React Native View onPress
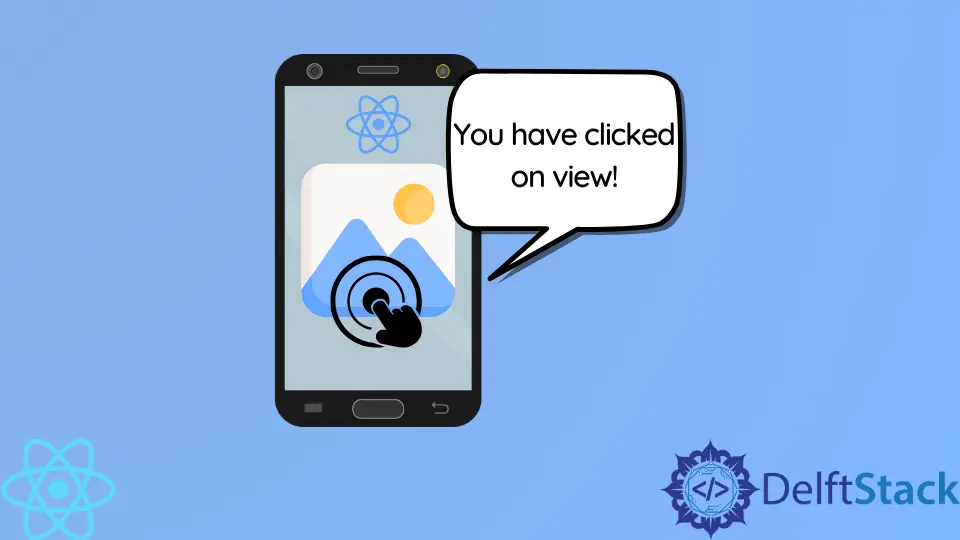
This tutorial teaches you how to configure onPress on a React Native view.
React Native View onPress
We need to set onPress on numerous scenarios when dealing with React Native components, but we must surround it with React Native Touchables.
What if we could set onPress on the View container itself? It is possible with onStartShouldSetResponder
, which only works with React Native version 55.3. Let’s learn it with the following code fence.
Example Code:
import React from 'react';
import {SafeAreaView, StyleSheet, Text, View} from 'react-native';
const App = () => {
return (
<SafeAreaView style={{
flex: 1 }}>
<View style={styles.container}>
<View
style={styles.innerView}
onStartShouldSetResponder={() => alert('You have clicked on view')}
>
<Text style={styles.paragraph}>Click on a View</Text>
</View>
</View>
</SafeAreaView>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
paddingTop: 25,
backgroundColor: '#72d4ed',
padding: 8,
},
innerView: {
flex: 1,
justifyContent: 'center',
paddingTop: 25,
backgroundColor: '#f77979',
marginTop: 200,
marginBottom: 200,
marginLeft: 20,
marginRight: 20,
},
paragraph: {
margin: 24,
fontSize: 18,
color: 'white',
fontWeight: 'bold',
textAlign: 'center',
},
});
export default App;
Buttons are utilized in all programming languages to capture user click events. However, in react native, we may activate the click onPress event on the View component by using its onStartShouldSetResponder
Prop.
Because we need to make the View component responsive at times or fire an event when the user clicks on View. So we have the onStartShouldSetResponder
property. It functions similarly to the button onPress event and may handle function calls.
When we set onStartShouldSetResponder
to true
, we’re telling our gesture responder that this View should get all of the motion events that occur on this portion of the screen.
Even if I move my hand over the cursor view, which is a parent’s child, it shouldn’t have any effect because only the parent has asked to be the touch responder. Remember, we have to request it; else, it will not be interactive by default.
Output:
When you run the code, you will see something like this:
As you can see, there is a button called click on view
; when you click on that, it triggers the onpress method, which will give a popup saying You have clicked on view
.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn