The Hooks-Based Alternative to componentDidUpdate() Lifecycle Method in React
- Lifecycle Methods in Class Components in React
-
How to Implement
componentDidUpdate()
With Hooks in React
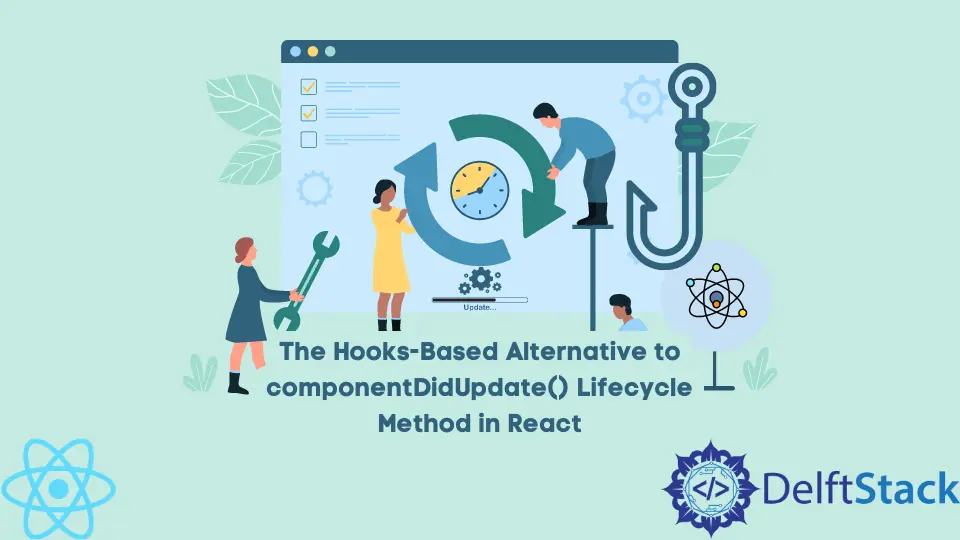
React is a component-based library for building beautiful interfaces using reusable components. Besides many useful features such as Virtual DOM and state management, React also allows us to execute certain code during a certain stage of a component’s lifecycle.
Lifecycle Methods in Class Components in React
Lifecycle methods allow us to execute code for side effects, such as loading data from an external source, setting up subscriptions, or other functions.
For instance, the componentDidMount()
lifecycle method allows you to execute the code when the component is mounted (or, in other words, rendered for the first time).
You can look at the live demo on codesandbox.
import { Component, useRef, useEffect } from "react";
export default class App extends Component {
componentDidMount() {
console.log("mounted!");
}
render() {
return <h1>Hello World</h1>;
}
}
If you check the console, you’ll see that the string argument to the console.log()
function is logged to the console.
The other major lifecycle method is componentDidUpdate()
, which executes every component state change, triggering the update. Let’s look at an example.
export default class App extends Component {
constructor(props) {
super(props);
this.state = {
boolean: false
};
}
componentDidMount() {
console.log("mounted!");
}
componentDidUpdate() {
console.log("updated!");
}
render() {
return (
<button onClick={() => this.setState({ boolean: !this.state.boolean })}>
Change the State
</button>
);
}
}
The component includes a button, which changes the state value. The state changes every time you click a button in the demo, triggering the update. The string "updated"
will be logged to the console every time the state changes if you look in the console.
You should read this article to learn more about typical lifecycle components for class components.
How to Implement componentDidUpdate()
With Hooks in React
Lifecycle methods are not available for functional components. However, a useEffect()
hook is an alternative to the methods above.
In order to recreate the functionality of componentDidUpdate()
lifecycle method in functional components, we can use the useRef()
hook to create an instance variable in the functional component.
Then we can conditionally check the current
property of the variable to execute a code in different lifecycles of a functional component.
Let’s look at an example.
function SomeComponent() {
const status = useRef();
useEffect(() => {
if (!status.current){
// componentDidMount
status.current = true
}
else {
// componentDidUpdate
console.log("updated")
}
});
return <h1>A sample component</h1>;
}
In this example, we use two conditional blocks: one to execute code when the component mounts and another to execute when it updates.
When the component is first created, you can set its current
property to true
, and after that, every time the callback function in the useEffect()
function is called, it will execute the code in the else
block.
You can check the demo on codesandbox.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn