React Button onClick to Redirect Page
-
What Does the
onClick
Function Do -
How Do We Combine
onClick
WithReact-Router-Dom
-
React Button
onClick
to Redirect Page - Conclusion
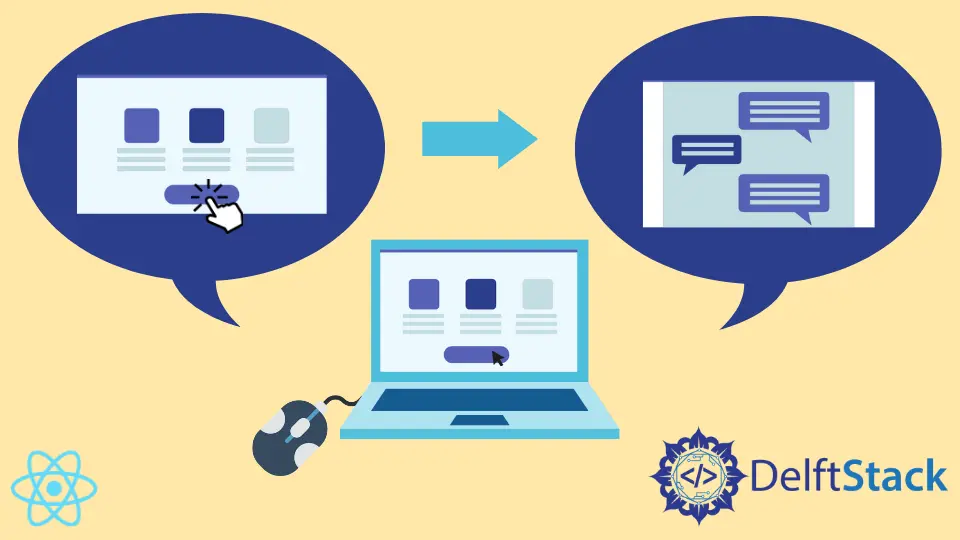
Thanks to the react-router-dom
function, web developers can easily create Single Page Apps that can navigate to another page when creating a multipage app. But what happens when we attempt to use the onClick
function alongside the react-router-dom
function?
What Does the onClick
Function Do
The onClick
function is an event listener. Its function is programmed to an element such that when that element (link or button) is clicked, the onClick
function is activated and carries out the function it is designed or programmed for.
For example, we want that when a link is clicked, the link should take us to another page or bring a pop-up message; this is the function that the onClick
event carries out.
How Do We Combine onClick
With React-Router-Dom
The usual practice with redirecting to another page on React is to use the react-router-dom
package; then, we apply the Route exact path
and the Link
function to complete the coding process.
The processes are mostly similar to the explanation above when we attempt to use the onClick
event listener with the react-router-dom
package.
We need to create other pages that we will redirect to; the difference comes mostly in the coding and that the onClick
is an event listener, a function more conducive to creating pop-up messages, among other things.
React Button onClick
to Redirect Page
To begin every React project, we start by putting this code in the terminal to create a fresh React app: npx create-react-app examplefive
. Once the project app is created, we need to install the react-router-dom
package using npm i react-router-dom
.
Next, we open the project folder in our text editor and create two more files to serve as the pages we want to redirect. We will head into the src
folder in the examplefive
project folder and create two more files, Home.js
and Contact.js
.
After this, we go through the proper coding, starting with the App.js
, like below.
Codding Snippet App.js
:
import React from "react";
import { BrowserRouter as Router, Routes, Route } from "react-router-dom";
import Home from "./Home";
import Contact from "./Contact";
const App = () => {
return (
<div style={styles.app}>
<Router>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/contact" element={<Contact />} />
</Routes>
</Router>
</div>
);
};
export default App;
const styles = {
app: {
padding: 50,
},
};
Here, we add code snippets that indicate the pages we want to navigate to after clicking a button.
After this, we navigate to the Home.js
to do some more coding.
Code Snippet Home.js
:
import React from "react";
import { useNavigate } from "react-router-dom";
const Home = (props) => {
const navigate = useNavigate();
return (
<>
<h1>Home Page</h1>
<p>Go to the contact page by using one of the following:</p>
<hr />
{/* Button */}
<p>
<button onClick={() => navigate("/contact")}>Go to Contact</button>
</p>
{/* Checkbox */}
<p>
<input
type="checkbox"
onChange={() => navigate("/contact")}
></input>
<span>Check this checkbox to go to the contact page</span>
</p>
{/* Text field */}
<p>
<input
type="text"
onChange={(e) => {
if (e.target.value === "contact") {
navigate("/contact");
}
}}
placeholder="Enter 'contact' to navigate"
></input>
</p>
</>
);
};
export default Home;
The Home.js
, the main page, houses most of the content and the coding. We can see that the onClick
event listener is linked to the contact
button such that once it is clicked, it navigates us to the contact page.
Code Snippet Contact.js
:
import React from "react";
import { useNavigate } from "react-router-dom";
const Contact = (props) => {
const navigate = useNavigate();
return (
<>
<h1>Contact Page</h1>
<br />
<button onClick={() => navigate(-1)}>Go Back</button>
</>
);
};
export default Contact;
Like the Home.js
file, we link the onClick
event listener to the Go Back
button; when this button is clicked, we are returned to the homepage.
Output:
Conclusion
The onClick
event listener is yet another solution that can be developed to create multipage applications like the react-router-dom
method. However, there has not been a known method to redirect to another page without using the react-router-dom
package.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn