How to Write Bytes to a File in Python
-
Method 1: Using the
open()
Function in Binary Mode - Method 2: Writing Multiple Bytes at Once
- Method 3: Writing Bytes from a Bytearray
- Method 4: Writing Bytes with Buffering
- Conclusion
- FAQ
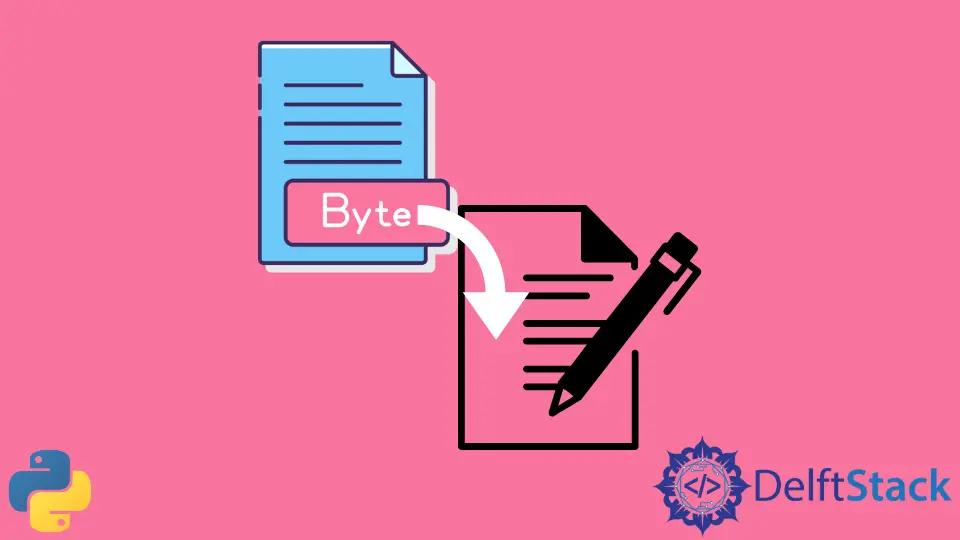
Writing bytes to a file in Python is a fundamental skill that can be incredibly useful for various applications. Whether you’re handling binary data, creating images, or saving audio files, understanding how to work with bytes can enhance your programming toolkit.
In this tutorial, we will explore the different methods to write bytes to a binary file in Python. We’ll cover the basics, from opening a file to writing data, and provide clear code examples for each method. By the end of this article, you’ll be equipped with the knowledge to effectively manage byte data in your Python projects.
Method 1: Using the open()
Function in Binary Mode
One of the simplest ways to write bytes to a file in Python is by using the built-in open()
function in binary mode. This method allows you to create or overwrite a file and write byte data directly to it. To achieve this, you’ll need to specify the file mode as 'wb'
, which stands for “write binary.”
Here’s a straightforward example:
data = b'This is some binary data.'
with open('example.bin', 'wb') as file:
file.write(data)
In this example, we define a byte string data
containing the text “This is some binary data.” Using the with
statement ensures that the file is properly closed after writing. The open()
function creates a new file named example.bin
(or overwrites it if it already exists), and the write()
method writes the byte data to the file. This method is efficient and straightforward, making it ideal for beginners.
Method 2: Writing Multiple Bytes at Once
Sometimes, you may want to write multiple byte sequences to a file at once. Instead of writing each byte individually, you can use the write()
method in a loop or create a byte array. This is particularly useful when dealing with large datasets or binary files that require multiple entries.
Here’s how to do it:
data = [b'First line of binary data.\n', b'Second line of binary data.\n', b'Third line of binary data.\n']
with open('multiple_example.bin', 'wb') as file:
for line in data:
file.write(line)
In this example, we create a list of byte strings. Each byte string represents a line of data. Using a for
loop, we iterate through the list and write each byte sequence to the file. This method is efficient for writing multiple lines or chunks of data, making it a great choice for applications like logging or data storage.
Method 3: Writing Bytes from a Bytearray
Another effective method for writing bytes to a file in Python is by using a bytearray
. A bytearray
is a mutable sequence of bytes, which means you can modify it after its creation. This is particularly useful when you need to build up a byte sequence dynamically before writing it to a file.
Here’s an example of how to use a bytearray
:
data = bytearray()
data.extend(b'Line 1: This is the first line.\n')
data.extend(b'Line 2: This is the second line.\n')
data.extend(b'Line 3: This is the third line.\n')
with open('bytearray_example.bin', 'wb') as file:
file.write(data)
In this code snippet, we create an empty bytearray
named data
. We then use the extend()
method to add multiple byte strings to this bytearray
. Finally, we open a file in binary write mode and write the entire bytearray
at once. This method is particularly useful when you need the flexibility to modify the byte data before writing it to a file.
Method 4: Writing Bytes with Buffering
For larger files or when performance is critical, you might want to consider buffering. By default, Python uses a buffer when writing files, but you can adjust the buffer size for optimal performance. This is especially useful when writing large amounts of binary data, as it can significantly speed up the writing process.
Here’s an example:
data = b'This is a large amount of binary data.' * 1000
with open('buffered_example.bin', 'wb', buffering=8192) as file:
file.write(data)
Output:
No output, but a file named buffered_example.bin is created with a large amount of binary data.
In this example, we create a large byte string by repeating a smaller byte string multiple times. We then open a file in binary write mode with a specified buffer size of 8192 bytes. This means that the data will be written in chunks of 8192 bytes, which can improve performance when dealing with large files. Adjusting the buffer size can be a useful technique for optimizing file operations in Python.
Conclusion
Writing bytes to a file in Python is a straightforward process that opens up a world of possibilities for handling binary data. Whether you’re creating simple binary files, logging data, or working with complex datasets, the methods discussed in this tutorial provide a solid foundation for your programming needs. Remember to experiment with different techniques to find the one that best suits your project. With practice, you’ll become proficient in managing byte data in Python, enhancing your coding skills and expanding your capabilities.
FAQ
-
How can I read bytes from a binary file in Python?
You can use theopen()
function with the mode'rb'
to read bytes from a binary file. -
What is the difference between
b''
andbytearray()
?
b''
creates an immutable bytes object, whilebytearray()
creates a mutable sequence of bytes that can be modified. -
Can I write text to a binary file?
Yes, but you need to encode the text into bytes first, for example, usingbytes(text, 'utf-8')
. -
What happens if I try to write non-byte data to a binary file?
Python will raise aTypeError
if you attempt to write non-byte data to a binary file. -
Is it possible to append bytes to an existing binary file?
Yes, you can open a binary file in append mode by using'ab'
as the mode in theopen()
function.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn