How to Append Elements to an Array in Scala
-
Use the
concat()
Method to Append Elements to an Array in Scala -
Use the
++
Method to Append Elements to an Array in Scala -
Use the
:+
Method to Append Elements to an Array in Scala
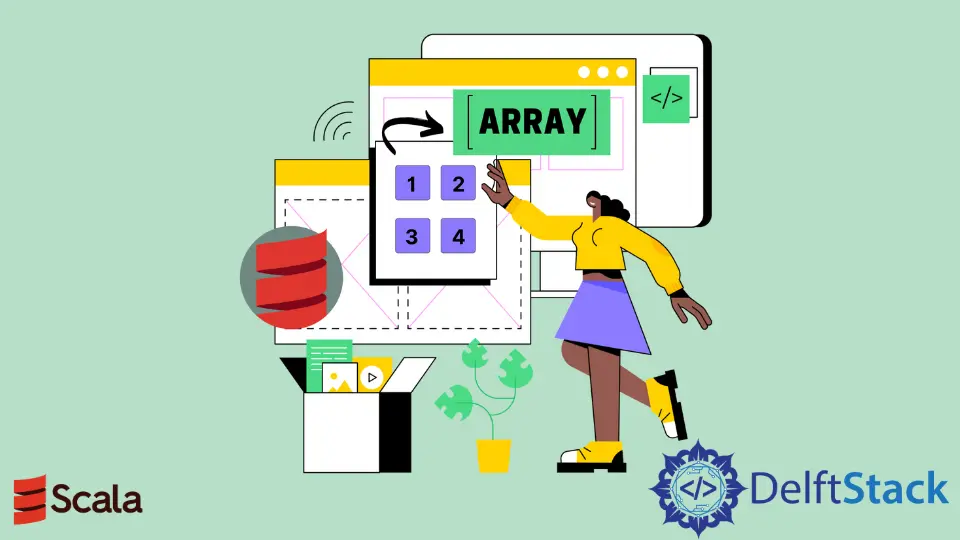
In this post, we’ll look at append elements to an Array in Scala using various methods.
Use the concat()
Method to Append Elements to an Array in Scala
Use the concat()
function to combine two or more arrays. This approach creates a new array rather than altering the current ones.
Syntax of concat()
:
newArray = concat(array1,array2,array3.....)
In the concat()
method, we can pass more than one array as arguments.
Example:
import Array._
object MyClass {
def main(args: Array[String]):Unit= {
var arr1 = Array(1, 2, 3, 4)
//create an array for the elements we want to append
var arr2 = Array(50, 60, 70, 80)
var arr3 = concat(arr1,arr2)
for(elem <- arr3)
println(elem)
}
}
Output:
1
2
3
4
50
60
70
80
Arrays arr1
and arr2
are concatenated, and a new concatenated array arr3
is created. We can see that arr3
is nothing but arr2
elements appended
at the end of arr1
.
Use the ++
Method to Append Elements to an Array in Scala
Instead of concat()
, we can also use ++
to create an appended array.
Example code:
import Array._
object MyClass {
def main(args: Array[String]):Unit= {
var arr1 = Array(1, 2, 3, 4)
var arr3 = arr1 ++ Array(100,200) //elements we want to append
for(elem <- arr3)
println(elem)
}
}
Output:
1
2
3
4
100
200
We create an array for the elements we want to append and use ++
.
Use the :+
Method to Append Elements to an Array in Scala
The :+
is the best method to append elements to an array as this method is in-place
. concat()
Method and ++
Method have to altogether create a new auxiliary array if we want to append elements to an array.
Example code:
import Array._
object MyClass {
def main(args: Array[String]):Unit= {
var array = Array( 1, 2, 3 )
array = array :+ 4
array = array :+ 5
for (x <- array )
{
println( x )
}
}
}
Output:
1
2
3
4
5
Using :+
, we can append the elements to the existing array without using an auxiliary
array.
Instead of :+
, we can also use +:
to prepend
, which means adding elements at the beginning of an array.
Example code:
import Array._
object MyClass {
def main(args: Array[String]):Unit= {
var array = Array( 1, 2, 3 )
array = 40 +: array //prepending the element
array = 55 +: array
for (x <- array )
{
println( x )
}
}
}
Output:
55
40
1
2
3