How to Create String Enum in Rust
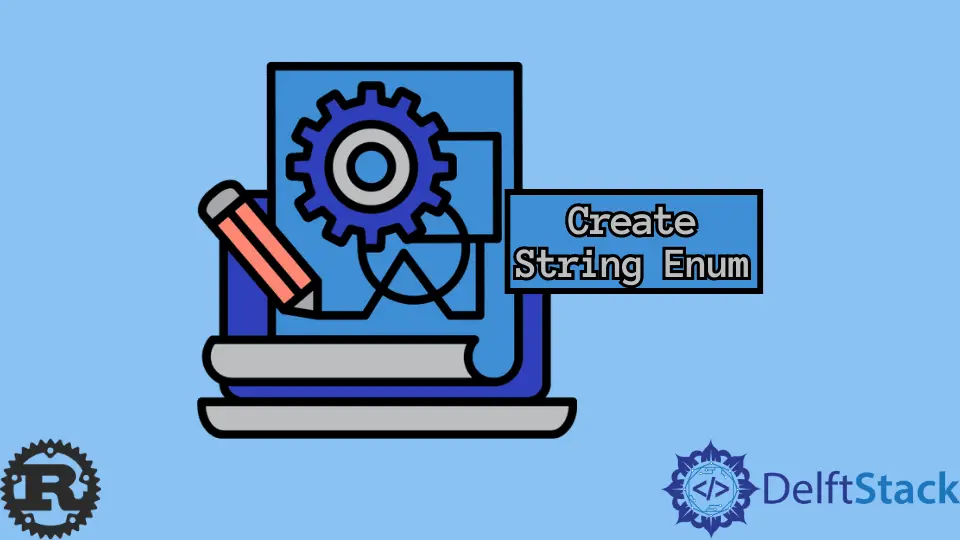
In this article, we will learn about creating string enum in Rust.
Create enums
in Rust
In Rust, an enum
represents data that is one of the multiple possible versions. The enum
keyword permits the development of a type that may be one of several possible versions.
Any version valid as a struct
is also valid as an enum
. In addition, each version in the enum
may have optionally related data:
enum Information {
Quit,
ChangeColor(i32, i32, i32),
Move { x: i32, y: i32 },
Write(String),
}
There are variations with no data, variants with named data, and variants with anonymous data. The syntax for defining variants mimics that of defining structs like tuple structs
.
In contrast to individual struct definitions, an enum
is a single type. An enum
value may match any of the variants.
This is why an enum
is frequently referred to as a sum type: the set of potential enum
values is the sum of the sets of possible variant values.
We use the ::
syntax to refer to each variant’s name, which is scoped by the name of the enum
itself.
Strum
in Rust
Strum
is a collection of macros
and characteristics that make working with enums
and strings in Rust
easier.
EnumString
: auto-derives std::str::FromStr
is applied to the enum
. Each enum
version will match its name.
This can be overridden by using serialize="DifferentName"
or to string="DifferentName"
on the attribute, as demonstrated below. It is possible to add many deserializations to the same variation.
If the variation contains additional data, deserialization will set them to their default values. The default
attribute can be applied to a tuple variation with a single data parameter.
The specified variant is returned if no match is discovered, and the input string is captured as a parameter. Here is an example of code created by EnumString
inheritance.
#[derive(EnumString)]
enum Cars {
BMW,
Volvo { range:usize },
#[strum(serialize="Toyota",serialize="b")]
Toyota(usize),
#[strum(disabled="true")]
Fiat,
}
Note that the default implementation of FromStr
only matches the variant’s name. Both Display
and ToString
return the specified enum
variation. This enables you to convert unit-style variants from enum
to string and back again.
In addition, ToString
and Display
select the appropriate serialization depending on the following criteria.
- This value will be utilized if a string property exists. Only one is allowed per version.
- The serialized property with the longest value is selected. If this behavior is not desired, use to string instead.
- Finally, the variant’s name will be utilized if neither
serialize
norto string
properties exist.
Display
is preferable over ToString
All types implementing ::std::fmt::Display
have a default implementation of ToString
.
use std::string::ToString;
#[derive(Display, Debug)]
enum Cars {
#[strum(serialize="redred")]
BMW,
Volvo { range:usize },
Toyota(usize),
Ferrari,
}
fn debug_cars() {
let BMW = Cars::BMW;
assert_eq!(String::from("BMWBMW"), BMW.to_string());
}
fn main () { debug_cars(); }