How to Append Elements to List in Scala
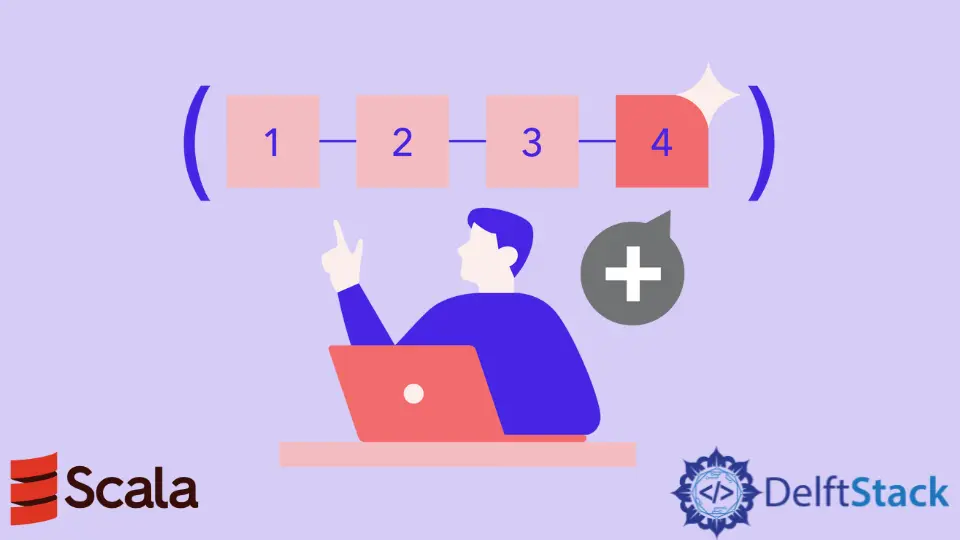
In this article, we’ll learn how to work with Scala’s list and see different ways to append elements to the list.
A list in Scala is a collection used to store sequential and similar kinds of data. They are used to represent linked lists in Scala.
Just like Strings in Java, lists are immutable in Scala. Immutable means that once a list is declared with some elements, it can’t be modified or altered.
Declaring and Initializing List in Scala
Syntax:
val listName: List[list type] = List()
Example Code:
object Main {
def main(args:Array[String])
{
//Immutable Integer type list
val mylist1: List[Int] = List(1,2,3)
//Immutable String type list
val mylist2: List[String] = List("IronMan","Hulk","BatMan")
println("Integer List :")
println(mylist1)
println("String List :")
println(mylist2)
}
}
Output:
Integer List :
List(1, 2, 3)
String List :
List(IronMan, Hulk, BatMan)
Appending Elements at the End of the List in Scala
Since Scala lists are immutable, we create a new list from the existing list with new elements added to it.
Method 1
Using the :+
method to append an element to the end of the list in Scala.
Syntax:
list_name:+new_element
Example code:
object Main {
def main(args:Array[String])
{
//Immutable String type list
val mylist1: List[String] = List("Tony","Stark","is")
println("Present List :")
println(mylist1)
// new list created using the existing list plus the new element
val newlist = mylist1 :+ "Iron Man"
println("New List :")
println(newlist)
}
}
Output:
Present List :
List(Tony, Stark, is)
New List :
List(Tony, Stark, is, Iron Man)
The same method could be used to prepend, i.e., append the values at the beginning of the list.
Example Code:
object Main {
def main(args:Array[String])
{
//Immutable String type list
val mylist1: List[String] = List("Tony","Stark")
println("Present List :")
println(mylist1)
val newlist: List[String] = "Hero" +: mylist1
println("New List :")
println(newlist)
}
}
Output:
Present List :
List(Tony, Stark)
New List :
List(Hero, Tony, Stark)
Method 2:
Using :::
in Scala to concatenate two lists. This method is beneficial when trying to append multiple values to a list.
Syntax:
list1 ::: list2
Example Code:
object Main {
def main(args:Array[String])
{
val mylist1: List[Int] = List(1,2,3,4)
println("List 1:")
println(mylist1)
val mylist2: List[Int] = List(11,22,33,55)
println("List 2:")
println(mylist2)
//appending list2 to list1
val mylist3: List[Int] = mylist1:::mylist2
println("Appended list:")
println(mylist3)
}
}
Output:
List 1:
List(1, 2, 3, 4)
List 2:
List(11, 22, 33, 55)
Appended list:
List(1, 2, 3, 4, 11, 22, 33, 55)
Method 3:
The idea here is to use mutable data structures such as ListBuffer
. Here we have +=
and append
methods to append elements to the list.
Example Code:
import scala.collection.mutable.ListBuffer
object Main {
def main(args:Array[String])
{
var myList1 = new ListBuffer[String]()
//appending values to the list
myList1+="Welcome"
myList1+="To"
myList1+=("Scala","Programming") //multiple values
myList1.append("it","is","very","easy")
println("Modified list:")
println(myList1)
}
}
Output:
Modified list:
ListBuffer(Welcome, To, Scala, Programming, it, is, very, easy)