How to Read Lines of a Files in Ruby
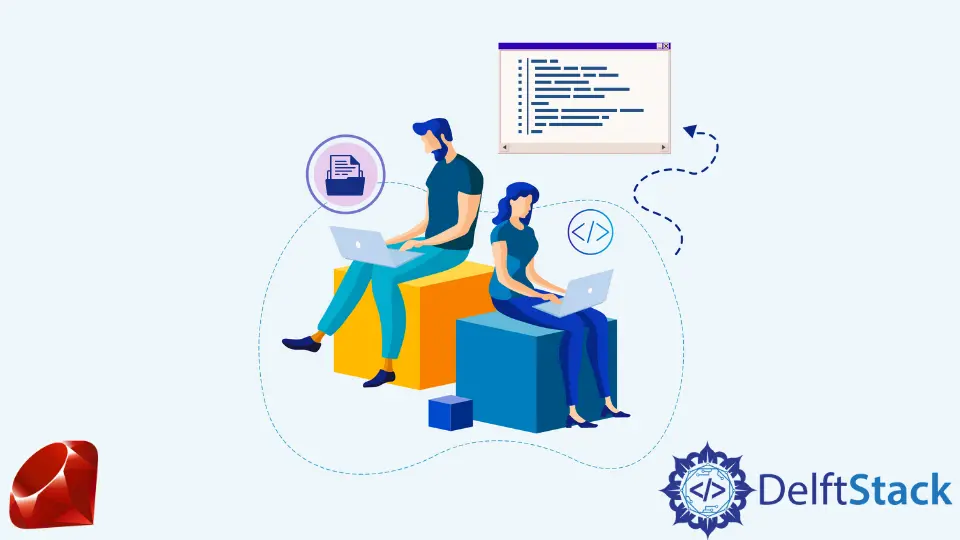
Reading lines from a file in Ruby is a fundamental skill for any developer, whether you’re working on a small script or a large application. Ruby provides several methods to accomplish this task, allowing for flexibility and efficiency.
In this article, we will explore two popular methods to read lines from a file in Ruby. We’ll provide clear code examples and explanations to help you understand how each method works. By the end of this guide, you’ll be equipped with the knowledge to handle file reading tasks with confidence and ease.
Method 1: Using File.readlines
The first method we will discuss is File.readlines
, a straightforward way to read all lines from a file into an array. This method is efficient for smaller files, as it loads the entire file content into memory at once. Here’s how you can use it:
lines = File.readlines('example.txt')
lines.each do |line|
puts line.chomp
end
In this example, we use File.readlines
to read all lines from a file named example.txt
. The method returns an array where each element corresponds to a line in the file. The each
method is then employed to iterate through each line. We use chomp
to remove the newline character at the end of each line before printing it.
Output:
This is the first line.
This is the second line.
This is the third line.
Using File.readlines
is particularly useful when you need to process all lines at once. However, keep in mind that if your file is large, this approach may consume a significant amount of memory. For smaller files or when you know the file size is manageable, this method is both simple and effective.
Method 2: Using File.foreach
The second method we will explore is File.foreach
, which is ideal for reading large files line by line without loading the entire file into memory. This method is memory-efficient and allows you to handle each line individually as you read it. Here’s how to implement it:
File.foreach('example.txt') do |line|
puts line.chomp
end
In this code snippet, we use File.foreach
to open and read the file example.txt
. The block provided to foreach
is executed for each line in the file. Just like in the previous method, we use chomp
to eliminate the trailing newline character before printing the line.
Output:
This is the first line.
This is the second line.
This is the third line.
The beauty of File.foreach
lies in its ability to handle large files efficiently. Since it reads one line at a time, it significantly reduces memory usage, making it an excellent choice for processing large datasets or log files. This method allows you to implement additional logic within the block, such as filtering or transforming data as it is read.
Conclusion
In summary, reading lines from a file in Ruby can be accomplished using various methods, with File.readlines
and File.foreach
being two of the most common. The choice between these methods depends on your specific needs—whether you prefer simplicity and ease of use for smaller files or memory efficiency for larger files. By mastering these techniques, you’ll enhance your Ruby programming skills and be better equipped to handle file operations in your projects.
FAQ
-
How do I handle errors when reading a file in Ruby?
You can use abegin-rescue
block to catch exceptions when opening or reading a file. This allows you to manage errors gracefully. -
Can I read files from a URL in Ruby?
Yes, you can use libraries likeopen-uri
to read files from a URL in Ruby, similar to reading from a local file. -
What is the difference between
File.readlines
andFile.foreach
?
File.readlines
reads the entire file into memory as an array, whileFile.foreach
reads one line at a time, making it more memory-efficient for large files. -
Is there a way to read specific lines from a file in Ruby?
Yes, you can read specific lines by usingFile.readlines
and then accessing the desired indices of the returned array. -
Can I write to a file while reading it in Ruby?
Writing to a file while reading it can lead to unpredictable results. It’s best to read and write in separate operations or use file locks.