How to Concatenate Strings in Rust
-
Using the
+
Operator -
Using the
format!
Macro -
Using the
push_str
Method -
Using the
push
Method - Conclusion
- FAQ
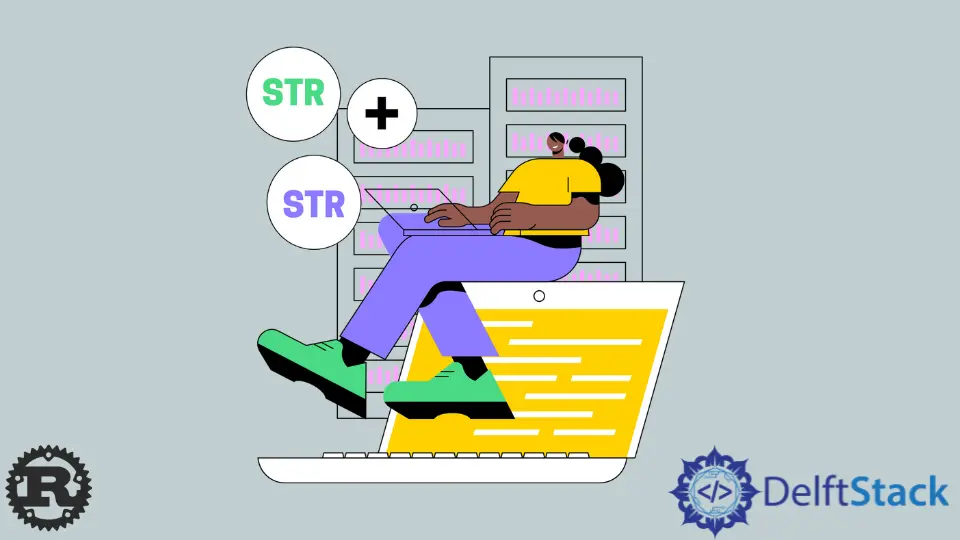
Concatenating strings in Rust is a fundamental skill that every Rustacean should master. Whether you’re working on a small project or a large application, string manipulation is often a necessary task.
In this tutorial, we’ll explore various methods to concatenate strings in Rust, ensuring you have a solid understanding of the best practices involved. Rust’s ownership model and memory safety features can make string handling a bit different from other programming languages, so it’s crucial to grasp these concepts. By the end of this article, you’ll be equipped with the knowledge to effectively concatenate strings in your Rust programs. Let’s dive in!
Using the +
Operator
One of the simplest ways to concatenate strings in Rust is by using the +
operator. This method allows you to combine two strings easily. However, it’s important to note that this operator consumes the first string and requires the second string to be a string slice (&str
). Here’s how you can do it:
fn main() {
let str1 = String::from("Hello, ");
let str2 = "world!";
let result = str1 + str2;
println!("{}", result);
}
Output:
Hello, world!
In this example, we create a String
called str1
and a string slice called str2
. By using the +
operator, we concatenate str1
and str2
into a new string, result
. The first string (str1
) is moved and can no longer be used after concatenation, while str2
remains intact since it was borrowed. This method is straightforward and efficient for simple concatenations.
Using the format!
Macro
Another powerful way to concatenate strings in Rust is by using the format!
macro. This method is more flexible than the +
operator, as it doesn’t consume the original strings. Instead, it creates a new String
without altering the existing ones. Here’s an example:
fn main() {
let str1 = String::from("Hello, ");
let str2 = String::from("world!");
let result = format!("{}{}", str1, str2);
println!("{}", result);
}
Output:
Hello, world!
In this snippet, we use the format!
macro to concatenate str1
and str2
. The syntax is similar to println!
, where we use curly braces {}
as placeholders for the variables. This method is particularly useful when you need to concatenate multiple strings or include other data types in the output. Since str1
and str2
are not consumed, you can still use them after the concatenation.
Using the push_str
Method
If you want to append a string slice to an existing String
, the push_str
method is an excellent option. This method modifies the original String
in place, making it efficient for scenarios where you want to build a string incrementally. Here’s how it works:
fn main() {
let mut str1 = String::from("Hello, ");
let str2 = "world!";
str1.push_str(str2);
println!("{}", str1);
}
Output:
Hello, world!
In this example, we create a mutable String
called str1
and a string slice str2
. By calling str1.push_str(str2)
, we append str2
to str1
. The result is that str1
now contains the concatenated string. This method is particularly useful when you are building strings in a loop or when you need to concatenate multiple pieces of data over time.
Using the push
Method
For situations where you want to add a single character to a String
, the push
method is the way to go. This method appends a character to the end of the String
, allowing for precise control over string content. Here’s an example:
fn main() {
let mut str1 = String::from("Hello");
str1.push(',');
str1.push(' ');
str1.push_str("world!");
println!("{}", str1);
}
Output:
Hello, world!
In this code, we first create a mutable String
called str1
. We then use the push
method to add a comma and a space before concatenating the string slice “world!” using push_str
. This method is particularly handy when you need to build strings character by character or when you want to insert specific characters at various points in the string.
Conclusion
In this tutorial, we explored several methods for concatenating strings in Rust, including the +
operator, the format!
macro, and the push_str
and push
methods. Each of these techniques has its advantages and is suited for different scenarios. Understanding these methods will enhance your string manipulation skills in Rust and enable you to write cleaner, more efficient code. As you practice, you’ll find the best method for your specific needs, making string concatenation a breeze in your Rust projects.
FAQ
-
What is the simplest way to concatenate strings in Rust?
The simplest way is to use the+
operator, which allows you to combine two strings easily. -
Can I concatenate strings without consuming the original ones?
Yes, you can use theformat!
macro, which creates a new string without altering the original ones. -
How do I append a string slice to an existing string?
You can use thepush_str
method to append a string slice to a mutableString
. -
Is there a method to add a single character to a string?
Yes, you can use thepush
method to append a single character directly to aString
. -
What happens to the original string when I use the
+
operator?
The original string on the left side of the+
operator is consumed and can no longer be used after concatenation.