R にスペースなしで文字列を貼り付ける
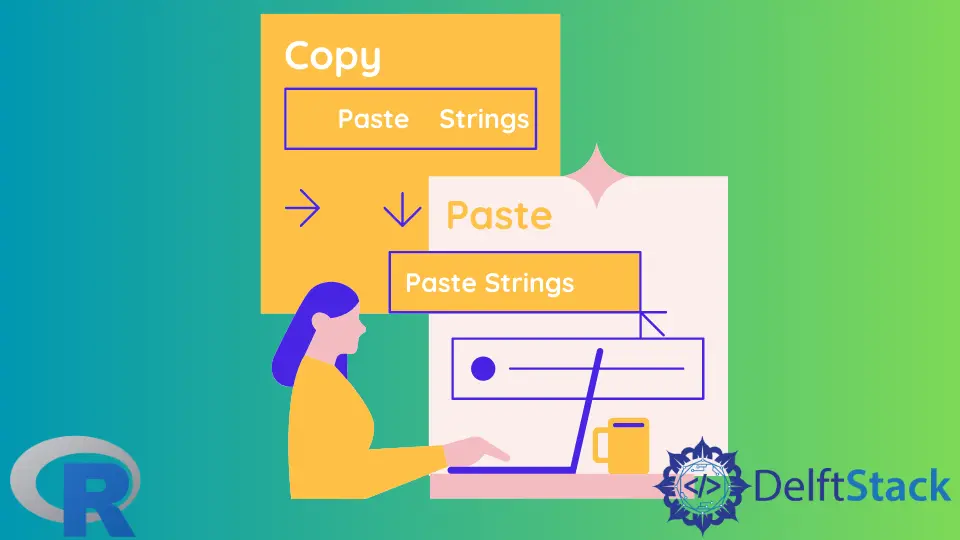
この記事では、文字列をマージ (連結) するときにスペースを削除する 3つの方法について説明します。
R で文字列をマージするときにスペースを削除する
デモンストレーションには、個々の文字列、文字列のベクトル、および文字列を含むデータ フレームを使用します。
コード例:
# The individual strings.
s1 = "The sky"
s2 = "is blue."
s1a = " The sky "
s2a = " is blue. "
# Columns for the data frame.
C1 = c("The sky", " The earth ")
C2 = c("is blue.", " is green. ")
# The data frame.
dtfr = data.frame(Col1=C1, Col2=C2)
ベース R 関数
次のベース R 関数を使用して、文字列からスペースを削除できます。
paste()
関数と sep=""
を使用してスペースを削除する
文字列を貼り付けるとき、R は間にスペースを追加します。 sep=""
を使用して、追加されたスペースを削除できます。
同じ長さのベクトルのコンテキストでは、paste()
はベクトルの対応する要素をマージします。 すべての出力要素をスペースを追加せずに 1つの長い文字列にマージするには、collapse=""
を使用できます。
スペースを追加せずにデータ フレームの列を結合するには、sep=""
を使用します。
コード例:
# MERGE WITH paste()
# Default: adds space.
paste(s1, s2)
# Remove added space.
paste(s1, s2, sep="")
# Does not remove existing spaces.
# Variables s1a and s2a have space characters at the beginning and end.
paste(s1a, s2a, sep="")
# Element-wise merging of vectors.
# No spaces are added in between.
paste(C1, C2, sep="")
# After element-wise merging, we can
# collapse all the elements of the result into a single string.
paste(C1, C2, sep="", collapse="")
# Merge columns of a data frame.
# No spaces are added in between.
dtfr$Mr = paste(dtfr$Col1, dtfr$Col2, sep="")
dtfr
出力:
> # Default: adds space.
> paste(s1, s2)
[1] "The sky is blue."
> # Remove added space.
> paste(s1, s2, sep="")
[1] "The skyis blue."
> # Does not remove existing spaces.
> # Variables s1a and s2a have space characters at the beginning and end.
> paste(s1a, s2a, sep="")
[1] " The sky is blue. "
> # Element-wise merging of vectors.
> # No spaces are added in between.
> paste(C1, C2, sep="")
[1] "The skyis blue." " The earth is green. "
> # After element-wise merging, we can
> # collapse all the elements of the result into a single string.
> paste(C1, C2, sep="", collapse="")
[1] "The skyis blue. The earth is green. "
> # Merge columns of a data frame.
> # No spaces are added in between.
> dtfr$Mr = paste(dtfr$Col1, dtfr$Col2, sep="")
> dtfr
Col1 Col2 Mr
1 The sky is blue. The skyis blue.
2 The earth is green. The earth is green.
trimws()
および paste()
関数を使用してスペースを削除する
trimws()
関数は、文字列の左、右、または両側から 空白
を削除できます。 空白文字の他に、空白
と見なされるその他の文字には、水平タブ、キャリッジ リターン、および改行文字が含まれます。
デフォルトでは、この関数は文字列の両側からすべての空白を削除します。
構文:
trimws(string, which="side", whitespace="[ \t\r\n]")
# where `side` can be `left` or `right` or `both`
コード例:
# trimws() removes spaces from the left, right or both
trimws(s2a, which="left", whitespace=" ")
trimws(s2a, which="right", whitespace=" ")
trimws(s2a, which="both", whitespace=" ")
# By default, it removes all whitespace from both sides.
s2a
trimws(s2a)
# Remove leading, trailing and added spaces when merging vectors.
paste(trimws(C1), trimws(C2), sep="")
# Remove leading, trailing and added spaces when combining columns of a data frame.
dtfr$Mr2 = paste(trimws(dtfr$Col1), trimws(dtfr$Col2), sep="")
dtfr
出力:
> # trimws() removes spaces from the left, right or both
> trimws(s2a, which="left", whitespace=" ")
[1] "is blue. "
> trimws(s2a, which="right", whitespace=" ")
[1] " is blue."
> trimws(s2a, which="both", whitespace=" ")
[1] "is blue."
> # By default, it removes all whitespace from both sides.
> s2a
[1] " is blue. "
> trimws(s2a)
[1] "is blue."
> # Remove leading, trailing and added spaces when merging vectors.
> paste(trimws(C1), trimws(C2), sep="")
[1] "The skyis blue." "The earthis green."
> # Remove leading, trailing and added spaces when combining columns of a data frame.
> dtfr$Mr2 = paste(trimws(dtfr$Col1), trimws(dtfr$Col2), sep="")
> dtfr
Col1 Col2 Mr Mr2
1 The sky is blue. The skyis blue. The skyis blue.
2 The earth is green. The earth is green. The earthis green.
最初に trimws()
を使用して、paste()
関数を使用してそれらをマージする前に、文字列の両側からスペースを削除しました。
gsub()
および paste()
関数を使用してスペースを削除する
構文:
gsub(search_for, replace_with, 文字列)
コード例:
# For vectors: merge strings and remove all spaces from the result.
gsub(" ", "", paste(C1, C2))
# For data frame: merge string columns of a data frame and remove all spaces.
dtfr$Mr3 = gsub(" ", "", paste(dtfr$Col1, dtfr$Col2))
dtfr
出力:
> # For vectors: merge strings and remove all spaces from the result.
> gsub(" ", "", paste(C1, C2))
[1] "Theskyisblue." "Theearthisgreen."
> # For data frame: merge string columns of a data frame and remove all spaces.
> dtfr$Mr3 = gsub(" ", "", paste(dtfr$Col1, dtfr$Col2))
> dtfr
Col1 Col2 Mr Mr2 Mr3
1 The sky is blue. The skyis blue. The skyis blue. Theskyisblue.
2 The earth is green. The earth is green. The earthis green. Theearthisgreen.
最初に paste()
を使用して文字列をマージし、次に gsub()
を使用してすべてのスペースを削除します。
stringr
パッケージと paste()
関数を使用してスペースを削除する
構文:
str_replace_all(string, search_for, replace_with)
コード例:
# Install the stringr package.
# install.packages("stringr")
# Load the stringr package.
library(stringr)
# With vectors.
str_replace_all(paste(C1, C2), " ", "")
# With columns of a data frame.
# We will first recreate the dataframe to improve readability.
dtfr = data.frame(Col1=C1, Col2=C2)
# Use the str_replace_all() function.
dtfr$StrRplAll = str_replace_all(paste(dtfr$Col1, dtfr$Col2), " ", "")
dtfr
出力:
> # Load the stringr package.
> library(stringr)
> # With vectors.
> str_replace_all(paste(C1, C2), " ", "")
[1] "Theskyisblue." "Theearthisgreen."
> # With columns of a data frame.
> # We will first recreate the dataframe to improve readability.
> dtfr = data.frame(Col1=C1, Col2=C2)
> # Use the str_replace_all() function.
> dtfr$StrRplAll = str_replace_all(paste(dtfr$Col1, dtfr$Col2), " ", "")
> dtfr
Col1 Col2 StrRplAll
1 The sky is blue. Theskyisblue.
2 The earth is green. Theearthisgreen.
R の tidyverse
の stringr
パッケージは stringi
パッケージの上に構築されています。 このパッケージは、gsub()
関数に似た str_replace_all()
関数を提供します。
まとめ
この記事では、次のものを削除する方法を示しました。
paste()
関数で文字列をマージすると、スペースが追加されます。- 文字列の先頭と末尾のスペース。
- 文字列のすべてのスペース。
目的の出力を取得する必要性に応じて、関数を組み合わせたりネストしたりできます。