How to Create a File in Ruby
- Method 1: Using the File Class
- Method 2: Using the FileUtils Module
- Method 3: Using the IO Class
- Method 4: Using the File.write Method
- Conclusion
- FAQ
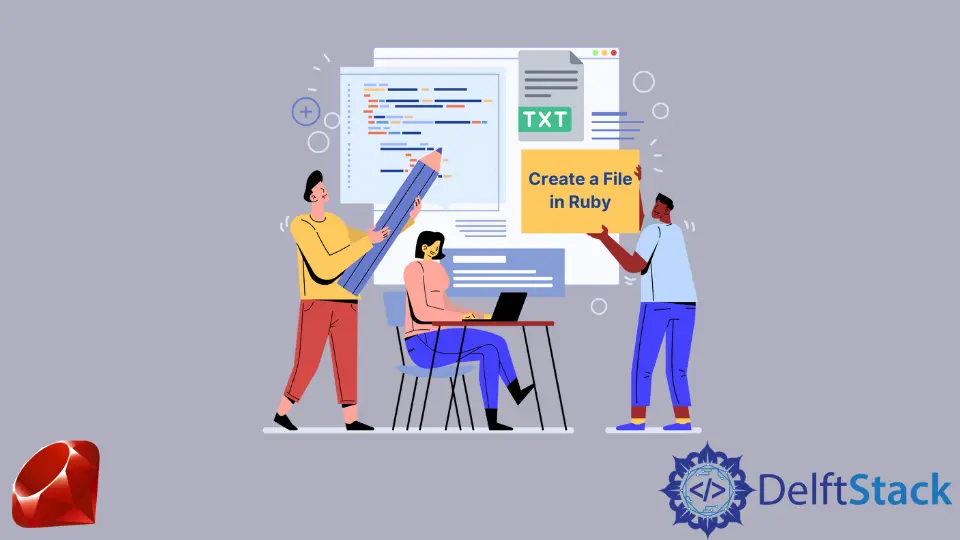
Creating files in Ruby is a fundamental skill for any developer looking to work with data storage, logging, or even just simple file manipulation. Whether you’re building a small application or a complex system, knowing how to create and manage files can significantly enhance your productivity.
In this article, we will walk you through the process of creating a file in Ruby, demonstrating various methods and providing clear code examples. By the end of this guide, you will have a solid understanding of file creation in Ruby, enabling you to implement these techniques in your own projects seamlessly.
Method 1: Using the File Class
The simplest way to create a file in Ruby is by using the built-in File
class. This method is straightforward and allows you to create a file with just a few lines of code. Here’s how you can do it:
File.open("example.txt", "w") do |file|
file.puts "Hello, this is a test file."
end
Output:
A new file named example.txt is created with the text "Hello, this is a test file."
In this code snippet, we use File.open
to create a new file called example.txt
. The "w"
mode indicates that we are opening the file for writing. If the file already exists, it will be overwritten. Inside the block, we use file.puts
to write a line of text into the file. This method is efficient because it automatically closes the file after the block is executed, ensuring that resources are properly managed.
Method 2: Using the FileUtils Module
Another way to create files in Ruby is by utilizing the FileUtils
module. This module provides a higher-level interface for file manipulation, making it easier to manage files and directories. Here’s an example of how to create a file using FileUtils
:
require 'fileutils'
FileUtils.touch("example_file.txt")
File.write("example_file.txt", "This file was created using FileUtils.")
Output:
A new file named example_file.txt is created with the text "This file was created using FileUtils."
In this example, we first require the fileutils
library, which provides us with additional methods for file handling. The FileUtils.touch
method creates an empty file named example_file.txt
. If the file already exists, it updates the file’s timestamp. After that, we use File.write
to add content to the file. This method is particularly useful when you want to create a file without immediately writing to it, giving you flexibility in file management.
Method 3: Using the IO Class
The IO
class in Ruby also allows you to create files, and it can be particularly useful when dealing with input and output operations. Here’s how you can create a file using the IO
class:
io_file = IO.new(IO.sysopen("new_file.txt", "w"))
io_file.write("This is written using the IO class.")
io_file.close
Output:
A new file named new_file.txt is created with the text "This is written using the IO class."
In this snippet, we use IO.sysopen
to open a file descriptor for new_file.txt
in write mode. We then create a new IO
object and use the write
method to add content to the file. Finally, we call close
to ensure that the file descriptor is properly released. This method provides more control over file operations, which can be beneficial in complex applications.
Method 4: Using the File.write Method
If you want a quick and straightforward way to create a file and write content to it in one go, you can use the File.write
method. This method is particularly handy for simple tasks. Here’s how to use it:
File.write("quick_file.txt", "Quickly created this file using File.write.")
Output:
A new file named quick_file.txt is created with the text "Quickly created this file using File.write."
In this example, File.write
takes two arguments: the name of the file and the content you want to write. If the file already exists, it will be overwritten. This method is efficient for creating files when you already know the content you want to include, making it a great choice for quick file operations.
Conclusion
Creating files in Ruby is an essential skill that can enhance your programming capabilities. Whether you choose to use the File
class, FileUtils
module, IO
class, or the File.write
method, each approach has its advantages. By understanding these methods, you can easily manage file creation and manipulation in your Ruby applications. Remember to choose the method that best fits your needs and coding style. Happy coding!
FAQ
- How do I check if a file exists before creating it?
You can useFile.exist?("filename.txt")
to check if a file exists before creating it.
-
Can I append data to an existing file in Ruby?
Yes, you can open a file in append mode using"a"
withFile.open
. -
What happens if I try to write to a file that is already open?
If you attempt to write to a file that is already open in write mode, it will overwrite the existing content. -
Is there a way to create a directory and a file in Ruby at the same time?
You can useDir.mkdir("new_directory")
to create a directory and then create a file inside it. -
Can I create files with different encodings in Ruby?
Yes, you can specify the encoding when creating a file by usingFile.new("filename.txt", "w:UTF-8")
.