How to Write to a File in Ruby
-
Method 1: Using the
File.open
Method -
Method 2: Using the
File.write
Method -
Method 3: Using the
IO#puts
Method - Conclusion
- FAQ
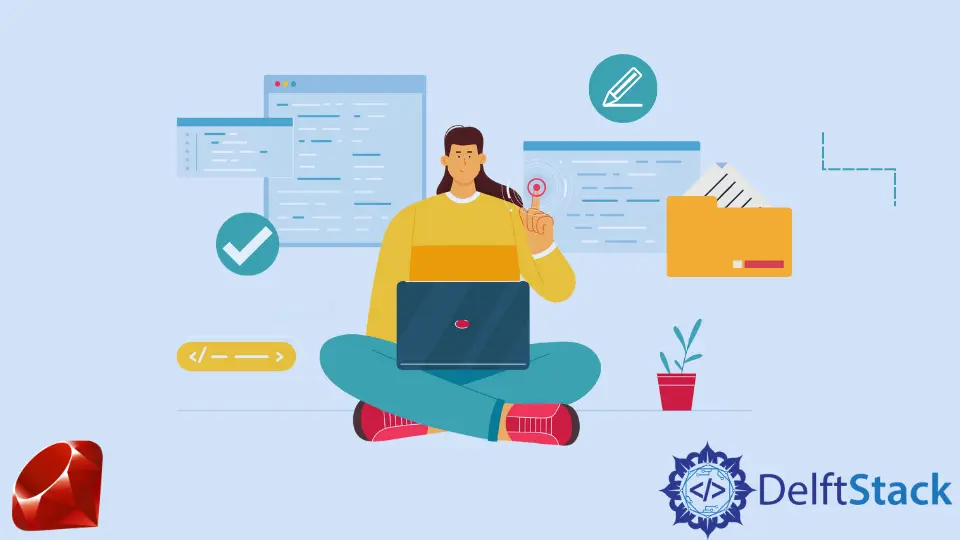
Writing to a file is a fundamental task in programming, and Ruby makes it easy to handle file operations. Whether you are logging data, saving user inputs, or generating reports, knowing how to write to a file in Ruby is essential for any developer.
In this article, we’ll explore various methods to write to a file in Ruby, complete with clear examples and explanations. By the end of this guide, you’ll have a solid understanding of how to effectively write to files, enhancing your programming toolkit. So, let’s dive in!
Method 1: Using the File.open
Method
One of the most straightforward ways to write to a file in Ruby is by using the File.open
method. This method allows you to specify the mode in which you want to open the file, giving you flexibility in how you handle file operations.
Here’s a simple example:
File.open("example.txt", "w") do |file|
file.puts "Hello, world!"
file.puts "This is my first file write in Ruby."
end
Output:
Hello, world!
This is my first file write in Ruby.
In this code, we open a file named example.txt
in write mode ("w"
). If the file doesn’t exist, Ruby will create it. Inside the block, we use the puts
method to write two lines of text to the file. The block automatically closes the file when it’s finished, ensuring that resources are properly released.
Using File.open
is beneficial because it allows for clean and efficient file handling. It’s particularly useful when you need to write multiple lines or handle files within a specific context. This method also supports various modes like "a"
for appending, which can be handy for logging or updating existing files without overwriting their content.
Method 2: Using the File.write
Method
Another convenient method to write to a file in Ruby is the File.write
method. This method is particularly useful when you want to write a string directly to a file without the need for a block.
Here’s how you can do it:
File.write("example.txt", "This will overwrite the existing content.")
Output:
This will overwrite the existing content.
In this example, we use File.write
to write a single string to example.txt
. If the file already contains data, this method will overwrite it entirely. It’s a quick and efficient way to write data, especially when you don’t need to manage file handling manually.
The File.write
method is ideal for scenarios where you want to replace the entire content of a file with new data. It’s straightforward and requires minimal code, making it a favorite among Ruby developers for quick file writing tasks.
Method 3: Using the IO#puts
Method
The IO#puts
method is another effective way to write to a file in Ruby. This method is similar to File.open
but can be used in a more general context. You can create a new file or append to an existing one using this approach.
Here’s an example:
file = File.new("example.txt", "a")
file.puts "Appending a new line to the file."
file.close
Output:
Appending a new line to the file.
In this code, we create a new file object using File.new
, specifying the append mode ("a"
). This allows us to add content to the end of the file without losing any existing data. After writing the new line using puts
, we close the file to ensure that all changes are saved.
Using the IO#puts
method is particularly useful for logging applications or when you need to keep a record of multiple entries over time. It allows for incremental updates to a file, making it a great choice for applications that require ongoing data storage.
Conclusion
Writing to a file in Ruby is a straightforward process, thanks to its intuitive methods like File.open
, File.write
, and IO#puts
. Each method serves a unique purpose, whether you need to overwrite existing content, append new lines, or manage file operations within a block. By mastering these techniques, you can enhance your Ruby programming skills and effectively handle file operations in your applications. With this knowledge, you can confidently work with files in Ruby, opening up new possibilities for your projects.
FAQ
-
What file modes can I use in Ruby?
You can use modes like “r” for reading, “w” for writing (overwriting), “a” for appending, and “r+” for reading and writing. -
How do I check if a file exists before writing?
You can useFile.exist?("filename.txt")
to check if a file exists before performing any write operations. -
Can I write binary data to a file in Ruby?
Yes, you can write binary data by opening the file in binary mode using “wb” or “ab”.
-
How do I handle errors when writing to a file?
You can usebegin...rescue
blocks to handle exceptions while performing file operations, ensuring your program can manage errors gracefully. -
Is there a way to write to a file without overwriting existing data?
Yes, using the append mode (“a”) allows you to add new content to the end of the file without removing existing data.
#puts. Learn effective techniques to handle file operations, including appending and overwriting data. Enhance your Ruby programming skills with clear examples and insights.