How to Find Array Size of an Element in Scala
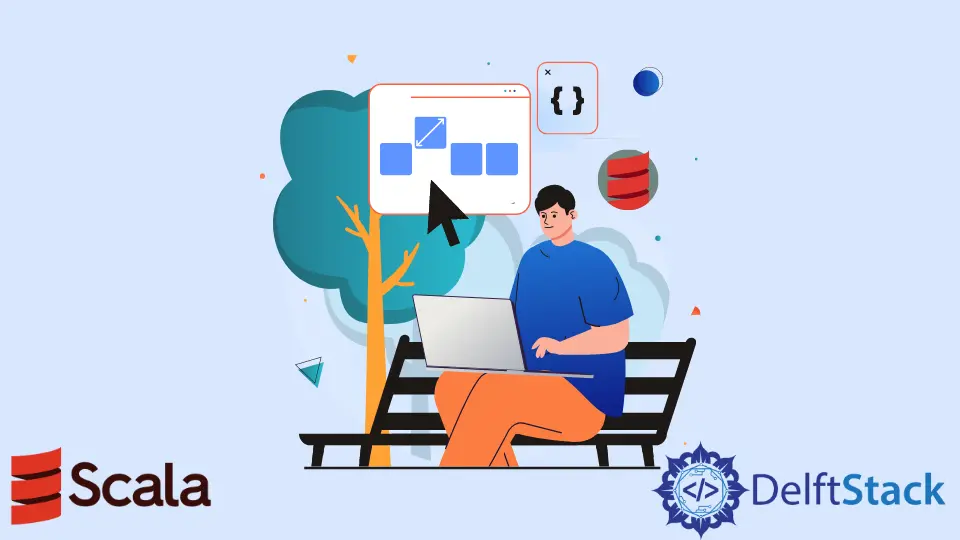
This article will explain finding an element’s size in an array. First, let’s understand how to get the size of the array and then extend that concept to get the size of elements present inside the array.
length
and size
Operator in Scala
length
operator
Syntax:
array_name.length
We can use Scala’s length
operator to calculate the array’s length in Scala. It returns the number of elements in an array.
Let’s have an example to understand it better.
Example code:
object MyClass {
def main(args: Array[String]) {
val myArr = Array("a", "b", Array(1,2,3,4,5,6), "c")
println(myArr.length)
}
}
Output:
4
We have declared an array myArr
, which contains 4 elements and one element is an array. Then we have used the length
operator to get the array’s length.
size
operator
We can use the size
property of the array to get its size. It returns the number of elements the array contains.
It is quite similar to the length
property of an array.
Syntax:
array_name.size
Let’s see an example to understand it better.
Example code:
object MyClass {
def main(args: Array[String]) {
val myArr1 = Array(3, 2, 5, 7)
val fruits = Array("Apple", "Orange",Array("watermelon","tomato"))
val rate : Array[Double] = Array(4.6, 7.8, 9.5, 4.5)
println(myArr1.size)
println(fruits.size)
println(rate.size)
}
}
Output:
4
3
4
In the above code, we have declared three arrays with some elements, and we have used the size
operator to get their respective sizes.
Get Size of the Element Inside an Array in Scala
When we create an array of different elements, it is created with java.io.Serializable
.
Example:
myArr = Array("a", "b" , Array(11,22,33), "c")
Now, if we refer to the 2nd element of myArr
, Array(11,22,33)
, its reference will be Serializable
, and there is no length
or size
for it. And if we try to use them, we will get the error.
Example code:
object MyClass {
def main(args: Array[String]) {
val myArr = Array("a", "b", Array(11,22,33), "c")
println(myArr(2).size)
}
}
Output: We can see that we get the error.
error: value size is not a member of java.io.Serializable
println(myArr(2).size)
To fix this, we have to explicitly typecast the second element of the array using asInstanceOf
.
Example code one:
object MyClass {
def main(args: Array[String]) {
val myArr = Array("a", "b", Array(11,22,33), "c")
if (myArr(2).isInstanceOf[Array[Int]])
println(myArr(2).asInstanceOf[Array[Int]].size)
}
}
Output: We can see that we get the size of the second element of the array myArr
.
3
In the above code, using the if
condition, first, we check the type and the instance of the 2nd element of the array, and then we use asInstanceOf
to typecast and then use the size
operator to get its size.
Example code two:
object MyClass {
def main(args: Array[String]) {
val myArr = Array("a", List("tony","stark","hulk","iron man"), "c")
if (myArr(1).isInstanceOf[List[String]])
println(myArr(1).asInstanceOf[List[String]].size)
}
}
Output:
4