How to Convert Strings to Lower Case in R
-
Use
tolower()
to Convert Simple Strings to Lower Case in R -
Use
tolower()
to Convert a Data Frame Column to Lower Case in R -
Use
casefold()
to Convert Strings to Lower Case in R
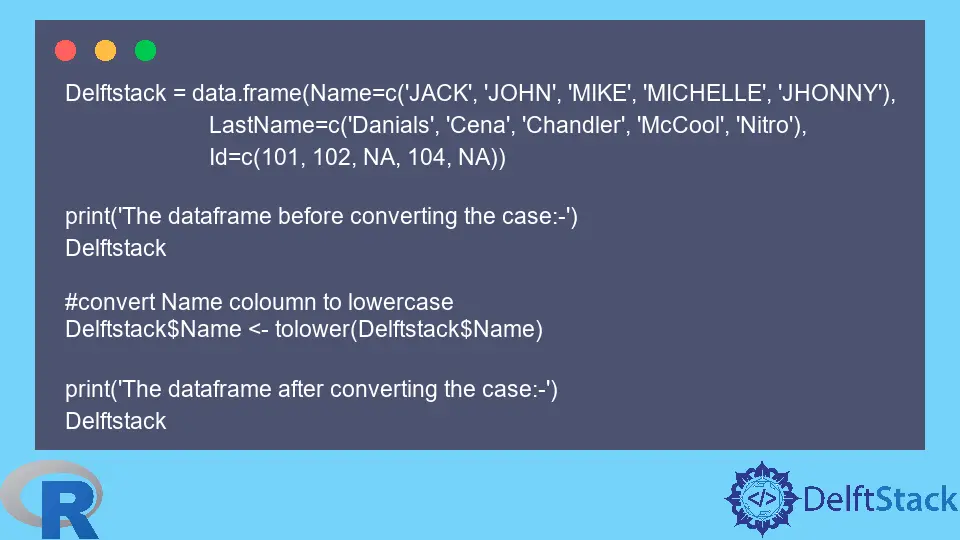
The tolower()
method and casefold()
method can convert the case of strings to lower. This tutorial demonstrates how to use these methods to convert the strings to lower case.
Use tolower()
to Convert Simple Strings to Lower Case in R
The tolower()
function takes the string as a parameter and converts it to the lower case. See example:
Demo_String <- "HELLO! THIS IS DELFTSTACK.COM"
result <- tolower(Demo_String)
print(result)
The code will convert the string to lower case.
[1] "hello! this is delftstack.com"
Use tolower()
to Convert a Data Frame Column to Lower Case in R
The tolower()
method can also convert a data frame column to a lower case. We pass the data frame with the column name as a parameter to the tolower()
method.
See example:
Delftstack = data.frame(
Name = c("JACK", "JOHN", "MIKE", "MICHELLE", "JHONNY"),
LastName = c("Danials", "Cena", "Chandler", "McCool", "Nitro"),
Id = c(101, 102, NA, 104, NA)
)
print("The dataframe before converting the case:-")
Delftstack
# convert Name coloumn to lowercase
Delftstack$Name <- tolower(Delftstack$Name)
print("The dataframe after converting the case:-")
Delftstack
The code above will convert the Name
column to lower case. See output:
[1] "The dataframe before converting the case:-"
Name LastName Id
1 JACK Danials 101
2 JOHN Cena 102
3 MIKE Chandler NA
4 MICHELLE McCool 104
5 JHONNY Nitro NA
[1] "The dataframe after converting the case:-"
Name LastName Id
1 jack Danials 101
2 john Cena 102
3 mike Chandler NA
4 michelle McCool 104
5 jhonny Nitro NA
Use casefold()
to Convert Strings to Lower Case in R
The casefold()
method can convert a string to lower and upper cases. It takes two parameters: the string and the other is Upper=F
or Upper=T
.
The Upper=F
means the lower case, and Upper=T
means the upper case. See example:
Demo_String <- "HELLO! THIS IS DELFTSTACK.COM"
result <- casefold(Demo_String, upper = F)
print(result)
The casefold()
with Upper=F
will convert the string to lower case. See output:
[1] "hello! this is delftstack.com"
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook