React 中基于 Hooks 的 componentDidUpdate() 生命周期方法的替代方案
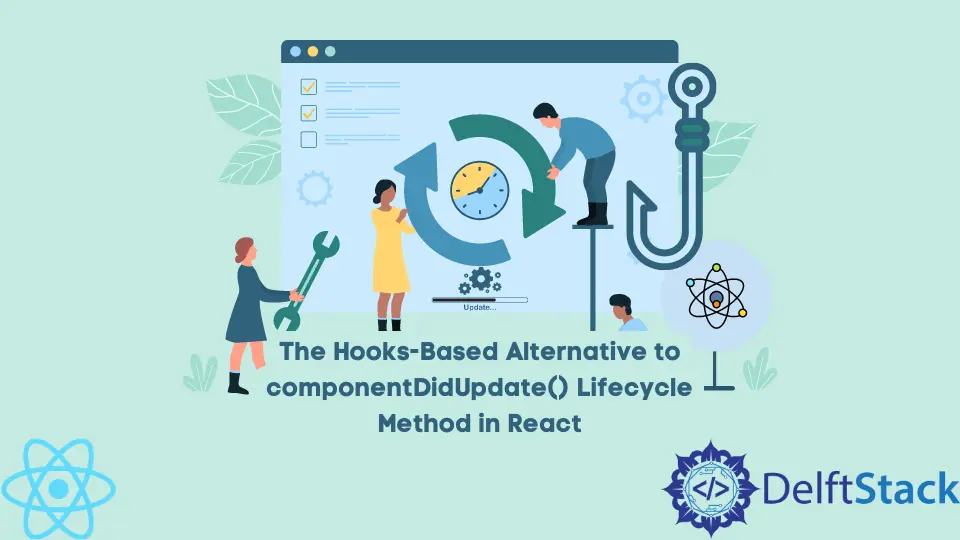
React 是一个基于组件的库,用于使用可重用组件构建漂亮的界面。除了 Virtual DOM 和状态管理等许多有用的功能外,React 还允许我们在组件生命周期的某个阶段执行某些代码。
React 中类组件中的生命周期方法
生命周期方法允许我们执行副作用代码,例如从外部源加载数据、设置订阅或其他功能。
例如,componentDidMount()
生命周期方法允许你在组件被挂载(或者,换句话说,第一次渲染)时执行代码。
你可以在 codesandbox 上查看演示。
import { Component, useRef, useEffect } from "react";
export default class App extends Component {
componentDidMount() {
console.log("mounted!");
}
render() {
return <h1>Hello World</h1>;
}
}
如果你检查控制台,你会看到 console.log()
函数的字符串参数已记录到控制台。
另一个主要的生命周期方法是 componentDidUpdate()
,它执行每个组件状态更改,触发更新。让我们看一个例子。
export default class App extends Component {
constructor(props) {
super(props);
this.state = {
boolean: false
};
}
componentDidMount() {
console.log("mounted!");
}
componentDidUpdate() {
console.log("updated!");
}
render() {
return (
<button onClick={() => this.setState({ boolean: !this.state.boolean })}>
Change the State
</button>
);
}
}
该组件包含一个按钮,用于更改状态值。每次单击 demo 中的按钮时,状态都会发生变化,从而触发更新。如果你在控制台中查看,每次状态更改时,字符串 "updated"
都会记录到控制台。
你应该阅读本文以了解有关类组件的典型生命周期组件的更多信息。
在 React 中如何使用 Hooks 实现 componentDidUpdate()
生命周期方法不适用于功能组件。但是,useEffect()
挂钩是上述方法的替代方法。
为了在功能组件中重新创建 componentDidUpdate()
生命周期方法的功能,我们可以使用 useRef()
挂钩在功能组件中创建实例变量。
然后我们可以有条件地检查变量的 current
属性以在功能组件的不同生命周期中执行代码。
让我们看一个例子。
function SomeComponent() {
const status = useRef();
useEffect(() => {
if (!status.current){
// componentDidMount
status.current = true
}
else {
// componentDidUpdate
console.log("updated")
}
});
return <h1>A sample component</h1>;
}
在这个例子中,我们使用了两个条件块:一个在组件挂载时执行代码,另一个在组件更新时执行。
第一次创建组件时,可以将其 current
属性设置为 true
,之后每次调用 useEffect()
函数中的回调函数时,都会执行 else
中的代码堵塞。
你可以在 codesandbox 上查看演示。
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn