How to Format Currency in React Native
- Using JavaScript’s Intl.NumberFormat
- Using the Numeral.js Library
- Using React Native Number Format Library
- Conclusion
- FAQ
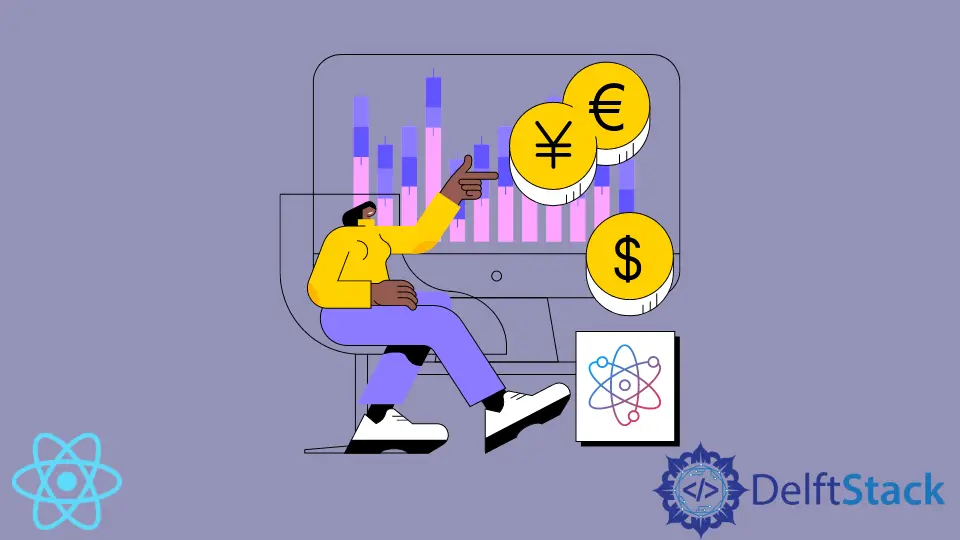
When developing applications in React Native, displaying currency correctly is crucial for ensuring a great user experience. Whether you’re building an e-commerce app or a financial tracking tool, formatting numbers as currency can make your application more intuitive and user-friendly.
In this article, we will explore various methods to format currency in React Native, helping you to present monetary values in a way that is clear and professional. We’ll cover built-in JavaScript methods, popular libraries, and best practices to ensure that your application meets the needs of your users. Let’s dive in!
Using JavaScript’s Intl.NumberFormat
One of the simplest ways to format currency in React Native is by using JavaScript’s built-in Intl.NumberFormat
object. This method allows you to format numbers based on the locale and currency you specify. It’s efficient, lightweight, and doesn’t require any additional libraries.
Here’s how you can use it:
const formatCurrency = (amount, currency) => {
return new Intl.NumberFormat('en-US', {
style: 'currency',
currency: currency,
}).format(amount);
};
const formattedAmount = formatCurrency(123456.78, 'USD');
console.log(formattedAmount);
Output:
$123,456.78
The formatCurrency
function takes two parameters: the amount
to be formatted and the currency
code (like ‘USD’ for US dollars). The Intl.NumberFormat
constructor creates a new object that formats the number according to the specified locale and currency style. In this example, we’re formatting an amount of 123456.78 as US dollars. The output is a nicely formatted string that includes the dollar sign and commas separating the thousands.
This method is particularly useful because it’s versatile and can easily adapt to different currencies and locales. Simply change the currency code and locale string to format numbers accordingly.
Using the Numeral.js Library
For those who prefer using libraries, Numeral.js is a fantastic choice for formatting numbers in JavaScript and React Native. It provides a simple API for formatting numbers, including currency, percentages, and more.
To get started, first, you need to install Numeral.js:
npm install numeral
Once installed, you can format currency like this:
import numeral from 'numeral';
const formattedAmount = numeral(123456.78).format('$0,0.00');
console.log(formattedAmount);
Output:
$123,456.78
In this example, we import the Numeral.js library and use the format
method to create a currency string. The format string '$0,0.00'
specifies that we want the number to have a dollar sign, commas for thousands, and two decimal places. This method is very flexible, allowing you to customize the format to suit your needs.
Numeral.js is particularly advantageous when you need to format numbers in various ways throughout your application. By using a single library, you can maintain consistency in number formatting, which enhances the overall user experience.
Using React Native Number Format Library
Another excellent option for formatting currency in React Native is the react-native-number-format
library. This library provides a simple way to format numbers directly in your components, making it ideal for use in forms and displays.
To use this library, first, install it:
npm install react-native-number-format
Here’s a quick example of how to use it:
import NumberFormat from 'react-native-number-format';
const MyComponent = () => {
return (
<NumberFormat
value={123456.78}
displayType={'text'}
thousandSeparator={true}
prefix={'$'}
decimalScale={2}
fixedDecimalScale={true}
renderText={value => <Text>{value}</Text>}
/>
);
};
Output:
$123,456.78
In this example, we create a functional component called MyComponent
. Inside it, we use the NumberFormat
component to format the number 123456.78. The displayType
prop is set to ’text’, which means the formatted value will be displayed as text. The thousandSeparator
, prefix
, decimalScale
, and fixedDecimalScale
props customize the output to display as currency.
This method is particularly useful for forms where users input numbers. It provides real-time formatting, which can enhance user experience by showing them how their input will appear as they type.
Conclusion
Formatting currency in React Native is essential for creating a polished, user-friendly application. Whether you choose to use JavaScript’s built-in Intl.NumberFormat
, a library like Numeral.js, or the react-native-number-format
library, each method has its advantages. By understanding these options, you can effectively present monetary values in your applications, ensuring clarity and professionalism. Choose the method that best fits your project’s needs and watch your application’s usability soar!
FAQ
-
What is the best way to format currency in React Native?
The best way depends on your project requirements. You can use JavaScript’sIntl.NumberFormat
, Numeral.js, or thereact-native-number-format
library. -
Can I format currency for different locales?
Yes, you can format currency for different locales usingIntl.NumberFormat
by specifying the appropriate locale string. -
Is it necessary to use a library for currency formatting?
No, it’s not necessary. JavaScript’s built-in methods can handle most formatting needs, but libraries can provide additional features and convenience. -
How can I format currency in user input fields?
You can use thereact-native-number-format
library to format currency in real-time as users input their values. -
Are there performance concerns with formatting currency in React Native?
Generally, formatting currency is not resource-intensive, but if you’re formatting large datasets or doing it frequently, consider optimizing your code.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn