How to Paste Strings Without Spaces in R
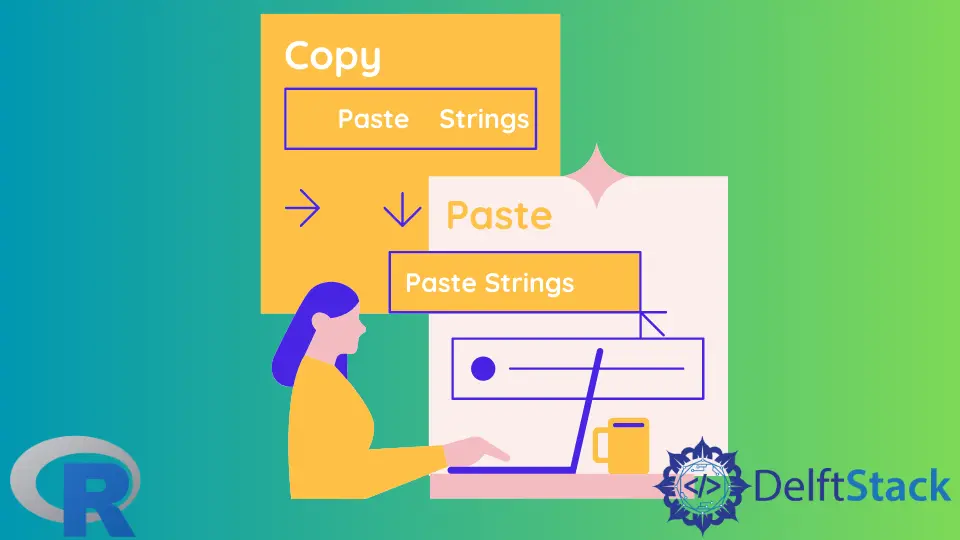
This article will explore three ways to remove spaces when merging (concatenating) strings.
Remove Spaces When Merging Strings in R
We will use individual strings, vectors of strings, and a data frame with strings for the demonstrations.
Example Code:
# The individual strings.
s1 = "The sky"
s2 = "is blue."
s1a = " The sky "
s2a = " is blue. "
# Columns for the data frame.
C1 = c("The sky", " The earth ")
C2 = c("is blue.", " is green. ")
# The data frame.
dtfr = data.frame(Col1=C1, Col2=C2)
Base R Functions
The following base R functions can be used to remove spaces from strings.
Use the paste()
Function With sep=""
to Remove Spaces
When pasting strings, R adds a space in between. We can use sep=""
to remove the added space.
In the context of vectors of the same length, paste()
merges corresponding elements of the vectors. To merge all output elements into one long string without added spaces, we can use collapse=""
.
To merge data frame columns without added spaces, use sep=""
.
Example Code:
# MERGE WITH paste()
# Default: adds space.
paste(s1, s2)
# Remove added space.
paste(s1, s2, sep="")
# Does not remove existing spaces.
# Variables s1a and s2a have space characters at the beginning and end.
paste(s1a, s2a, sep="")
# Element-wise merging of vectors.
# No spaces are added in between.
paste(C1, C2, sep="")
# After element-wise merging, we can
# collapse all the elements of the result into a single string.
paste(C1, C2, sep="", collapse="")
# Merge columns of a data frame.
# No spaces are added in between.
dtfr$Mr = paste(dtfr$Col1, dtfr$Col2, sep="")
dtfr
Output:
> # Default: adds space.
> paste(s1, s2)
[1] "The sky is blue."
> # Remove added space.
> paste(s1, s2, sep="")
[1] "The skyis blue."
> # Does not remove existing spaces.
> # Variables s1a and s2a have space characters at the beginning and end.
> paste(s1a, s2a, sep="")
[1] " The sky is blue. "
> # Element-wise merging of vectors.
> # No spaces are added in between.
> paste(C1, C2, sep="")
[1] "The skyis blue." " The earth is green. "
> # After element-wise merging, we can
> # collapse all the elements of the result into a single string.
> paste(C1, C2, sep="", collapse="")
[1] "The skyis blue. The earth is green. "
> # Merge columns of a data frame.
> # No spaces are added in between.
> dtfr$Mr = paste(dtfr$Col1, dtfr$Col2, sep="")
> dtfr
Col1 Col2 Mr
1 The sky is blue. The skyis blue.
2 The earth is green. The earth is green.
Use the trimws()
and paste()
Functions to Remove Spaces
The trimws()
function can remove whitespace
from the left, right, or both sides of a string. Besides space characters, other characters considered whitespace
include the horizontal tab, carriage return and new line characters.
By default, this function removes all whitespace from both sides of a string.
Syntax:
trimws(string, which="side", whitespace="[ \t\r\n]")
# where `side` can be `left` or `right` or `both`
Example Code:
# trimws() removes spaces from the left, right or both
trimws(s2a, which="left", whitespace=" ")
trimws(s2a, which="right", whitespace=" ")
trimws(s2a, which="both", whitespace=" ")
# By default, it removes all whitespace from both sides.
s2a
trimws(s2a)
# Remove leading, trailing and added spaces when merging vectors.
paste(trimws(C1), trimws(C2), sep="")
# Remove leading, trailing and added spaces when combining columns of a data frame.
dtfr$Mr2 = paste(trimws(dtfr$Col1), trimws(dtfr$Col2), sep="")
dtfr
Output:
> # trimws() removes spaces from the left, right or both
> trimws(s2a, which="left", whitespace=" ")
[1] "is blue. "
> trimws(s2a, which="right", whitespace=" ")
[1] " is blue."
> trimws(s2a, which="both", whitespace=" ")
[1] "is blue."
> # By default, it removes all whitespace from both sides.
> s2a
[1] " is blue. "
> trimws(s2a)
[1] "is blue."
> # Remove leading, trailing and added spaces when merging vectors.
> paste(trimws(C1), trimws(C2), sep="")
[1] "The skyis blue." "The earthis green."
> # Remove leading, trailing and added spaces when combining columns of a data frame.
> dtfr$Mr2 = paste(trimws(dtfr$Col1), trimws(dtfr$Col2), sep="")
> dtfr
Col1 Col2 Mr Mr2
1 The sky is blue. The skyis blue. The skyis blue.
2 The earth is green. The earth is green. The earthis green.
We first used trimws()
to remove spaces from both sides of strings before merging them using the paste()
function.
Use the gsub()
and paste()
Functions to Remove Spaces
Syntax:
gsub(search_for, replace_with, string)
Example Code:
# For vectors: merge strings and remove all spaces from the result.
gsub(" ", "", paste(C1, C2))
# For data frame: merge string columns of a data frame and remove all spaces.
dtfr$Mr3 = gsub(" ", "", paste(dtfr$Col1, dtfr$Col2))
dtfr
Output:
> # For vectors: merge strings and remove all spaces from the result.
> gsub(" ", "", paste(C1, C2))
[1] "Theskyisblue." "Theearthisgreen."
> # For data frame: merge string columns of a data frame and remove all spaces.
> dtfr$Mr3 = gsub(" ", "", paste(dtfr$Col1, dtfr$Col2))
> dtfr
Col1 Col2 Mr Mr2 Mr3
1 The sky is blue. The skyis blue. The skyis blue. Theskyisblue.
2 The earth is green. The earth is green. The earthis green. Theearthisgreen.
We first merge the strings using paste()
and then remove all spaces using gsub()
.
Use the stringr
Package and paste()
Function to Remove Spaces
Syntax:
str_replace_all(string, search_for, replace_with)
Example Code:
# Install the stringr package.
# install.packages("stringr")
# Load the stringr package.
library(stringr)
# With vectors.
str_replace_all(paste(C1, C2), " ", "")
# With columns of a data frame.
# We will first recreate the dataframe to improve readability.
dtfr = data.frame(Col1=C1, Col2=C2)
# Use the str_replace_all() function.
dtfr$StrRplAll = str_replace_all(paste(dtfr$Col1, dtfr$Col2), " ", "")
dtfr
Output:
> # Load the stringr package.
> library(stringr)
> # With vectors.
> str_replace_all(paste(C1, C2), " ", "")
[1] "Theskyisblue." "Theearthisgreen."
> # With columns of a data frame.
> # We will first recreate the dataframe to improve readability.
> dtfr = data.frame(Col1=C1, Col2=C2)
> # Use the str_replace_all() function.
> dtfr$StrRplAll = str_replace_all(paste(dtfr$Col1, dtfr$Col2), " ", "")
> dtfr
Col1 Col2 StrRplAll
1 The sky is blue. Theskyisblue.
2 The earth is green. Theearthisgreen.
The stringr
package of R’s tidyverse
is built on top of the stringi
package. This package provides the str_replace_all()
function, which is similar to the gsub()
function.
Conclusion
This article showed how to remove the following:
- Spaces are added when merging strings with the
paste()
function. - Leading and trailing spaces from strings.
- All spaces from a string.
The functions can be combined/nested depending on the need to get the desired output.