How to Use the try...else Block in Python
-
Use the
try...except
Block Without anelse
Clause in Python -
Use the
try...except
Block With theelse
Clause in Python
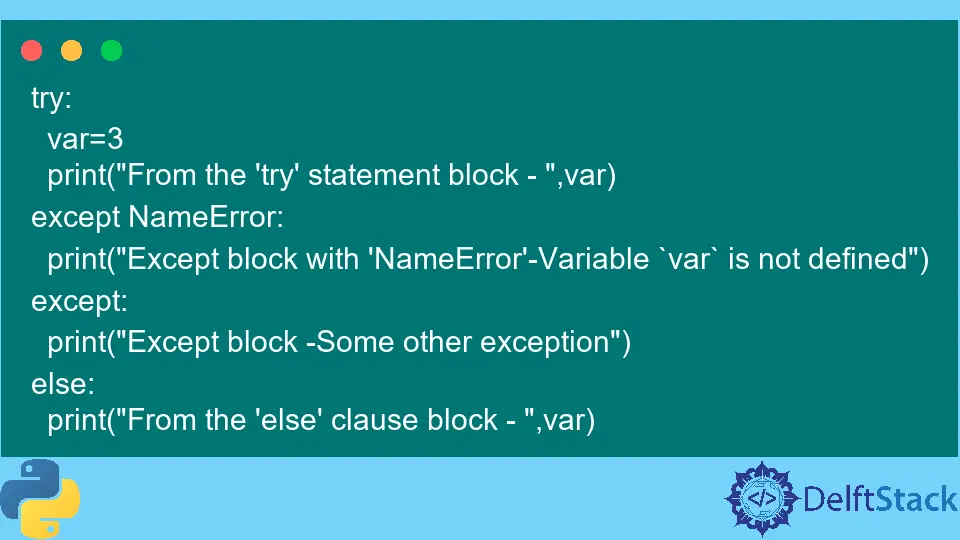
This article explains how to use the else
along with the try...except
pair. We’ve included some methods you use as your guide to incorporate the pair in a program.
try:
<a code block >
except:
<a code block >
else:
<a code block. Note: 'else' clause is optional>
The except
clause is used to specify >=1
exception handlers. The code block associated with this clause is executed if there are exceptions thrown in the try
block and hence, handles the error.
The else
clause is optional. It’s placed after all the except
clauses. The code block associated with this clause is executed only if there are no exceptions thrown in the try
block.
Let us try examples with and without the else
statements.
Use the try...except
Block Without an else
Clause in Python
Here’s an example code demonstrating the use of try...except
without the else
clause.
try:
print("From the 'try' statement block - ", var1)
except:
print("Except block - An exception occurred")
Here, var1
is not defined in the try
block, and the following occurs.
- An exception occurs in the
try
block. - The
print
statement present in thetry
block is NOT printed. - The
except
code block is executed.
Output:
Except block - An exception occurred
Use the try...except
Block With the else
Clause in Python
Here, the example code shows how you can use the try...except
with the else
clause.
try:
var = 3
print("From the 'try' statement block - ", var)
except NameError:
print("Except block with 'NameError'-Variable `var` is not defined")
except:
print("Except block -Some other exception")
else:
print("From the 'else' clause block - ", var)
Here, var
is defined in the try
block, and so the following occurs.
- No exception occurs in the
try
block. - The
print
statement present in thetry
block is printed. - The
except
code block is NOT executed. - The
else
code block is executed. - The
print
statement present in theelse
block is printed.
Output:
From the 'try' statement block - 3
From the 'else' clause block - 3