How to Concatenate Strings in R
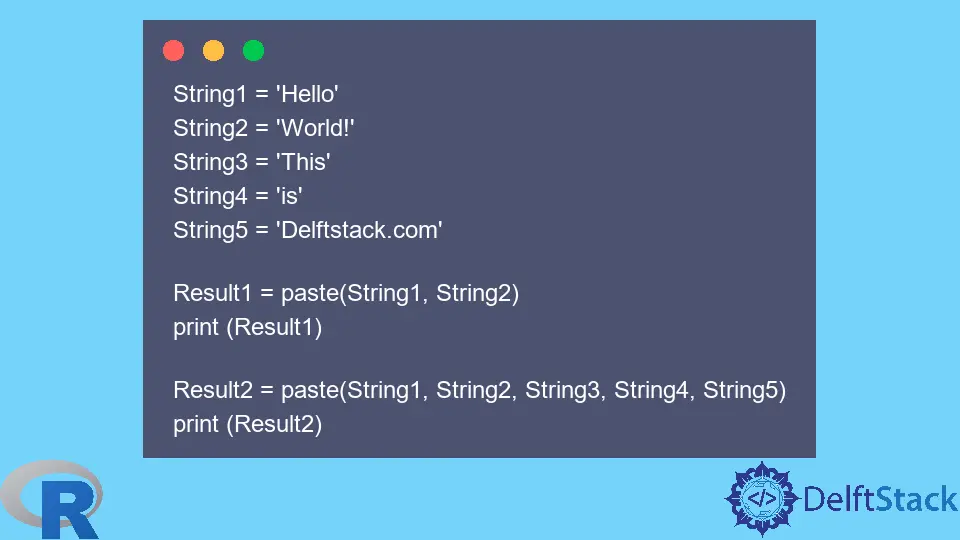
We can join two or more strings with concatenating strings; the R language has a few ways to concatenate strings.
This tutorial demonstrates how to concatenate strings in R.
Concatenate Strings Using the paste()
Method in R
paste()
is the most common way to concatenate strings in R. It takes one mandatory and two optional parameters.
The syntax of the paste()
function is:
paste(Strings, sep="", collapse=NULL)
Where:
Strings
are the strings you want to concatenate.sep
is the separator character that will be appended between two strings. It can be space or any other character.collapse
is an optional character to be used as a separator.
Let’s try a few examples.
Concatenate Strings Using paste()
Simple concatenation means without any separator or collapse. It should be mentioned here that the default separator for paste()
is space.
Example:
String1 = 'Hello'
String2 = 'World!'
String3 = 'This'
String4 = 'is'
String5 = 'Delftstack.com'
Result1 = paste(String1, String2)
print (Result1)
Result2 = paste(String1, String2, String3, String4, String5)
print (Result2)
The code above first concatenates two strings and then more than two strings.
Output:
[1] "Hello World!"
[1] "Hello World! This is Delftstack.com"
Concatenate Strings Using paste()
With sep
sep
means the separator. The example below demonstrates the concatenation with and without separators.
Example:
String1 = 'Hello'
String2 = 'World!'
String3 = 'This'
String4 = 'is'
String5 = 'Delftstack.com'
# without any separator
Result1 = paste(String1, String2, String3, String4, String5, sep="")
print (Result1)
# with space separator
Result2 = paste(String1, String2, String3, String4, String5, sep=" ")
print (Result2)
# with a symbol separator
Result3 = paste(String1, String2, String3, String4, String5, sep=",")
print (Result3)
The code concatenates strings without separators and with space and comma separators.
Output:
[1] "HelloWorld!ThisisDelftstack.com"
[1] "Hello World! This is Delftstack.com"
[1] "Hello,World!,This,is,Delftstack.com"
Concatenate Strings Using paste()
With collapse
To use collapse
, we must create a vector of strings first and then pass it to the paste()
method as a parameter with collapse
.
Example:
String1 <- 'Hello'
String2 <- 'World!'
String3 <- 'This'
String4 <- 'is'
String5 <- 'Delftstack.com'
String_Vec <- cbind(String1, String2, String3, String4, String5) # combined vector.
Result1 <- paste(String1, String2, String3, String4, String5, sep ="")
print(Result1)
Result2 <- paste(String_Vec, collapse = ":")
print(Result2)
The code above first creates a vector of string, then passes it to the paste()
function with a collapse
separator.
Output:
[1] "HelloWorld!ThisisDelftstack.com"
[1] "Hello:World!:This:is:Delftstack.com"
Concatenate Strings Using the cat()
Method in R
cat()
works similarly to the paste()
method. We pass strings and separator as parameters.
Example:
String1 <- 'Hello'
String2 <- 'World!'
String3 <- 'This'
String4 <- 'is'
String5 <- 'Delftstack.com'
Result1 <- cat(String1, String2, String3, String4, String5, sep =" ")
The code above concatenates strings similarly.
Output:
Hello World! This is Delftstack.com
Use cat()
to Save the Concatenation Result in a File
cat()
can also save the concatenation result in a file.
Example:
String1 <- 'Hello'
String2 <- 'World!'
String3 <- 'This'
String4 <- 'is'
String5 <- 'Delftstack.com'
cat(String1, String2, String3, String4, String5, sep = '\n', file = 'temp.csv')
readLines('temp.csv')
The code above will save the results in a CSV file.
Output:
[1] "Hello" "World!" "This" "is" "Delftstack.com"
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook