How to Convert String to STR in Rust
- Concept of String in Rust
-
Concept of
str
in Rust -
Conversion of String to
str
in Rust -
Difference Between
str
and String in Rust
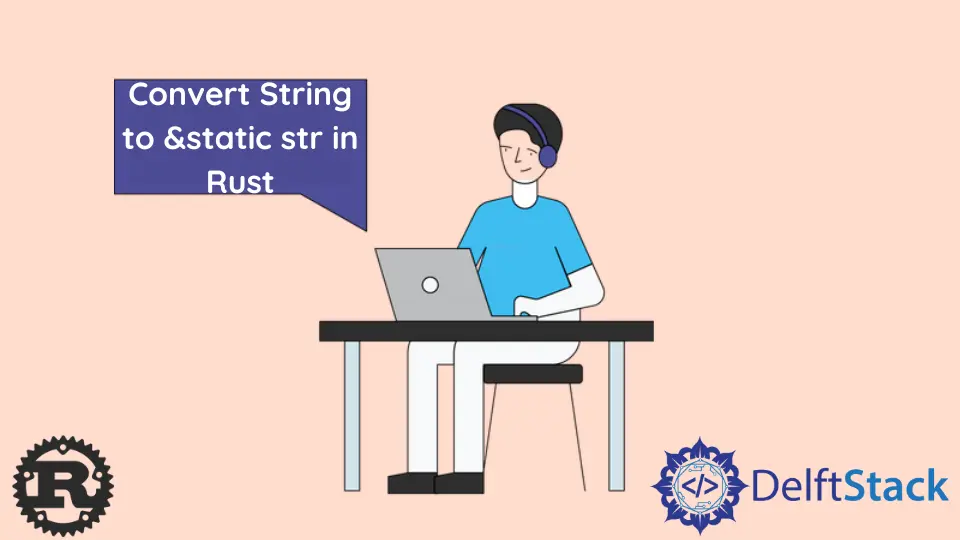
Rust is a language that was designed to be robust and safe. It also focuses on performance for anything from systems programming to scripting.
The String type in Rust is not immutable, but the String type does have methods for creating and manipulating strings. This article will discuss the String
and &static str
in detail.
Concept of String in Rust
A String is a struct containing a vector Vec
, an 8-bit unsigned array that can expand.
A String, unlike str
, holds ownership of the data. Hence it’s not essential to utilize & or borrow state when assigning a String’s value to a variable.
During initiation, the size of a String can be known or unknown at compile-time, but it can expand until its length hits its limit.
Syntax:
let my_string = String::from("Hello World");
Concept of str
in Rust
In Rust, str
is a primitive type that defines a string literal. Its information is assigned in the program binary’s memory location.
String Slice
A slice is a view that contains a sequence of items and is denoted by the syntax. Slices do not have ownership, but they allow you to refer to the order in which items appear.
As a result, a string slice is a reference to a string’s sequence of elements.
let hello_string = String::from("hello world");
let hello_slice = &hello_string[4.8];
The letters "hello world"
are stored in the hello_string
variable. The String, a growable array, contains a location or index for each character.
String Literals
A string literal is constructed by enclosing text in double quotes. String literals are a little different.
They are string slices that refer to "pre-allocated text"
stored as part of the executable in read-only memory. In other words, RAM comes with our software and doesn’t rely on stack caches.
Conversion of String to str
in Rust
Strings may not exist for the whole life of your code, which is what &'static str
lifetime signifies, therefore, you can’t get &'static str
from them. Only a slice specified by String’s lifespan may be obtained from it.
Example Code:
fn main() {
let hello_string = String::from("Hello world");
print_infi(&hello_string);
print!("Adil {}", hello_string);
}
fn print_infi(infi: &str) {
println!("Steve {} ", infi);
}
Output:
Steve Hello world
Adil Hello world
Difference Between str
and String in Rust
String |
Str |
|
---|---|---|
1. | Mutable | Immutable |
2. | At compilation time, the size is unknown. | At compilation time, the size is known. |
3. | Data is stored in a heap. | Data is stored in the application binary’s memory location. |
4. | To allocate a single str variable, employ or reference. |
To allocate a String variable value, you don’t need to employ or reference. |
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook