How to Use the onChange Event in React
- Understanding the onChange Event
- Implementing onChange with Text Inputs
- Working with Checkboxes
- Using onChange with Select Dropdowns
- Best Practices for Using onChange
- Conclusion
- FAQ
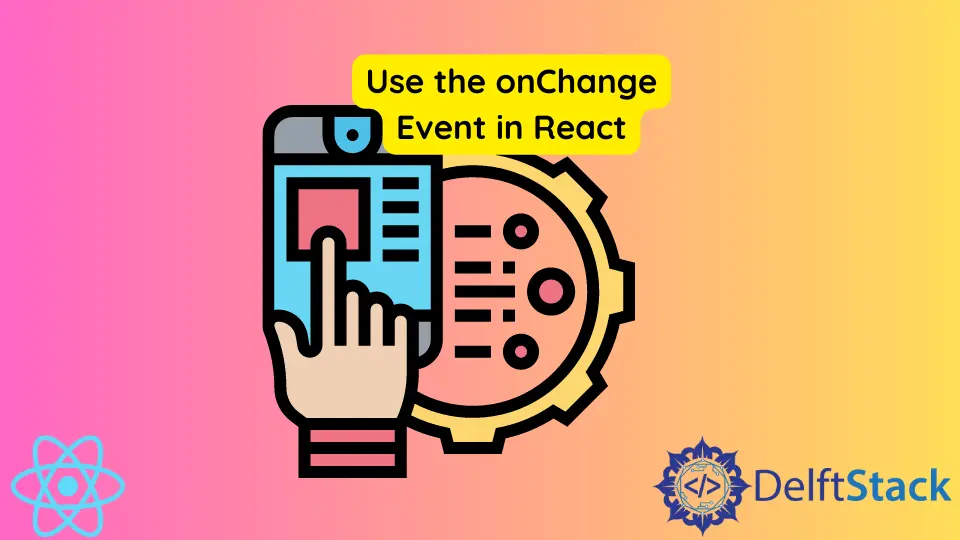
The onChange event is a fundamental aspect of working with forms in React. It allows developers to capture user input dynamically, making it essential for creating interactive web applications. Whether you’re handling text input, checkboxes, or drop-downs, understanding how to implement the onChange event can significantly enhance your user experience.
In this article, we’ll delve into the mechanics of the onChange event in React, provide practical examples, and explore best practices to ensure your forms are both functional and user-friendly. Let’s get started on mastering this crucial event in React!
Understanding the onChange Event
The onChange event in React is triggered when the value of an input element changes. This event is commonly used with form elements like <input>
, <textarea>
, and <select>
. When a user types in a text box or selects an option from a dropdown, the onChange event fires, allowing you to capture this change and update your component’s state accordingly.
One of the key features of the onChange event in React is that it works seamlessly with controlled components. A controlled component is one where the form data is handled by the component’s state. This means that the input’s value is derived from the state, and any changes to the input will trigger a state update. This two-way binding ensures that your UI is always in sync with the underlying data.
Implementing onChange with Text Inputs
To illustrate the use of the onChange event, let’s create a simple text input component. This component will capture user input and display it in real time.
import React, { useState } from 'react';
function TextInput() {
const [inputValue, setInputValue] = useState('');
const handleChange = (event) => {
setInputValue(event.target.value);
};
return (
<div>
<input type="text" value={inputValue} onChange={handleChange} />
<p>You typed: {inputValue}</p>
</div>
);
}
export default TextInput;
Output:
You typed: [user input]
In this example, we start by importing React and the useState hook. The TextInput
component maintains a piece of state called inputValue
, initialized to an empty string. The handleChange
function updates this state whenever the input changes. As the user types, the onChange
event triggers the handleChange
function, which updates the displayed text in real time. This approach ensures that the input field and the paragraph displaying the typed text are always in sync.
Working with Checkboxes
Checkboxes are another common form element where the onChange event plays a crucial role. Let’s look at how we can implement a checkbox that toggles a message based on its checked state.
import React, { useState } from 'react';
function CheckboxExample() {
const [isChecked, setIsChecked] = useState(false);
const handleCheckboxChange = (event) => {
setIsChecked(event.target.checked);
};
return (
<div>
<label>
<input type="checkbox" checked={isChecked} onChange={handleCheckboxChange} />
Check me!
</label>
{isChecked && <p>You checked the box!</p>}
</div>
);
}
export default CheckboxExample;
Output:
You checked the box!
In this example, we create a checkbox that updates its state based on whether it is checked or not. The isChecked
state is initialized to false. The handleCheckboxChange
function updates this state when the checkbox is toggled. If the checkbox is checked, a message is displayed. This method allows for a simple yet effective way to manage user interactions with checkboxes in your React applications.
Using onChange with Select Dropdowns
Select dropdowns are another area where the onChange event shines. Let’s create a simple dropdown that updates a displayed message based on the selected option.
import React, { useState } from 'react';
function SelectDropdown() {
const [selectedOption, setSelectedOption] = useState('Option 1');
const handleSelectChange = (event) => {
setSelectedOption(event.target.value);
};
return (
<div>
<select value={selectedOption} onChange={handleSelectChange}>
<option value="Option 1">Option 1</option>
<option value="Option 2">Option 2</option>
<option value="Option 3">Option 3</option>
</select>
<p>You selected: {selectedOption}</p>
</div>
);
}
export default SelectDropdown;
Output:
You selected: [selected option]
In this example, we create a dropdown menu with three options. The selectedOption
state holds the currently selected value, initialized to “Option 1”. The handleSelectChange
function updates this state whenever the user selects a different option from the dropdown. This allows us to display the currently selected option dynamically, showcasing how to effectively manage select elements in React.
Best Practices for Using onChange
When working with the onChange event in React, there are several best practices to keep in mind. Firstly, always ensure that your input elements are controlled components. This means that their values should be derived from the component’s state, allowing for a more predictable and manageable form state.
Secondly, consider debouncing your onChange handlers for performance, especially when dealing with large forms or complex computations. Debouncing limits the rate at which a function can fire, preventing unnecessary re-renders and improving application performance.
Lastly, always validate user input to ensure data integrity. Implementing validation logic can help catch errors early and improve the user experience by providing immediate feedback.
Conclusion
Understanding how to use the onChange event in React is crucial for creating interactive and user-friendly applications. By mastering this event, you can effectively manage user input across various form elements, ensuring that your UI remains in sync with your application state. Whether you’re working with text inputs, checkboxes, or dropdowns, the onChange event is a powerful tool in your React toolkit. Keep practicing and exploring different use cases to enhance your skills further!
FAQ
-
What is the onChange event in React?
The onChange event in React is triggered when the value of an input element changes, allowing developers to capture user input dynamically. -
How do I use onChange with text inputs in React?
You can use the onChange event with text inputs by creating a controlled component where the input’s value is derived from the component’s state. -
Can I use onChange with checkboxes in React?
Yes, the onChange event works well with checkboxes, allowing you to manage their checked state effectively. -
What are controlled components in React?
Controlled components are form elements whose values are controlled by the component’s state, ensuring that the UI is always in sync with the underlying data. -
How can I improve performance when using onChange?
You can improve performance by debouncing your onChange handlers to limit the rate at which they fire, preventing unnecessary re-renders.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn