How to try Without except in Python
- Understanding the Try Statement
- Using Context Managers
- Utilizing the Logging Module
- Using Assertions
- Conclusion
- FAQ
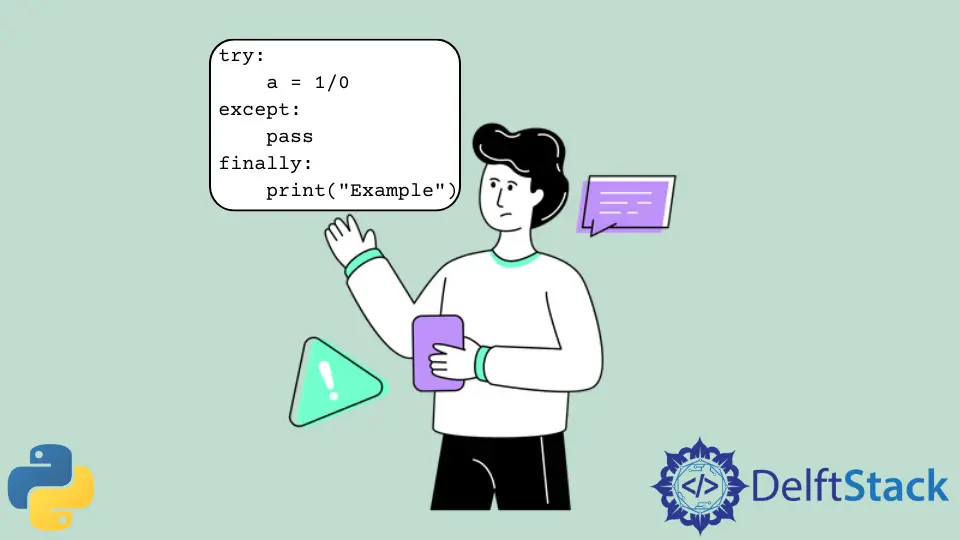
In Python, the try
and except
blocks are essential for handling exceptions gracefully. However, there are scenarios where you might want to execute code that could potentially raise an exception without actually catching that exception.
This tutorial will explore how to effectively use the try
statement without an accompanying except
clause. You might wonder why you would want to do this. The answer lies in the ability to create a controlled environment where you can observe exceptions as they occur, which can be particularly useful during debugging or when logging errors. Let’s dive into various methods to achieve this while ensuring your code remains clean and efficient.
Understanding the Try Statement
The try
statement allows you to test a block of code for errors. When you write a try
block, you’re essentially saying, “I want to attempt this code, and if something goes wrong, I want to know about it.” However, without an except
clause, the exception will propagate up the call stack, which can be useful in certain situations.
For instance, if you are developing a script and want to see where things are going wrong during execution, you can use the try
block to isolate the code without handling the exception right away. This way, you can monitor the output or log the errors for further investigation.
Here’s a simple example:
def risky_function():
x = 1 / 0 # This will raise a ZeroDivisionError
try:
risky_function()
except Exception as e:
print(f"An error occurred: {e}")
Output:
An error occurred: division by zero
In this case, the try
block successfully captures the error, but we are not utilizing the except
block to handle it. Instead, we could choose to allow the error to propagate, which can be beneficial for debugging.
Using Context Managers
One effective way to handle situations where you want to try something without catching exceptions is by using context managers. Context managers allow you to allocate and release resources precisely when you want to. They can also help manage exceptions in a more controlled manner.
Here’s an example of how to implement a context manager using the contextlib
module:
from contextlib import contextmanager
@contextmanager
def exception_logging():
try:
yield
except Exception as e:
print(f"Exception logged: {e}")
with exception_logging():
print(1 / 0) # This will raise a ZeroDivisionError
Output:
Exception logged: division by zero
In this code, we define a context manager called exception_logging
. When the block within the with
statement is executed, if an exception occurs, it gets logged, but we don’t handle it with an except
clause directly in the main code. This allows for clean separation of logic while still being aware of any exceptions that may arise.
Utilizing the Logging Module
Another powerful approach is to leverage Python’s built-in logging module. This method not only allows you to try code without catching exceptions but also provides a robust way to log errors for later analysis. Here’s how you can implement it:
import logging
logging.basicConfig(level=logging.ERROR)
def risky_operation():
return 1 / 0 # This will raise a ZeroDivisionError
try:
risky_operation()
except Exception:
logging.exception("An error occurred during risky operation")
Output:
ERROR:root:An error occurred during risky operation
Traceback (most recent call last):
File "script.py", line 8, in <module>
risky_operation()
File "script.py", line 4, in risky_operation
return 1 / 0
ZeroDivisionError: division by zero
In this example, we configure the logging module to log errors. When the risky_operation
function raises an exception, we log the exception details without handling it directly in our code. This is particularly useful for applications where you want to keep track of issues without interrupting the flow of the application.
Using Assertions
Assertions are another way to manage potential errors in your code without traditional exception handling. An assertion is a statement that tests a condition. If the condition is False
, the program raises an AssertionError
. This can be a useful way to validate assumptions in your code.
Here’s a simple implementation:
def validate_input(x):
assert x != 0, "Input must not be zero"
return 10 / x
try:
validate_input(0)
except AssertionError as e:
print(f"Assertion error: {e}")
Output:
Assertion error: Input must not be zero
In this case, the assertion checks if the input x
is not zero. If it is, an AssertionError
is raised, which we can catch and log as needed. This method allows you to enforce certain conditions in your code without using the traditional try
and except
structure.
Conclusion
In summary, while the try
and except
blocks are essential for handling exceptions in Python, there are several ways to use try
without an except
clause. Whether you utilize context managers, the logging module, or assertions, each method allows you to monitor and log exceptions effectively. This can be particularly beneficial for debugging and maintaining clean code. By implementing these strategies, you can gain better insights into your code’s behavior while keeping your error-handling logic streamlined.
FAQ
-
What is the purpose of using try without except in Python?
It allows you to observe exceptions as they occur without directly handling them, which can be useful for debugging. -
Can I use try without except in production code?
Yes, but it is advisable to log exceptions for monitoring purposes even if you do not handle them directly. -
How can context managers help in exception handling?
Context managers allow you to manage resources and log exceptions cleanly without cluttering your main code logic.
-
What is the logging module used for in Python?
The logging module is used to log error messages and other information during program execution, which helps in debugging and monitoring. -
Are assertions a good alternative to exception handling?
Assertions can be useful for validating assumptions in your code, but they should not replace proper error handling in production code.