How to List File in Folder in Ruby
- Create a Sample Folder
-
Use
Dir::[]
to List All Files in a Folder in Ruby -
Use
Dir.glob
to List All Files in a Folder in Ruby -
Use
Dir.entries
to List All Files in a Folder in Ruby -
Use
Pathname
to List All Files in a Folder in Ruby - Conclusion
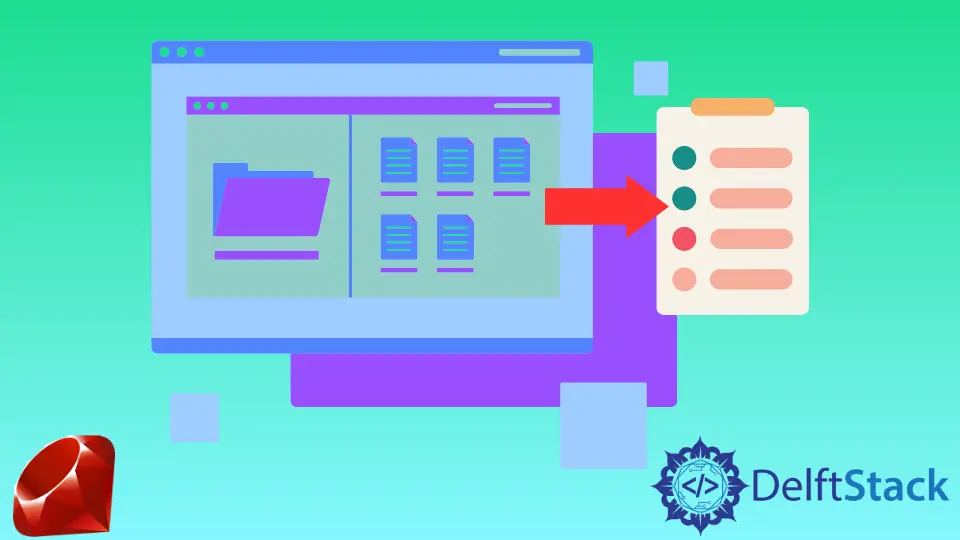
Listing all files in a specific folder can be essential for various tasks in Ruby programming. In this guide, we’ll explore different methods to accomplish this task.
We’ll create a sample folder structure for demonstration purposes and then showcase the following methods: using Dir::[]
, Dir.glob
, Dir.entries
, and Pathname
.
Create a Sample Folder
Before we dive into the code examples, let’s set up a sample folder structure in your current directory.
Command:
mkdir parent_folder
touch parent_folder/music_1.txt
touch parent_folder/music_2.txt
mkdir parent_folder/child_folder
touch parent_folder/child_folder/doc_1.txt
touch parent_folder/child_folder/doc_2.txt
We’ve created a parent_folder
with two files (music_1.txt
and music_2.txt
) and a subfolder child_folder
containing two more files (doc_1.txt
and doc_2.txt
).
Use Dir::[]
to List All Files in a Folder in Ruby
The Dir::[]
method returns an array containing all file and folder names inside the specified directory. To list only files, we can apply the File.file?
method to filter the results.
In the syntax below, the Dir['directory_path/*']
uses the Dir::[]
method to list all items (files and directories) in the specified directory ('directory_path'
) and the wildcard *
is used to match all items in the directory.
Syntax:
items = Dir['directory_path/*']
Example:
files = Dir['parent_folder/*'].select { |path| File.file?(path) }
puts files
The code starts by using the Dir
class to list all items (files and directories) in the 'parent_folder'
directory. The Dir['parent_folder/*']
expression returns an array of file and directory paths inside the 'parent_folder'
.
The .select
method is then called on the array of paths returned by Dir['parent_folder/*']
. Inside the block of .select
, each path
is checked using File.file?(path)
to determine if it’s a file (as opposed to a directory or other types of items).
Only the file paths are selected and stored in the files
variable. Finally, the code prints the contents of the files
variable using puts
, which displays a list of file paths found within the 'parent_folder'
directory.
Output:
parent_folder/music_1.txt
parent_folder/music_2.txt
The output lists the files located in the "parent_folder"
. It displays the full paths of the files, with each file on a new line.
To list files recursively inside subfolders, you can use the following pattern.
Example:
files = Dir['parent_folder/**/*'].select { |path| File.file?(path) }
puts files
Output:
parent_folder/music_1.txt
parent_folder/music_2.txt
parent_folder/child_folder/doc_2.txt
parent_folder/child_folder/doc_1.txt
The output lists all the files located in both the "parent_folder"
and its subfolder "child_folder"
, including their respective paths.
Use Dir.glob
to List All Files in a Folder in Ruby
The Dir.glob
method is equivalent to using Dir::[]
and allows us to list files in a folder. It also supports recursive listing.
In the syntax below, the Dir.glob('parent_folder/**/*')
uses the Dir.glob
method to list all items (files and directories) in the 'parent_folder'
directory and its subdirectories and the pattern 'parent_folder/**/*'
is used to match all files and subdirectories recursively.
Syntax:
files = Dir.glob('parent_folder/**/*')
Example:
files = Dir.glob('parent_folder/**/*').select { |path| File.file?(path) }
puts files
Example:
require 'pathname'
folder_path = 'parent_folder'
path = Pathname.new(folder_path)
path.find do |entry|
puts entry if entry.file?
end
In the code above, we include the 'pathname'
library to work with file paths. We define the folder path (folder_path = 'parent_folder'
) we want to explore.
Then, we create a Pathname object called path
representing the 'parent_folder'
. Next, we use the find
method to traverse the directory and its subdirectories.
Lastly, we check if entry
is a file, and if so, we print its path.
Output:
parent_folder/music_1.txt
parent_folder/music_2.txt
parent_folder/child_folder/doc_2.txt
parent_folder/child_folder/doc_1.txt
In the code, it uses the Pathname
library to list all files located in both the "parent_folder"
and its subfolder "child_folder"
, including their respective paths.
Conclusion
Listing files in a folder is a common operation in Ruby programming. Choose the method that best suits your needs and coding style.
Whether you prefer the simplicity of Dir::[]
and Dir.glob
, the flexibility of Dir.entries
, or the object-oriented nature of Pathname
, Ruby provides options to efficiently list files, making your file-related tasks more manageable.