How to Match a String Against String Literals
-
Method 1: Using the
==
Operator -
Method 2: Using the
in
Operator - Method 3: Using String Methods
- Method 4: Using Regular Expressions
- Conclusion
- FAQ
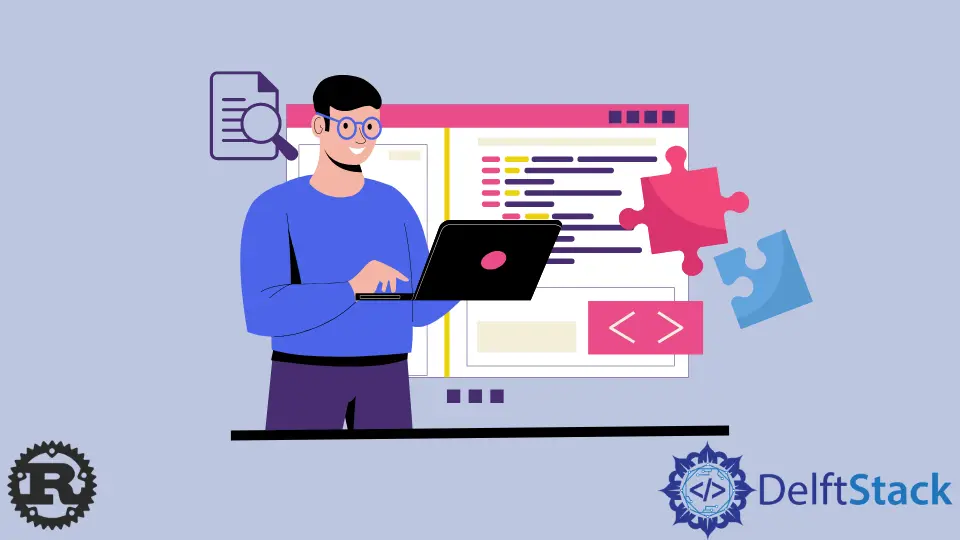
When working with strings in programming, one common task is matching a string against predefined string literals. This process is essential in various applications, from validating user input to implementing search functionalities.
In this tutorial, we will explore how to effectively match strings against string literals using Python. Whether you’re a beginner or an experienced developer, this guide will provide you with practical insights and examples to enhance your string manipulation skills. By the end of this article, you will have a solid understanding of different methods for string matching, allowing you to implement these techniques in your projects seamlessly.
Method 1: Using the ==
Operator
The simplest way to match a string against a string literal in Python is by using the equality operator (==
). This method is straightforward and effective for exact matches. When you use this operator, Python checks if the two strings are identical, returning True
if they are and False
otherwise.
Here’s a basic example:
input_string = "Hello, World!"
literal_string = "Hello, World!"
match = input_string == literal_string
print(match)
Output:
True
In this example, we define an input_string
and a literal_string
. By using the ==
operator, we check if both strings are equal. Since they are identical, the output is True
. This method is particularly useful for scenarios where you need to verify user input against expected values, such as passwords or commands.
Method 2: Using the in
Operator
Another effective way to match a string against string literals is by utilizing the in
operator. This operator checks if a substring exists within a larger string, making it ideal for scenarios where you want to determine if a specific keyword or phrase is part of a string.
Consider the following example:
input_string = "Welcome to the world of programming"
literal_string = "world"
match = literal_string in input_string
print(match)
Output:
True
In this case, we check if the literal_string
“world” is present within the input_string
. The output is True
, indicating that the substring exists. This method is particularly useful for search functionalities, where you need to find specific terms or phrases within a larger body of text. It can also be applied in filtering operations, where you want to include only certain entries based on keyword matches.
Method 3: Using String Methods
Python offers a variety of built-in string methods that can be used for matching strings against literals. One of the most commonly used methods is str.startswith()
and str.endswith()
. These methods allow you to check if a string starts or ends with a specified literal, providing additional flexibility in string matching.
Here’s an example using str.startswith()
:
input_string = "Hello, World!"
literal_string = "Hello"
match = input_string.startswith(literal_string)
print(match)
Output:
True
In this example, we check if input_string
starts with the literal_string
. The output is True
, confirming that the input begins with “Hello”. Similarly, you can use str.endswith()
to check if a string ends with a specific literal. This approach is particularly useful when validating formatted strings, such as file paths or URLs, ensuring they conform to expected patterns.
Method 4: Using Regular Expressions
For more complex string matching scenarios, Python’s re
module allows you to use regular expressions. This powerful tool enables you to define patterns for string matching, making it possible to match strings against a wide variety of formats and conditions.
Here’s an example using regular expressions:
import re
input_string = "My email is example@mail.com"
pattern = r"[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}"
match = re.search(pattern, input_string)
print(bool(match))
Output:
True
In this case, we use the re.search()
function to look for an email pattern within the input_string
. The regular expression defined in pattern
matches typical email formats. The output is True
, indicating a match was found. Regular expressions are invaluable for tasks like validating input formats, parsing data, or extracting specific information from strings. Although they can be complex, mastering them greatly enhances your string manipulation capabilities in Python.
Conclusion
Matching strings against string literals is a fundamental skill in programming, especially in Python. Whether you choose to use simple equality checks, substring searches, string methods, or regular expressions, understanding these techniques can significantly improve your coding efficiency and accuracy. Each method has its own strengths, making it essential to select the right one for your specific use case. With the examples and explanations provided in this tutorial, you should now feel confident in implementing string matching techniques in your projects. Keep practicing, and you’ll soon find that string manipulation becomes second nature.
FAQ
-
What is the simplest way to match strings in Python?
The simplest way is to use the equality operator (==
) to check if two strings are identical. -
How can I check if a substring exists within a string?
You can use thein
operator to determine if a substring is present in a larger string. -
What are
str.startswith()
andstr.endswith()
used for?
These methods check if a string starts or ends with a specified literal, respectively. -
When should I use regular expressions for string matching?
Regular expressions are best for complex matching scenarios where patterns are needed, such as validating formats. -
Can I use string methods for case-insensitive matching?
Yes, you can convert both strings to the same case usingstr.lower()
orstr.upper()
before comparison.