How to Go Back to the Previous Page Using React Router
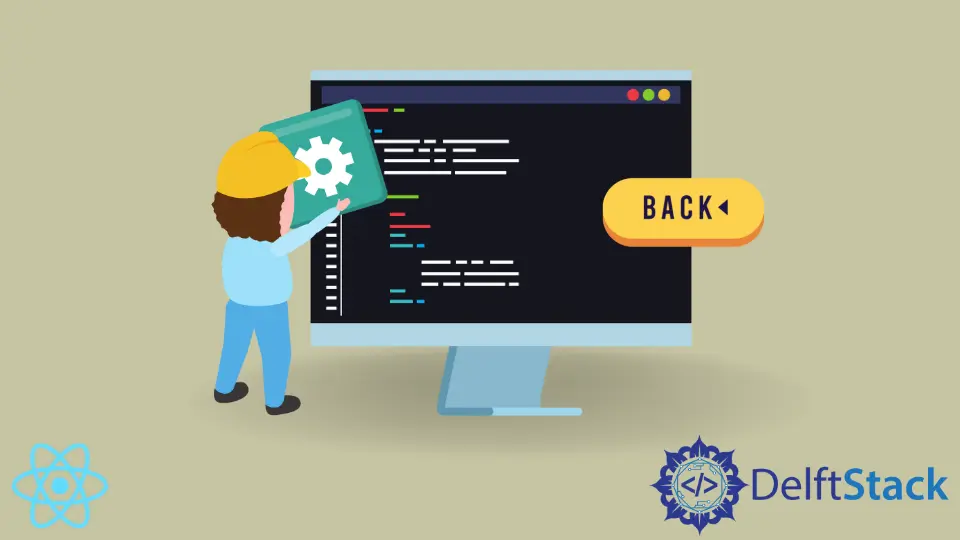
React is the most commonly used front-end library today. We can’t call it a framework because it does not have navigation and other essential features; hence, React developers usually import external libraries like react-router
to implement navigation features.
This article aims to help you implement essential navigation features in React, specifically, how to instruct React Router to return to the previous page.
Go Back to the Previous Page Using React Router
Anyone with experience browsing the internet understands how essential the go-back feature is. It is a good UX practice to implement a feature within your React app that lets your users navigate back to the previous page or URL.
History Stack
Internet browsers keep a record of your actions. History stack is a collection of your steps as you navigate the website.
History stack is a browser feature, but the react-router
library also stores this information in the history
object. You can work with this object to help users smoothly navigate your application.
Go Back to the Previous Page Using React Router v4 and v5
In the previous versions of the react-router
library, we used the useHistory()
hook to navigate to a specific page. It provides access to the instance of the history
object.
You can use the .push()
, .pop()
, and .replace()
methods to change the URL as needed. The history
instance has a goBack()
method, which allows us to navigate to the previous page.
Let’s take a look at the example:
function NavigateHome() {
let history = useHistory();
function handleClick() {
history.push("/somePage");
}
function goBack() {
history.goBack();
}
return (
<div>
<button onClick={handleClick}>
Go to some page
</button>
<button onClick={goBack}>
Go back
</button>
</div>
);
}
In this example, whenever a user clicks on the first button, react-router
will take the user to the URL passed as an argument to the push()
method. However, if the user clicks on the second button, they will be returned to the previous page.
Go Back to the Previous Page Using React Router v6
The newest version of the React Router library does not support the useHistory()
hook anymore. Developers of the library recommend using the useNavigate()
hook instead.
Unlike history
, the navigate
API does not require you to use .push()
and other JavaScript methods. It takes the argument itself.
Also, instead of using the .goBack()
method, you need to change the argument to the navigate
instance. All you need to do is to call navigate
with -1
as an argument.
The example from above can be rewritten with React Router v6, which uses the navigate
API:
import { useNavigate, Link, BrowserRouter as Router } from "react-router-dom";
export default function App() {
let navigate = useNavigate();
let goToAnotherPage = () => {
navigate("/another");
};
let goToSomePage = () => {
navigate("/somepage");
};
let goBack = () => {
navigate(-1);
};
return (
<div className="App">
<span
onClick={goToAnotherPage}
style={{ backgroundColor: "yellow", fontSize: 33, padding: 5 }}
>
Go To Another Page
</span>
<br />
<span
onClick={goToSomePage}
style={{ backgroundColor: "yellow", fontSize: 33, padding: 5 }}
>
Go To Some Page
</span>
<br />
<span
onClick={goBack}
style={{ backgroundColor: "lightblue", fontSize: 33, padding: 5 }}
>
Go Back
</span>
</div>
);
}
In this example, we have three buttons. The first two take users to a specific URL, and the third returns them to the previous location.
We used the navigate
API to do it; navigate
is a function that accepts the destination URL as an argument. If you provide -1
as an argument, it will go back to the previous page.
You can play with the code on CodeSandbox.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn