How to Write String to a File in Python
- Write a String to a File With File Handling in Python
-
Write a String to a File With the
print()
Function in Python
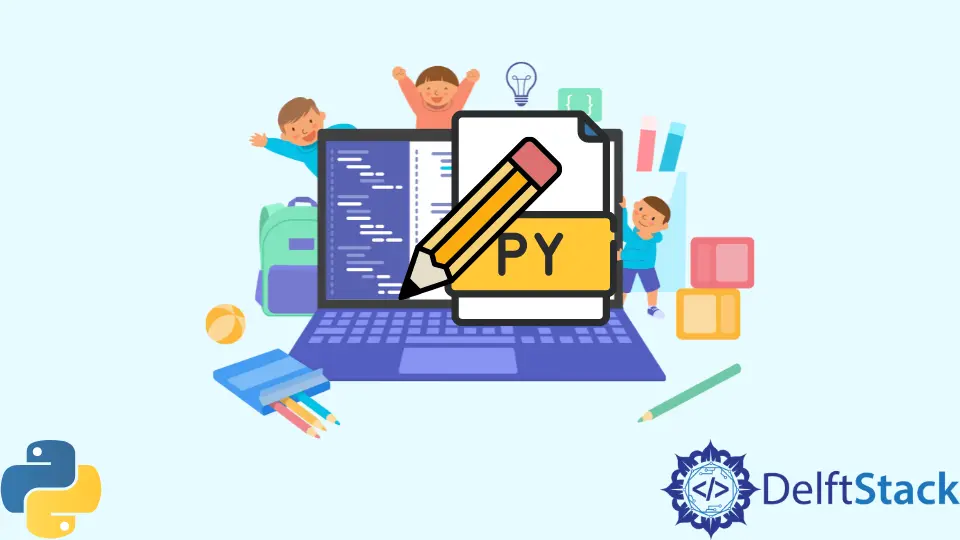
In this tutorial, we will discuss methods to write a string to a file in Python.
Write a String to a File With File Handling in Python
Python provides methods for opening, closing a file, reading from, and writing to a file. We can use file handling to write a string variable to a text file in Python. But, if we only use file handling to write a string variable to a file, we have to close the file using the close()
function each time we open the file using the open()
function. If we use a context manager, we do not have to worry about closing the file with the close()
function. The context managers provide us an efficient way to allocate and deallocate resources whenever we need them. The following code example shows us how to write a string variable to a file with file handling and context managers in Python.
var = "Some text to write in the file."
with open("Output.txt", "w") as text_file:
text_file.write("String Variable: %s" % var)
Output.txt
file:
String Variable: Some text to write in the file.
In the above code, we first initialize the string variable var
with the data that we want to write to the Output.txt
file, which lies in the same directory as our code file. We open the file using the open()
function and a context manager and write the string variable var
to the Output.txt
file with the file.write()
function in Python.
Write a String to a File With the print()
Function in Python
We can also write a string variable to a text file with the conventional print()
function in Python. The print()
function is typically used to display output to the console in Python. But there is a file parameter in the print()
function that can be used to write data inside the print function to a specific file. The following code example shows us how we can use the print()
function to write a string variable to a file in Python.
var = "Some text to be written to the file."
with open("Output.txt", "w") as txtfile:
print("String Variable: {}".format(var), file=txtfile)
Output.txt
file:
String Variable: Some text to be written to the file.
In the above code, we first initialized the string variable var
that we want to write to the Output.txt
file, which is in the same directory as our code file. We open the file using the open()
function and a context manager. Then we write the string variable var
to the Output.txt
file with the print()
function by specifying the file
parameter as txtfile
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn