0), employee(3,stark,15000.0))
We have sorted the data in ascending order based on the salary
in the above code.