How to Create Dynamic Routes in React Router
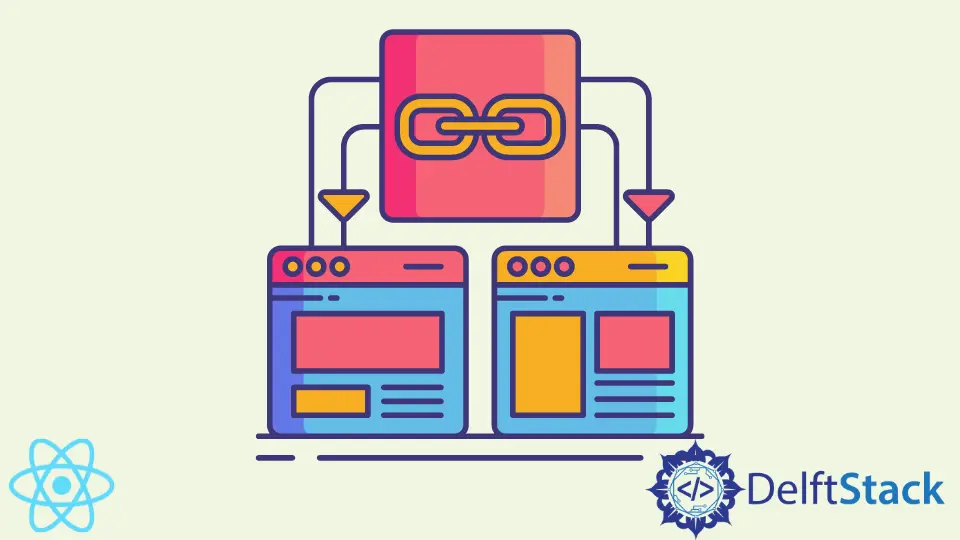
React is one of, if not the most popular library for building web applications. However, not many people know React is only a library that deals with rendering UI.
It does not have built-in solutions for routing as Angular does. For this reason, React developers need to use external libraries to implement routing features in React.
Most developers use react-router
to implement navigation and routing features in React.
React Router Dynamic Routes
If you’re going to build a web application that has more than one page, you’re going to need to define routes, which are patterns for URLs.
For example, you can use react-router
to specify that if the URL is www.mywebsite.com/
, React should display your Home
component.
Things get a little more complicated when your application has many pages of the same kind. For example, it is common that when you visit a blog, each post has its title in the URL, like this:
When you have thousands of posts or products, you can’t manually define routes for each. Fortunately, React Router allows you to easily create dynamic routes that automatically generate a URL for a specific item.
This article will show how to achieve such functionality in React apps.
React Router Custom Components
First, let’s start with four custom components you will import. These are the most commonly used custom components from the react-router
library.
The <BrowserRouter>
is the main component that handles navigation between pages. It works with the browser to display the right page for each URL.
The <BrowserRouter>
needs to wrap around the entire application. You can only use other custom components between this wrapper’s opening and closing tags.
Read official documentation to learn more.
We use the <Routes>
and its children <Route>
components to specify what needs to be rendered based on the current URL (location). Learn more here.
Finally, the <Link>
component is a modified version of HTML’s <a>
element. It provides a smoother transition from one page to another.
When users click on a <Link>
URL, React app does not reload. It immediately takes users to the destination.
Find out more about <Link>
component here.
React Router Dynamic Routes Example
Let’s look at a practical example of an application with three posts.
import "./styles.css";
import { BrowserRouter, Routes, Link, Route } from "react-router-dom";
import { useParams } from "react-router-dom";
export default function App() {
return (
<BrowserRouter>
<Routes>
<Route path="posts/:title" element={<Post />} />
<Route path="/" element={<Home />} />
</Routes>
</BrowserRouter>
);
}
function Home() {
var posts = [
{ title: "Guide to React" },
{ title: "Guide to Vue" },
{ title: "Guide to Angular" }
];
return (
<div className="App">
Hello
{posts.map((post) => (
<>
<br />
<Link to={"posts/" + post.title}>{post.title}</Link>
<br />
</>
))}
</div>
);
}
function Post() {
const urlParameters = useParams();
return <h1>{urlParameters.title}</h1>;
}
You can play with the code on CodeSandbox.
First, we import four custom components - <BrowserRouter>
, <Routes>
, <Route>
, <Link>
. We also import the useParams
hook, which we will explain later.
We have a main component, App
, which contains <Route>
components to map paths with components.
The first instance of the <Route>
component is fairly simple. It tells React to render the <Home>
component if the visitor is on the '/'
page ('website.com/'
).
The second instance of the <Route>
component is different. Its path
attribute is equal to "posts/:title"
string.
This means it will display the <Post>
component if the URL matches "posts/:title"
. The semicolon :
means that the title
part of the URL can be dynamically generated.
If the post has a title of 'Guide to React'
, the URL will be 'website.com/posts/Guide To React'
.
We generate the dynamic URL in the Home
component. We have an array of three posts, each of them with unique titles.
We use the .map()
JavaScript method to generate custom <Link>
components that create unique links for each post.
The most important part is the to
attribute of the <Link>
custom component. Let’s take a closer look.
<Link to={"posts/" + post.title}>{post.title}</Link>
We concatenate two strings: "posts"
and post.title
value, which is unique for each post. As a result, each URL will have the post’s title in the URL.
Access Values From the URL
Each <Route>
component specifies the URL path and what component needs to be rendered based on the URL. Once the <Post>
child component is rendered, you can use the useParams()
hook to access the :title
variable from the URL.
function Post() {
const urlParameters = useParams();
return <h1>{urlParameters.title}</h1>;
}
We use the {urlParameters.title}
syntax to display the value from URL parameters. Any JavaScript expression needs to be wrapped in curly braces.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn