How to Use Redirect in React Router
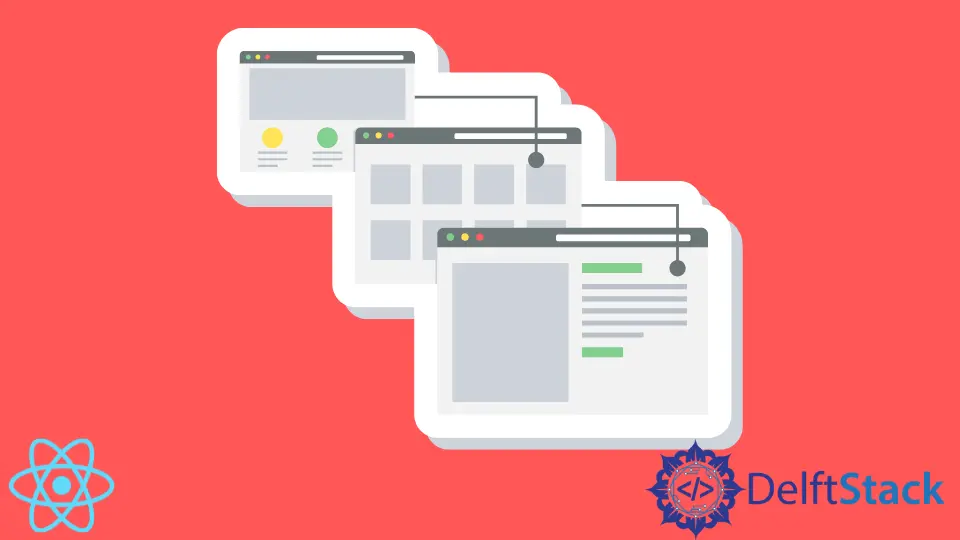
If you’re a developer working on complex web applications, you probably understand the importance of routing. Routes are one of the key pieces that make up the navigation system of the app. ReactJs developers tend to use the react-router-dom
package to create routes for applications.
After submitting a form (or any other user action) sometimes the application needs to navigate to a different URL. In this article, we’ll look at how to handle redirects in React.
React Router Redirect in Functional Components
Hooks can extend the basic functionality of functional components. React developers can use built-in hooks like useReducer()
, useState()
, useEffect()
or create their own customized versions.
useHistory()
Hook
The useHistory()
returns a history
instance, which contains the current location (URL) of the component. Handling redirects is not a primary purpose of this hook. However, new versions of React Router (5 and higher) allow you to use this hook to redirect the user in an efficient and readable way.
Let’s look at the example:
import { useHistory } from "react-router-dom"
function SampleComponent() {
let history = useHistory()
function handleClick() {
history.push("/somepage")
}
return (
<span onClick={() => handleClick()}>
Go home
</span>
)
}
In this example, we import the useHistory
hook from the react-router-dom
package. Then we define a simple functional component that returns a single <span>
element with an event handler for the click
event.
We also create a history
variable to store the instance returned by the useHistory()
hook.
In the body of the event handler function, we use a .push()
method and pass a string as an argument. The argument for this method will be added to the current URL at the end. So, if our application was running on localhost:3000
port, and we had to handle a click
event, our URL would change to localhost:3000/somepage
.
React Router Redirect in Class Components
Another way to navigate to a different URL after performing an action (submitting a form, clicking a button, or any other user action) is to use the custom <Redirect>
component. You can import it from "react-router-dom"
library.
You can control when the redirect takes place by rendering it conditionally based on state value. Let’s look at this example:
import React from "react"
import { Redirect } from "react-router-dom"
class MyComponent extends React.Component {
constructor(props){
super(props)
this.state = {
condition: false
}
}
handleClick () {
axios.post(/*URL*/)
.then(() => this.setState({ condition: true }));
}
render () {
const { condition } = this.state;
if (condition) {
return <Redirect to='/somePage'/>;
}
return <button onClick={() => this.handleClick()}>Redirect</button>;
}
Our state has a condition
property, which is set to false
. Because of this boolean value, the <Redirect>
component does not render and the user’s URL does not change.
By clicking the single button, users can trigger an event handler that sets the condition
property to true
. In this case, the criteria for the if
statement will be met, and the render function is going to return the <Redirect>
component, which will take users to the specified relative path.
In this case, if our application has a homepage at localhost:3000
, and our <Redirect>
component has a to
attribute with the value of '/somePage'
, the users will be redirected to the following location: localhost:3000/somePage
.
this.props.history.push("")
Using the useHistory()
hook is not the only way to access the user’s current location. Developers can achieve the same in both types of components by reading the history
property of the props
object.
If you want the redirect to happen after clicking a button, you must set up an onClick
event handler, which will use the .push("")
method to provide a relative path to the location where the user should be redirected. Here’s an example:
<button onClick={this.props.history.push('/somePage')}>Redirect to some page </button>
If their current location is on the localhost:3000
homepage, clicking this button will take users to localhost:3000/somePage
URL.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn