How to Force Rerender Using React Hooks
- Understanding React Hooks
- Method 1: Using useState Hook
- Method 2: Using useReducer Hook
- Method 3: Using useEffect for Side Effects
- Conclusion
- FAQ
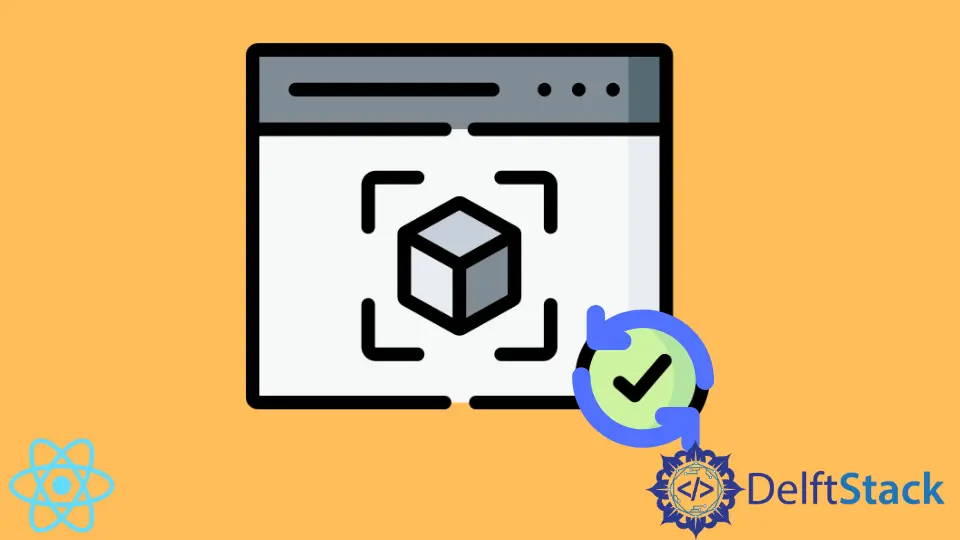
The introduction of React Hooks has revolutionized how we write and structure our React components. Hooks allow developers to use state and other React features without writing a class. However, one common challenge developers face is how to force a component to rerender. Whether you’re dealing with data updates or just need to refresh the UI, understanding how to trigger a rerender can significantly enhance your application’s interactivity.
In this article, we’ll explore various methods to force rerender using React Hooks, ensuring your components stay up-to-date and responsive to user actions.
Understanding React Hooks
Before diving into the methods of forcing a rerender, it’s essential to have a solid grasp of what React Hooks are. React Hooks, introduced in version 16.8, allow you to use state and lifecycle features in functional components. The most commonly used hooks are useState
and useEffect
.
The useState
hook lets you add state to your functional components, while useEffect
allows you to perform side effects, such as data fetching or subscriptions. By leveraging these hooks, you can create dynamic and interactive user interfaces. However, there are scenarios where you might need to force a rerender, especially when the state doesn’t change but the UI still needs to reflect some updates.
Method 1: Using useState Hook
One of the simplest ways to force a rerender in a React component is by using the useState
hook. By updating the state with a new value, you can trigger a rerender. This method is straightforward and effective.
import React, { useState } from 'react';
const RerenderExample = () => {
const [count, setCount] = useState(0);
const forceRerender = () => {
setCount(count + 1);
};
return (
<div>
<p>Current Count: {count}</p>
<button onClick={forceRerender}>Force Rerender</button>
</div>
);
};
export default RerenderExample;
Output:
Current Count: 0
In the example above, we create a functional component called RerenderExample
. We utilize the useState
hook to manage a count state. When the button is clicked, the forceRerender
function is called, which increments the count by one. This change in state triggers a rerender, updating the displayed count accordingly. This method is particularly useful when you want to refresh the UI based on user interaction.
Method 2: Using useReducer Hook
Another effective way to force a rerender is by using the useReducer
hook. This hook is beneficial when you have complex state logic or want to manage state transitions in a more structured way.
import React, { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'reset':
return initialState;
default:
throw new Error();
}
}
const RerenderWithReducer = () => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'reset' })}>Reset</button>
</div>
);
};
export default RerenderWithReducer;
Output:
Count: 0
In this example, we define a reducer
function that manages the count state. The useReducer
hook returns the current state and a dispatch function. By clicking the “Increment” button, we dispatch an action that updates the count, causing a rerender. The “Reset” button resets the count to its initial state. This method provides a clear and scalable way to manage state changes and rerenders.
Method 3: Using useEffect for Side Effects
The useEffect
hook can also be utilized to force a rerender, especially when dealing with side effects or external data. By updating the state inside an effect, you can trigger a rerender based on specific conditions.
import React, { useState, useEffect } from 'react';
const RerenderWithEffect = () => {
const [count, setCount] = useState(0);
const [data, setData] = useState(null);
useEffect(() => {
// Simulating a data fetch
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
};
fetchData();
}, [count]); // Rerun effect when count changes
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<p>Data: {JSON.stringify(data)}</p>
</div>
);
};
export default RerenderWithEffect;
Output:
Count: 0
Data: null
In this code, we use the useEffect
hook to simulate a data fetch whenever the count
state changes. By updating the data
state, we can trigger a rerender of the component. This method is particularly useful when you want to synchronize your component with external data sources or APIs. The combination of useEffect
and state updates allows for a dynamic and responsive user experience.
Conclusion
Forcing a rerender in React using hooks is an essential skill for any developer looking to create dynamic and interactive applications. Whether you choose to use useState
, useReducer
, or useEffect
, each method has its advantages and can be tailored to fit your specific needs. By understanding these techniques, you can ensure that your components remain responsive to user interactions and data changes. Embrace the power of React Hooks, and elevate your application to new heights of interactivity.
FAQ
-
How do React Hooks improve component functionality?
React Hooks allow developers to manage state and lifecycle events in functional components, making code more concise and easier to read. -
Can I use multiple hooks in a single component?
Yes, you can use multiple hooks in a single component, but they must be called in the same order on every render. -
What is the difference between useState and useReducer?
useState
is ideal for simple state management, whileuseReducer
is better suited for complex state logic with multiple sub-values or when the next state depends on the previous one. -
When should I use useEffect?
UseuseEffect
for side effects such as data fetching, subscriptions, or manually changing the DOM. -
Is it possible to force a rerender without changing state?
Yes, you can force a rerender by using a method likesetState
with a new value, even if the value is the same.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn