How to Print Array in Scala
-
Use a
for
Loop to Print Array in Scala -
Use a
foreach
Loop to Print Array in Scala -
Use the
mkstring()
Method to Print Array in Scala -
Use a
while
Loop to Print Array in Scala - Converting Array to Another Scala Collection
-
Use the
ScalaRunTime
Object to Print Array in Scala - Conclusion
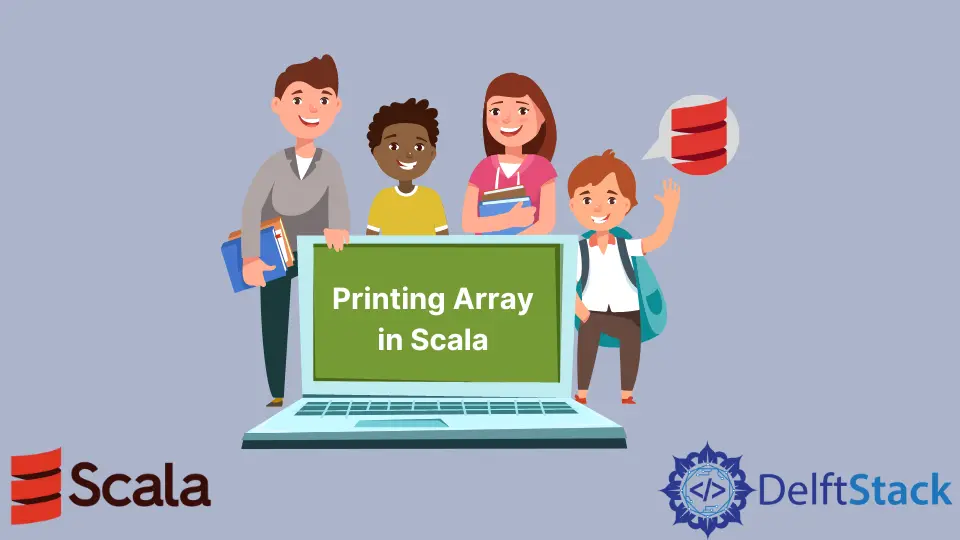
An array in Scala is equivalent to Java’s native arrays. When you print an array in Scala, the default output is often the result of calling the Array.toString
method, which displays the object’s hash code.
This article explores various methods to print array elements in Scala.
Use a for
Loop to Print Array in Scala
The most common approach to printing arrays in Scala is using a for
loop. It iterates through array elements and prints them one by one, using array indices to access elements.
Syntax:
for(i <- 0 to array_name.length-1)
print(array_name[i])
The syntax is a for
loop that iterates through the indices of an array named array_name
, and for each index i
, it prints the corresponding element from the array.
Example Code:
object MyClass {
def main(args: Array[String]): Unit = {
var marks = Array(97, 100, 69, 78, 40, 50, 99)
println("Array elements are : ")
for (i <- 0 to marks.length - 1) {
print(marks(i) + " ")
}
}
}
First, we initialize an array named marks
with some integer values (97, 100, 69, 78, 40, 50, 99
). Print a message indicating that we’re displaying the array elements.
Then, use a for
loop to iterate through the indices of the marks
array. Access each element in the marks
array and print it with a space.
Output:
Array elements are :
97 100 69 78 40 50 99
It displays the elements of the marks
array, separated by spaces.
Use a foreach
Loop to Print Array in Scala
You can also use a foreach
loop to print array elements. Inside foreach
, you can use print
or printf
for a single-line output.
Syntax:
array_name.foreach(println)
In Scala, array_name.foreach(println)
is another way to iterate through each element in an array called array_name
and print each element to the console using the println
function.
Example Code:
object MyClass {
def main(args: Array[String]): Unit = {
var marks = Array(97, 100, 69, 78, 40, 50, 99)
println("Array elements are : ")
marks.foreach(println)
}
}
We initialize an array named marks
with integer values (97, 100, 69, 78, 40, 50, 99
). A message to indicate that we’re going to display the array elements.
Next, the foreach()
method is used on the marks
array. It iterates through each element in the array.
Inside the foreach
loop, the println
function is applied to each element, which means each element is printed to the console on a separate line.
Output:
Array elements are :
97
100
69
78
40
50
99
The output displays the array elements, one per line.
The following example demonstrates a looping concept that you can apply to multidimensional arrays as well.
Example Code:
object MyClass {
def main(args: Array[String]): Unit = {
var arr = Array(Array(11, 22, 33, 44, 55),
Array(111, 222, 333, 444, 555))
for (i <- 0 to 1) {
for (j <- 0 to 4) {
print(" " + arr(i)(j))
}
println()
}
}
}
We initialize a two-dimensional array named arr
, which contains two arrays of integers. This creates a matrix-like structure with rows and columns.
We use nested for
loops to iterate through the elements of the two-dimensional array.
The outer loop (for (i <- 0 to 1)
) iterates over the rows. The 'i
’ takes values 0
and 1
, corresponding to the two rows of arr
.
The inner loop (for (j <- 0 to 4)
) iterates over the columns. The 'j'
takes values from 0
to 4
, covering the five columns in each row.
Inside the inner loop, we print the element at the current row 'i'
and column 'j'
of the arr
array. We add a space as a prefix to separate elements and make the output more readable.
After printing all elements in a row (inside the inner loop), we print an empty line using println()
to separate rows in the output, creating a matrix-like representation in the console.
Output:
11 22 33 44 55
111 222 333 444 555
The output displays the elements of the two-dimensional array in a grid-like format, with each row separated by an empty line.
Use the mkstring()
Method to Print Array in Scala
You can convert the array to a string and use the mkString()
method to print it. This method converts array elements to string representations.
Example Code:
object MyClass {
def main(args: Array[String]): Unit = {
var marks = Array(97, 100, 69, 78, 40, 50, 99)
print(marks.mkString(", "))
}
}
We initialize an array named marks
with integer values (97, 100, 69, 78, 40, 50, 99
). We use the mkString
method on the marks
array, and this method is used to convert the elements of an array into a single string, separated by the specified delimiter.
Inside the mkString
method, we specify ", "
as the delimiter. This means that each element of the marks
array will be separated by a comma and a space in the resulting string.
The print
function is then used to print the resulting string to the console.
Output:
97, 100, 69, 78, 40, 50, 99
The code converts the array elements into a single string, and inside mkString()
, we passed ","
to print comma-delimited output.
Use a while
Loop to Print Array in Scala
Another option is to use a while
loop to iterate through array elements and print them.
In the following code, we define an array and initialize a variable i
to 0
for indexing. Use the while
loop to iterate through the array and print the element at index i
.
Example Code:
val arr = Array(1, 2, 3, 4, 5)
var i = 0
while (i < arr.length) {
println(arr(i))
i += 1
}
In the code above, we create an immutable array named arr
containing integer values (1, 2, 3, 4, 5
). Then, initialize a mutable variable 'i'
to 0
, and 'i'
will be used to keep track of the current index we are accessing in the array.
Next, the while
loop will continue to execute as long as 'i'
is less than the length of the arr
array. This ensures that we don’t go beyond the bounds of the array.
Inside the while
loop, the println(arr(i))
prints the element at the current index 'i'
of the arr
array. This line displays the element to the console.
We increment 'i'
using i += 1
to move to the next index in the array. This step ensures that we process all elements in the array one by one.
Output:
1
2
3
4
5
The output will display the arr
array elements, one per line.
Converting Array to Another Scala Collection
Though it’s not a commonly used solution, we can convert an array to another Scala collection like List
and then print it directly. We will use the toList
method to convert an array into a List.
In the following code, we create an array named marks
containing integer values. Then convert the array to a list and store it in the variable l
.
Example Code:
object MyClass {
def main(args: Array[String]): Unit = {
var marks = Array(97, 100, 69, 78, 40, 50, 99)
val l = marks.toList
print(l)
}
}
First, we initialize a mutable array named marks
with integer values (97, 100, 69, 78, 40, 50, 99
).
Then, we use the toList
method on the marks
array to convert it into an immutable list named 'l'
. Lists in Scala are immutable by default, meaning their elements cannot be modified after creation.
Finally, we use the print(l)
function to print the 'l'
list to the console.
Output:
List(97, 100, 69, 78, 40, 50, 99)
The output prints the contents of the 'l'
list, which contains the same elements as the marks
array but in list format.
Use the ScalaRunTime
Object to Print Array in Scala
Another solution is to use the ScalaRunTime
object. The ScalaRunTime
object provides a convenient way to get a string representation of an array in Scala, which can be useful for debugging and displaying array contents.
In the example below, we define an array of integers. Use the ScalaRunTime
object to generate a string representation of the array.
Example Code:
object MyClass {
def main(args: Array[String]): Unit = {
var marks = Array(97, 100, 69, 78, 40, 50, 99)
val arrayString = runtime.ScalaRunTime.replStringOf(marks, marks.length)
print(arrayString)
}
}
First, we initialize a mutable array named marks
with integer values (97, 100, 69, 78, 40, 50, 99
). Then, we use the replStringOf()
method from the runtime.ScalaRunTime
package to convert the marks
array into a string.
The replStringOf()
method is used to create a string representation of an array. The second argument, marks.length
, specifies the length of the array.
The resulting string representation of the marks
array is stored in the arrayString
variable. Finally, we use the print(arrayString)
function to print the arrayString
to the console.
Output:
Array(97, 100, 69, 78, 40, 50, 99)
The output will print the generated string representation of the array.
Conclusion
Scala offers several methods for printing an array of elements, including for
loops, foreach
loops, mkString()
method, while
loops, and using the ScalaRunTime
object. Select the method that works best for you to get Scala printing arrays.