用 Python 向文件写入字节
Manav Narula
2023年1月30日
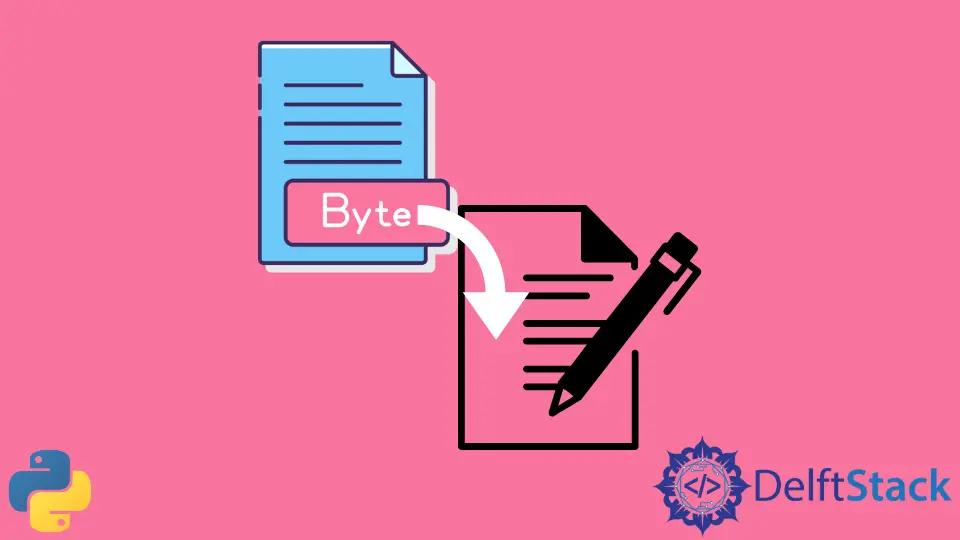
在本教程中,我们将介绍在 Python 中如何向二进制文件写入字节。
二进制文件包含类型为字节的字符串。当我们读取二进制文件时,会返回一个字节类型的对象。在 Python 中,字节用十六进制数字表示。它们的前缀是 b
字符,表示它们是字节。
在 Python 中向文件写入字节
为了向文件写入字节,我们将首先使用 open()
函数创建一个文件对象,并提供文件的路径。文件应该以 wb
模式打开,它指定了二进制文件的写入模式。下面的代码显示了我们如何向文件写入字节。
data = b"\xC3\xA9"
with open("test.bin", "wb") as f:
f.write(data)
当我们需要在现有文件的末尾添加更多的数据时,我们也可以使用追加模式 - a
。比如说
data = b"\xC3\xA9"
with open("test.bin", "ab") as f:
f.write(data)
要在特定位置写入字节,我们可以使用 seek()
函数,指定文件指针的位置来读写数据。例如:
data = b"\xC3\xA9"
with open("test.bin", "wb") as f:
f.seek(2)
f.write(data)
在 Python 中向文件写入字节数组
我们可以使用 bytearray()
函数创建一个字节数组。它返回一个可变的 bytearray
对象。我们也可以将其转换为字节来使其不可变。在下面的例子中,我们将一个字节数组写入一个文件。
arr = bytearray([1, 2, 5])
b_arr = bytes(arr)
with open("test.bin", "wb") as f:
f.write(b_arr)
将 BytesIO
对象写入二进制文件中
io
模块允许我们扩展与文件处理有关的输入输出函数和类。它用于在内存缓冲区中分块存储字节和数据,并允许我们处理 Unicode 数据。这里使用 BytesIO
类的 getbuffer()
方法来获取对象的读写视图。请看下面的代码。
from io import BytesIO
bytesio_o = BytesIO(b"\xC3\xA9")
with open("test.bin", "wb") as f:
f.write(bytesio_o.getbuffer())
作者: Manav Narula
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn